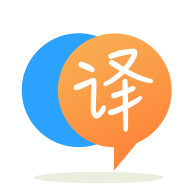
[英]spring boot is not creating table from entity using postgres container
[英]Prevent @Entity from re creating a database table - Spring Boot
I'm kinda new to spring boot data jpa, as far as I know @Entity is used to represent a database table in the application, for this project im using spring-boot 2.2.5.RELEASE
and H2
as in memory database.
到目前为止,我已经得到了这个。
在资源/数据内部。sql
CREATE TABLE CURRENCY (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(250) NOT NULL,
code VARCHAR(250) NOT NULL
);
CREATE TABLE EXCHANGE_CURRENCY (
id INT AUTO_INCREMENT PRIMARY KEY,
IdFx1 INT NOT NULL,
IdFx2 INT NOT NULL,
equivalent DECIMAL NOT NULL,
FOREIGN KEY (IdFx1) REFERENCES CURRENCY(id),
FOREIGN KEY (IdFx2) REFERENCES CURRENCY(id)
);
我的实体 class
import javax.persistence.*;
@Entity
@Table(name = "CURRENCY")
public class Currency {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String code;
}
存储库
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.query.Param;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface CurrencyRepository extends CrudRepository<Currency, Long> {
@Query("SELECT c FROM CURRENCY WHERE c.code LIKE %:code%")
List<Currency> findCurrencyByCode(@Param("code") String code);
}
和服务
import com.currency.canonical.models.Currency;
import com.currency.canonical.request.ExchangeValueRequest;
import com.currency.canonical.response.ExchangeValueResponse;
import com.currency.dao.CurrencyService;
import com.currency.dao.repository.CurrencyRepository;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class CurrencyConversionServiceImpl implements CurrencyConversionService {
Logger logger = LoggerFactory.getLogger(CurrencyConversionServiceImpl.class);
@Autowired
private CurrencyRepository currencyRepository;
@Override
public ExchangeValueResponse performCurrencyConversion(ExchangeValueRequest request) {
final long initialTime = System.currentTimeMillis();
ExchangeValueResponse objExchangeValueResponse = new ExchangeValueResponse();
try {
List<Currency> currencyList = currencyRepository.findCurrencyByCode(request.getMonedaOrigen());
currencyList.forEach(System.out::println);
} catch (Exception e) {
}
return objExchangeValueResponse;
}
}
执行应用程序时出现此错误
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'entityManagerFactory' defined in class path resource [org/springframework/boot/autoconfigure/orm/jpa/HibernateJpaConfiguration.class]: Initialization of bean failed; nested exception is org.springframework.jdbc.datasource.init.ScriptStatementFailedException: Failed to execute SQL script statement #2 of URL [file:/C:/Users/Usuario/Documents/IdeaProjects/currency-converter/currency-converter-resource/target/classes/data.sql]: CREATE TABLE CURRENCY ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(250) NOT NULL, code VARCHAR(250) NOT NULL ); nested exception is org.h2.jdbc.JdbcSQLSyntaxErrorException: Tabla "CURRENCY" ya existe
Table "CURRENCY" already exists; SQL statement:
CREATE TABLE CURRENCY ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(250) NOT NULL, code VARCHAR(250) NOT NULL ) [42101-200]
为什么@Entity 试图重新创建一个应该只代表的表,有没有办法禁用它?
这里的问题是文件data.sql的名称,正如 spring 所建议的,有两个重要的文件可以帮助您控制数据库的创建,它们是
schema.sql
- 这个文件顾名思义,是创建数据库模式所需的 DDL 语句。data.sql
- 此文件将包含正确填充初始数据库所需的所有 DML 语句。 您的应用程序的问题是在data.sql
文件中指定了 DDL,这使 spring 感到困惑,并且它尝试执行 DDL,因为它认为它是 DML。
您问题的解决方案只需将data.sql
重命名为schema.sql
和 spring 将处理 Z65E8800B5C68006AFB8B826。
我还发现存储库的另一个问题,因为您使用自定义查询@Query("SELECT code FROM CURRENCY WHERE code LIKE %:code%")
可能会在启动存储库时导致错误,因为 java 实体名称是大小写-敏感的。 您可以通过以下方式解决此问题 -
A. 由于它的类似查询 spring 存储库已经支持它,您可以重写方法,如 -
List<Currency> findByCodeLike(@Param("code") String code);
B. 使用 JPQL 查询,与您在代码中所做的相同,只是更改表名,因为 JPA 实体名称区分大小写
@Query("SELECT code FROM Currency WHERE code LIKE %:code%")
List<Currency> findCurrencyByCode(@Param("code") String code);
C。 如果您仍想使用数据库模式“CURRENCY”中的表名保留当前查询,那么您可以在@Query
中使用nativeQuery
标志让 spring 知道您使用的是本机查询而不是 JPQL -
@Query(value = "SELECT code FROM CURRENCY WHERE code LIKE %:code%", nativeQuery = true)
List<Currency> findCurrencyByCode(@Param("code") String code);
希望这可以帮助!
发生错误是因为当您运行应用程序时,它会尝试重新创建表,解决方法如下:
drop table if exists [tablename]
表。CREATE TABLE
更改为CREATE TABLE IF NOT EXISTS
JPA 具有 DDL 生成功能,这些功能可以设置为在启动时针对数据库运行。 这是通过两个外部属性控制的:
spring.jpa.generate-ddl
(布尔值)打开和关闭该功能,并且与供应商无关。spring.jpa.hibernate.ddl-auto
(enum) is a Hibernate feature that controls the behavior in a more fine-grained way. spring.jpa.hibernate.ddl-auto
属性如何工作? 您可以根据环境参考以下配置。
开发——创建-删除、更新
生产 - 无
spring.jpa.hibernate.ddl-auto 属性在 Z38008DD81C2F4AFDFE798 中如何工作?
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.