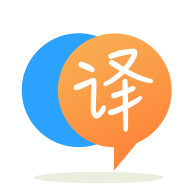
[英]How to print array of numbers given i and j, such that sum of all elements of i is equal to j?
[英]first print all numbers in given sequence, then all characters and other symbols using Array
如何首先打印给定序列中的所有数字,然后仅使用数组而不是 C++ 中的字符串打印所有字符和字母???
例如
intput: 5 (size of array) --> a 1 2 r 4
output: 1 2 4 a r
input: 10 --> a s d @ # $ 7 8 9 1 0
output: 7 8 9 1 0 a s d @ # $
#include <iostream>
int main()
{
int n, c, j = 0, m = 0, a = 0;
char arr[1000];
std::cin >> n;
for (int i = 0; i < n; ++i)
{
std::cin >> arr[i];
std::cout << arr[i] << " ";
}
}
如果您现在想坚持使用for
循环,只需使用cctype header 中的一些帮助程序来清理您的代码:
#include <cctype>
for (int i = 0; i < n; i++) {
if (std::isdigit(arr[i]))
std::cout << (char)arr[i] << ' ';
}
for (int i = 0; i < n; i++) {
if (std::isalpha(arr[i]))
std::cout << (char)arr[i] << ' ';
}
for (int i = 0; i < n; i++) {
if (!std::isdigit(arr[i]) && !std::isalpha(arr[i]))
std::cout << (char)arr[i] << ' ';
}
这段代码绝对不是最优的,正如你可以从其他人的std::partition
更优雅的解决方案中看到的那样。 一旦您对自己的技能更有信心,请考虑尝试一种算法方法:标准库有很多选择。 std::partition
在这里运行良好,但您也可以尝试使用排序或删除算法。 所有这些函数需要的是您的数据范围(在您的情况下, &arr[0]
或arr
是范围的开始,而arr + n
是结束),通常是一些 function 来指定您希望数据如何分区,排序等
您可以首先对数组进行分区以将所有数字放在开头:
auto nums = std::stable_partition(arr, arr + n,
[](unsigned char c) { return std::isdigit(c); });
然后对数组的剩余部分进行分区以获取字符:
std::stable_partition(nums, arr + n,
[](unsigned char c) { return std::isalpha(c); });
然后将所有内容打印出来:
std::copy(arr, arr + n, std::ostream_iterator<char>(std::cout, " "));
看来您的意思如下。 也就是说,使用标准算法 std::partition 可以很容易地完成分配,该算法两次应用于输入的序列。 如果您想将符号的顺序保持在可以使用算法std::stable_partition
的同一类别中。 只需在下面的程序中将std::partition
替换为std::stable_partition
。
#include <iostream>
#include <algorithm>
#include <iterator>
#include <cctype>
int main()
{
const size_t N = 1000;
char a[N];
size_t n = 0;
std::cin >> n;
if ( N < n ) n = N;
for ( size_t i = 0; i < n; i++ )
{
std::cin >> a[i];
}
auto it = std::partition( a, a + n, []( unsigned char c ){ return ::isdigit( c ); } );
std::partition( it, a + n, []( unsigned char c ){ return ::isalpha( c ); } );
for ( size_t i = 0; i < n; i++ )
{
std::cout << a[i] << ' ';
}
std::cout << '\n';
return 0;
}
如果进入序列
10
a s d @ # $ 7 8 9 1 0
那么程序 output 将是
1 9 8 7 a s d @ $ #
这是相同的程序,但使用了 std::stable_partition。
#include <iostream>
#include <algorithm>
#include <iterator>
#include <cctype>
int main()
{
const size_t N = 1000;
char a[N];
size_t n = 0;
std::cin >> n;
if ( N < n ) n = N;
for ( size_t i = 0; i < n; i++ )
{
std::cin >> a[i];
}
auto it = std::stable_partition( a, a + n, []( unsigned char c ){ return ::isdigit( c ); } );
std::stable_partition( it, a + n, []( unsigned char c ){ return ::isalpha( c ); } );
for ( size_t i = 0; i < n; i++ )
{
std::cout << a[i] << ' ';
}
std::cout << '\n';
return 0;
}
相同输入序列的程序 output 看起来像
7 8 9 1 a s d @ # $
```
#include <iostream>
int main() {
int n, c, j = 0, m = 0, a = 0;
char A[1000], B[1000], C[1000];
std::cin >> n;
for (int i=0; i<n; ++i)
{
std::cin >> A[i];
}
for (int i = 0; i < n; ++i)
{
if(A[i] >= '0' && A[i] <= '9')
{
B[j] = A[i];
j++;
c = j;
}
else
{
C[m] = A[i];
m++;
}
}
for (int i = 0; i < n; ++i)
{
A[i] = B[i];
}
for (int i = c; i < n; ++i)
{
A[i] = C[a];
a++;
}
for (int i = 0; i < n; ++i)
std::cout << A[i] << " ";
}
#include <bits/stdc++.h>
using namespace std;
#include <iostream>
int main()
{
int n;
int arr[1000];
std::cin >> n;
int t[1000];
int count=0;
for (int i = 0; i < n; ++i)
{
char temp;
cin>>temp;
if((int(temp)>=91 && int(temp)<=96) || (int(temp)>=58 && int(temp)<=64)||(int(temp)>=33 && int(temp)<=47)){
t[count]=int(temp);
count++;
}
else
{
arr[i]=int(temp);
}
}
sort(arr,arr+n);
for (int i = 0; i < n; ++i){
cout<<char(arr[i])<<" ";
}
for (int i = 0; i < n; ++i){
cout<<char(t[i])<<" ";
}
}
将输入作为“char”并将其类型转换为“int”。如果 int 值表示一个特殊字符,则将其存储在另一个数组中。然后根据 integer 值执行排序,最后在打印时再次转换为“char”。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.