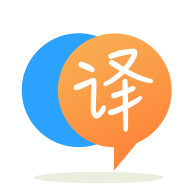
[英]How to fix “Exception thrown: read access violation. **Surface** was nullptr. occurred” in SDL2 C++
[英]C++ How do I fix Exception thrown: write access violation. this was nullptr. on Line 20
*创建一个简单的链表程序来创建一个class链表包含
class node {
void *info;
node *next;
public:
node (void *v) {info = v; next = 0; }
void put_next (node *n) {next = n;}
node *get_next ( ) {return next;}
void *get_info ( ) {return info;}
};
能够初步填写清单。 提供插入/追加节点和从链表中删除节点的功能。 能够显示列表的内容。 编写一个小驱动程序,在插入/追加、删除和显示数据时至少传入 5 个值(以便创建 5 个节点),显示程序操作。 Output:显示数据时,确保使用信息标签——使用术语“插入”、“附加”、“删除”和任何其他显示操作的术语。 显示的所有数据必须以导师易于阅读和理解的方式显示。*
我的代码运行但它抛出异常抛出:写访问冲突。 这是空指针。 调试时在第 20 行。 在 output 中,它还在我列表的 Delete 04 处停止,并且在第 156 行之后不再输出任何数据。我不知道如何解决这个问题。 我试图用另一个 cout 语句强制它到 output 新列表,但这没有用。 任何援助将不胜感激。 完整代码如下。
#include <iostream>
#include <iomanip>
using namespace std;
class Node
{
void* info;
Node* next;
public:
Node(void* v) { info = v; next = 0; }
void put_next(Node* n)
{
next = n;
}
Node* get_next()
{
return next;
}
void* get_info()
{
return info;
}
};
class List {
Node* head;
public:
List() { head = NULL; };
void PRINT();
void APPEND(void* info);
void DELETE(void* info);
};
void List::PRINT() {
Node* temp = head;
if (temp == NULL)
{
cout << "Enter Values" << endl;
return;
}
if (temp->get_next() == NULL)
{
cout << *(int*)temp->get_info();
cout << " => ";
cout << "Invalid" << endl;
}
else
{
while (temp != NULL)
{
cout << *(int*)temp->get_info();
cout << " --> ";
temp = temp->get_next();
}
cout << "Invalid" << endl;
}
}
void List::APPEND(void* info) {
Node* newNode = new Node(info);
newNode->put_next(NULL);
Node* temp = head;
if (temp != NULL)
{
while (temp->get_next() != NULL)
{
temp = temp->get_next();
}
temp->put_next(newNode);
}
else
{
head = newNode;
}
}
void List::DELETE(void* info) {
Node* temp = head;
if (temp == NULL)
{
cout << "Enter Values" << endl;
return;
}
if (temp->get_next() == NULL)
{
if ((int*)(temp->get_info()) == info)
{
delete temp;
head = NULL;
}
}
else
{
Node* previous = NULL;
while (temp != NULL)
{
if ((int*)(temp->get_info()) == info) break;
previous = temp;
temp = temp->get_next();
}
previous->put_next(temp->get_next());
delete temp;
}
}
int main()
{
List list;
int a = 04;
int b = 11;
int c = 12;
int d = 15;
int e = 29;
int* Node1 = &a;
int* Node2 = &b;
int* Node3 = &c;
int* Node4 = &d;
int* Node5 = &e;
cout << "Append: 04" << endl;
list.APPEND(Node1);
cout << "Append: 11" << endl;
list.APPEND(Node2);
cout << "Append: 12" << endl;
list.APPEND(Node3);
cout << "Append: 15" << endl;
list.APPEND(Node4);
cout << "Append: 29" << endl;
list.APPEND(Node5);
cout << endl << "Print List" << endl;
list.PRINT();
cout << endl << "Delete 04" << endl;
list.DELETE(Node1);
list.PRINT();
cout << "Start New List" << endl;
cout << endl << "Delete 12" << endl;
list.DELETE(Node3);
list.PRINT();
return 0;
}
解决方案:
//Node.h
#pragma once
class Node
{
void* info;
Node* next;
public:
Node(void* v) { info = v; next = 0; }
void put_next(Node* n)
{
next = n;
}
Node* get_next()
{
return next;
}
void* get_info()
{
return info;
}
};
//List.h
#pragma once
#include "Node.h"
#include <iomanip>
class List {
Node* head;
public:
List() { head = NULL; };
void PRINT();
void APPEND(void* info);
void DELETE(void* info);
};
//List.cpp
#include <iostream>
#include "List.h"
using namespace std;
void List::PRINT() {
Node* temp = head;
if (temp == NULL)
{
cout << "Enter Values" << endl;
return;
}
if (temp->get_next() == NULL)
{
cout << *(int*)temp->get_info();
cout << " => ";
cout << "Invalid" << endl;
}
else
{
while (temp != NULL)
{
cout << *(int*)temp->get_info();
cout << " --> ";
temp = temp->get_next();
}
cout << "Invalid" << endl;
}
}
void List::APPEND(void* info) {
Node* newNode = new Node(info);
newNode->put_next(NULL);
Node* temp = head;
if (temp != NULL)
{
while (temp->get_next() != NULL)
{
temp = temp->get_next();
}
temp->put_next(newNode);
}
else
{
head = newNode;
}
}
void List::DELETE(void* info) {
Node* temp = head;
if (temp == NULL)
{
cout << "Enter Values" << endl;
return;
}
if (temp->get_next() == NULL)
{
if ((int*)(temp->get_info()) == info)
{
delete temp;
head = NULL;
}
}
else
{
Node* previous = NULL;
while (temp != NULL)
{
if ((int*)(temp->get_info()) == info) {
// if node is head then changing the head
if (temp == head)
head = temp->get_next();
break;
}
previous = temp;
temp = temp->get_next();
}
// skip this operation if node is head
if (temp->get_next() != head)
previous->put_next(temp->get_next());
delete temp;
}
}
//Main.cpp
#include <iostream>
#include "List.h"
using namespace std;
int main()
{
List list;
int a = 04;
int b = 11;
int c = 12;
int d = 15;
int e = 29;
int* Node1 = &a;
int* Node2 = &b;
int* Node3 = &c;
int* Node4 = &d;
int* Node5 = &e;
cout << "Append: 04" << endl;
list.APPEND(Node1);
cout << "Append: 11" << endl;
list.APPEND(Node2);
cout << "Append: 12" << endl;
list.APPEND(Node3);
cout << "Append: 15" << endl;
list.APPEND(Node4);
cout << "Append: 29" << endl;
list.APPEND(Node5);
cout << endl << "Print List" << endl;
list.PRINT();
cout << endl << "Delete 04" << endl;
list.DELETE(Node1);
list.PRINT();
cout << "Start New List" << endl;
cout << endl << "Delete 12" << endl;
list.DELETE(Node3);
list.PRINT();
return 0;
}
因此,每当您删除first
节点时,您都错过了将 head 设置为head
的next node of head
。
注释DELETE
function 的else
语句中修改的部分
代码:
else
{
Node* previous = NULL;
while (temp != NULL)
{
if ((int*)(temp->get_info()) == info){
// if node is head then changing the head
if (temp == head)
head = temp->get_next();
break;
}
previous = temp;
temp = temp->get_next();
}
// skip this operation if node is head
if (temp->get_next() != head)
previous->put_next(temp->get_next());
delete temp;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.