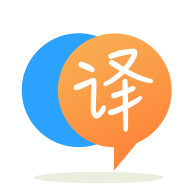
[英]Fix for Cognitive Complexity for multiple if else conditions in Java
[英]Simplify if else conditions to reduce cognitive complexity in Java
有没有办法简化这个 java function?
为了代码的可维护性,需要简化。
public void pushDocument(ESDocumentType esDocumentType, Object data, String documentId, long userId, long organizationId) {
boolean proceed = false;
if (esDocumentType.equals(ESDocumentType.XMLACTIVITY)) {
proceed = Constants.ELASTIC_LOGGING_ENABLED && Constants.ELASTIC_XMLACTIVITY_ENABLED || Constants.SQS_LOGGING_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.XMLREQRES)) {
proceed = Constants.ELASTIC_LOGGING_ENABLED && Constants.ELASTIC_XMLREQRES_ENABLED || Constants.SQS_LOGGING_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.ORDERHISTORY)) {
proceed = Constants.ELASTIC_LOGGING_ENABLED && Constants.ELASTIC_ORDERHISTORY_ENABLED || Constants.SQS_LOGGING_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.SINGIN)) {
proceed = Constants.ELASTIC_LOGGING_ENABLED && Constants.ELASTIC_SIGNIN_ENABLED || Constants.SQS_LOGGING_ENABLED;
} else if (esDocumentType.equals(ESDocumentType.GOOGLESEARCH)) {
proceed = Constants.ELASTIC_LOGGING_ENABLED && Constants.ELASTIC_GOOGLESEARCH_ENABLED || Constants.SQS_LOGGING_ENABLED;
}
if (proceed) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data, documentId, userId, organizationId);
}
}
我不知道你的确切用例,你必须自己改进一下,但这样的事情可能会奏效。
List<ESDocumentType> enabled; // fill this based on your "Constant.ELASTIC_<BLAH>_ENABLED" constants in the constructor
public void pushDocument(ESDocumentType type, other parameters) {
boolean proceed = (Constants.ELASTIC_LOGGING_ENABLED && enabled.contains(type)) || Constants.SQS_LOGGING_ENABLED;
if (proceed) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data, documentId, userId, organizationId);
}
}
使用 switch 语句,我认为它应该像这样工作(未经测试):
switch(ESDocumentType)
{
case ESDocumentType.XMLACTIVITY:
proceed = Constants.ELASTIC_LOGGING_ENABLED &&
Constants.ELASTIC_XMLACTIVITY_ENABLED || Constants.SQS_LOGGING_ENABLED;
break;
[ .... add the other cases here]
default:
//we do not need to set proceed to false manually, but here would be the case for that
break;
}
这是一种可能性。 如果我这样做,我会设置一个 map 来获取适当的 boolean。
public void pushDocument(ESDocumentType esDocumentType,
Object data, String documentId, long userId,
long organizationId) {
boolean proceed = esDocumentType.equals(ESDocumentType.XMLACTIVITY);
proceed = proceed || esDocumentType.equals(ESDocumentType.XMLREQRES);
proceed = proceed || esDocumentType.equals(ESDocumentType.ORDERHISTORY);
proceed = proceed || esDocumentType.equals(ESDocumentType.SINGIN);
proceed = proceed || esDocumentType.equals(ESDocumentType.GOOGLESEARCH);
// if any logging is to be done, proceed and one of the others must be true.
if (proceed && (Constants.SQS_LOGGING_ENABLED
|| Constants.ELASTIC_LOGGING_ENABLE)) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data,
documentId, userId, organizationId);
}
}
这是我提到的替代方案。 唯一的区别是如何确定proceed
。
Map<ESDocumentType, Boolean> docType = Map.of(
ESDocumentType.EMLACTIVITY, Constants.ELASTIC_XMLACTIVITY_ENABLED,
ESDocumentType.XMLREQRES, Constants.ELASTIC_XMLREQRES_ENABLED,
ESDocumentType.ORDERHISTORY, Constants.ELASTIC_ORDERHISTORY_ENABLED,
ESDocumentType.SINGIN, Constants.ELASTIC_SINGIN_ENABLED,
ESDocumentType.GOOGLESEARCH, Constants.ELASTIC_GOOGLESEARCH_ENABLED);
public void pushDocument(ESDocumentType esDocumentType,
Object data, String documentId, long userId,
long organizationId) {
boolean proceed = docType.getOrDefault(esDocumentType, false);
// if any logging is to be done, proceed and one of the others must be true.
if (proceed && (Constants.SQS_LOGGING_ENABLED
|| Constants.ELASTIC_LOGGING_ENABLE)) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data,
documentId, userId, organizationId);
}
}
public void pushDocument(ESDocumentType esDocumentType, Object data, String documentId, long userId, long organizationId) {
boolean proceed = Constants.ELASTIC_LOGGING_ENABLED;
if (esDocumentType.equals(ESDocumentType.XMLACTIVITY)) {
proceed &&= Constants.ELASTIC_XMLACTIVITY_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.XMLREQRES)) {
proceed &&= Constants.ELASTIC_XMLREQRES_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.ORDERHISTORY)) {
proceed &&= Constants.ELASTIC_ORDERHISTORY_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.SINGIN)) {
proceed &&= Constants.ELASTIC_SIGNIN_ENABLED;
}
else if (esDocumentType.equals(ESDocumentType.GOOGLESEARCH)) {
proceed &&= Constants.ELASTIC_GOOGLESEARCH_ENABLED;
}
if (proceed || Constants.SQS_LOGGING_ENABLED) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data, documentId, userId, organizationId);
}
}
对于 Java8 使用下面的代码,这将有助于避免认知和圈复杂度
public void pushDocument(ESDocumentType esDocumentType, Object data, String documentId, long userId, long organizationId) {
EnumMap<ESDocumentType, Boolean> docType = new EnumMap<>(ESDocumentType.class);
docType.put(ESDocumentType.XMLACTIVITY, Constants.ELASTIC_XMLACTIVITY_ENABLED);
docType.put(ESDocumentType.XMLREQRES, Constants.ELASTIC_XMLREQRES_ENABLED);
docType.put(ESDocumentType.ORDERHISTORY, Constants.ELASTIC_ORDERHISTORY_ENABLED);
docType.put(ESDocumentType.SINGIN, Constants.ELASTIC_SIGNIN_ENABLED);
docType.put(ESDocumentType.GOOGLESEARCH, Constants.ELASTIC_GOOGLESEARCH_ENABLED);
boolean proceed = docType.getOrDefault(esDocumentType, false);
if (proceed && Constants.ELASTIC_LOGGING_ENABLED || Constants.SQS_LOGGING_ENABLED) {
LogThread logThread = new LogThread();
logThread.pushDocument(esDocumentType, data, documentId, userId, organizationId);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.