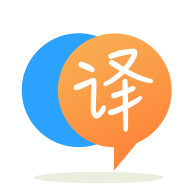
[英]C++ : 2-D Pointer Array Sorting: Selection Sort doesn't work for certain instance
[英]C++ Selection Sort not Sorting Some Values of an Array
我正在尝试使用选择排序算法对整数数组进行排序,但有些数字没有排序。 下面是我的代码的隔离部分,它进行排序和 output。 有什么建议么?
#include <iostream>
using namespace std;
int main()
{
int smallestIndex;
int temp;
int X[13] = {1, 19, 2, 18, 4, 12, 7, 8, 10, 3, 11, 17, 9};
for (int Index = 0; Index < 12; Index++) {
smallestIndex = Index;
for (int minIndex = Index+1; minIndex < 13; minIndex++) {
if (X[minIndex] < X[smallestIndex]) {
smallestIndex=minIndex;
}
if (smallestIndex != Index) {
temp = X[Index];
X[Index] = X[smallestIndex];
X[smallestIndex] = temp;
}
}
}
for (int i = 0; i < 13; i++) {
cout << X[i] << endl;
}
}
output:
1
2
4
7
9
3
10
8
11
12
17
18
19
通过使用swap()
function 并使用另外两个称为void selectionSort()
和void printArray()
的函数来使代码看起来更清晰,有一种更简单的方法可以做到这一点。
#include <iostream>
#include <algorithm>
void swap(int *xp, int *yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
void selectionSort(int arr[], int n)
{
int i, j, min_idx;
// One by one move boundary of unsorted subarray
for (i = 0; i < n-1; i++)
{
// Find the minimum element in unsorted array
min_idx = i;
for (j = i+1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
swap(&arr[min_idx], &arr[i]);
}
}
void printArray(int arr[], int size)
{
int i;
for (i=0; i < size; i++)
std::cout << arr[i] << " ";
std::cout << std::endl;
}
int main()
{
int arr[] = {1, 19, 2, 18, 4, 12, 7, 8, 10, 3, 11, 17, 9};
int n = sizeof(arr)/sizeof(arr[0]);
selectionSort(arr, n);
std::cout << "The sorted array is: \n";
printArray(arr, n);
return 0;
}
Output:
The sorted array is:
1 2 3 4 7 8 9 10 11 12 17 18 19
您可以做到这一点的另一种方法是严格使用std::swap
而不使用pointers
。 确保包含std::swap
的#include<algorithm>
header 文件。 您还需要为std::size
包含#include <iterator>
。
#include <iostream>
#include <algorithm>
#include <iterator>
int main()
{
int arr[] = {1, 19, 2, 18, 4, 12, 7, 8, 10, 3, 11, 17, 9};
constexpr int length{ static_cast<int>(std::size(arr)) };
//constexpr means that value of a variable can appear in a constant expression.
// Step through each element of the array
for (int startIndex{ 0 }; startIndex < length - 1; ++startIndex)
{
int smallestIndex{ startIndex };
// Then look for a smaller element in the rest of the array
for (int currentIndex{ startIndex + 1 }; currentIndex < length; ++currentIndex)
{
if (arr[currentIndex] < arr[smallestIndex])
smallestIndex = currentIndex;
}
// swap our start element with our smallest element
std::swap(arr[startIndex], arr[smallestIndex]);
}
// Now that the whole array is sorted, print it.
for (int index{ 0 }; index < length; ++index)
std::cout << arr[index] << ' ';
std::cout << '\n';
return 0;
}
您也可以只使用std::sort
。 它在 header 文件#include<algorithm>
中,默认情况下只是按升序排序。 您还需要 header 文件#include <iterator>
for std::size
#include <iostream>
#include <algorithm>
#include <iterator>
int main()
{
int arr[] = {1, 19, 2, 18, 4, 12, 7, 8, 10, 3, 11, 17, 9};
std::sort(std::begin(arr), std::end(arr));
for (int i{ 0 }; i < static_cast<int>(std::size(arr)); ++i)
std::cout << array[i] << ' ';
std::cout << '\n';
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.