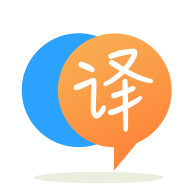
[英]How to reduce an array of objects by key and sum its values in javascript
[英]Use reduce to sum values in array of objects
我有这个对象数组:
const data = [
{val: 40, color: 'red'},
{val: 5, color: 'green'},
{val: 55, color: 'lime'}
]
这是我想要获得的:
const result = [
{val: 40, color: 'red'},
{val: 45, color: 'green'},
{val: 100, color: 'lime'}
]
所以每个项目应该有相同的颜色和以前数据的累积值。
这是我尝试的:
const data = [ {val: 40, color: 'red'}, {val: 5, color: 'green'}, {val: 55, color: 'lime'} ] // const result = [ // {val: 40, color: 'red'}, // {val: 45, color: 'green'}, // {val: 100, color: 'lime'} // ] const result = data.reduce((r, value, i) => { const { val, color } = value const cumVal = i === 0? val: r[i - 1].val const newDatum = { val: cumVal, color } return newDatum }, data[0]) console.log(result)
错误在哪里? 为什么r[i - 1]
未定义?
您从单个元素开始 reduce,该元素不是数组。
相反,您可以关闭sum
和 map 新对象。
const data = [{ val: 40, color: 'red' }, { val: 5, color: 'green' }, { val: 55, color: 'lime' }], result = data.map( (sum => ({ val, color }) => ({ val: sum += val, color })) (0) ); console.log(result);
您的代码中有四个问题:
const cumVal = i === 0? val: r[i - 1].val
const cumVal = i === 0? val: r[i - 1].val
你应该指定0
作为默认值,而不是val
const newDatum = { val: cumVal, color }
您需要将val
添加到cumVal
data
数组的第一个元素,因为您希望得到一个数组,而不是 objectr
,而不是newDatum
- 再次,您希望最后有一个数组,而不是 object这是一个固定版本:
const data = [ {val: 40, color: 'red'}, {val: 5, color: 'green'}, {val: 55, color: 'lime'} ] // const result = [ // {val: 40, color: 'red'}, // {val: 45, color: 'green'}, // {val: 100, color: 'lime'} // ] const result = data.reduce((r, value, i) => { const { val, color } = value const cumVal = i === 0? 0: r[i - 1].val const newDatum = { val: val + cumVal, color } r.push(newDatum); return r; }, []) console.log(result)
const result2 = data.reduce((acc, { val, color }, i) => {
val += (i>0) ? acc[i - 1].val: 0;
return acc.concat([{ val, color }])
}, [])
您的问题的解决方案。
问题:
虽然我认为 Map 在这里会是一个更好的选择。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.