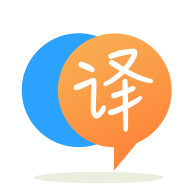
[英]if ( (my_fgets(line, max, fp)) == NULL) warning :comparison between pointer and integer [enabled by default]
[英]if(fp != EOF) -> Warning: Comparison between pointer and integer
#include <stdio.h>
#include <stdlib.h>
int main()
{
FILE *fp;
char written[]= "This is not as easy as I thought it would be.";
fp = fopen("Aufgabe 3.txt", "w");
if(fp != EOF)
{
fprintf(fp, "%s", written);
printf("Text was written!\n");
}
else
{
printf("File can not be found!");
}
fclose(fp);
return 0;
}
嗨,我是编码新手,需要一点帮助。 :D
有谁知道如何摆脱警告,我只是想将一句话写成a.txt。
行警告:10 if(fp != EOF)
:
指针与integer比较
The fopen
function returns a pointer to the newly opened file stream or, on error, a NULL
pointer (which is not the same as the EOF
integer constant). 改用这个:
if(fp != NULL)
{
fprintf(fp, "%s", written);
printf("Text was written!\n");
}
else
{
printf("File can not be found!");
}
The variable fp is a pointer so you should compare it with NULL, not EOF which is an integer (see the reference documentation for fopen : https://pubs.opengroup.org/onlinepubs/9699919799/ ). 还
这是一个更正的版本:
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
const char *filename = "Aufgabe 3.txt";
const char *written = "This is not as easy as I thought it would be.";
FILE *fp;
fp = fopen(filename, "w");
if (fp != NULL) {
fprintf(fp, "%s\n", written);
printf("Text was written!\n");
fclose(fp);
} else {
fprintf(stderr, "Opening file '%s' failed: %s:\n", filename, strerror(errno));
exit(EXIT_FAILURE);
}
return 0;
}
EOF
是负 integer 常数的宏。 fp
是指向FILE
的指针。
如果你使用
if (fp != EOF)
您将指针与非零值的 integer 进行比较,这是不允许的。
用NULL
替换EOF
,这是一个用指针检查错误的宏:
if (fp != NULL)
旁注:
此外,您应该只在指向已成功打开的 stream 的指针上使用fclose()
。 如果fp
为NULL
时使用flose(fp)
,则程序具有未定义的行为。
此外,使用if (fp != NULL)
检查 stream 的打开是否没有错误,然后当 stream 成功打开文件时继续进入if
s 正文。 else如果打开不成功你把go放到else
体中。
这段代码可以简化。
只需检查是否发生了错误,如果是,则通过if
正文中的错误例程。 如果不是,则控制流立即继续到if
语句之后的main()
中的代码。 不需要else
。
您的错误例程应该在生产代码中得到增强。 仅仅打印出文件打开不成功是不够的。
#include <stdio.h>
#include <stdlib.h>
int main (void)
{
FILE *fp;
char written[] = "This is not as easy as I thought it would be.";
fp = fopen("Aufgabe 3.txt", "w");
if (fp == NULL)
{
perror("fopen: ");
exit(EXIT_FAILURE);
}
fprintf(fp, "%s", written);
printf("Text was written!\n");
fclose(fp);
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.