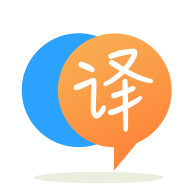
[英]How to use strstr() function to compare content in character arrays in C?
[英]How to use strstr() function?
我有两个文件blacklist.txt和email.txt 。 blacklist.txt包含一些域名。 email.txt还包含一些域名。 我必须比较两个文件,并使用strstr()
function 将blacklist.txt的域名查找到email.txt中。 以下是我编写的代码。 这段代码的问题是它返回 output NULL
而不是匹配的文本/域名。
#include <stdio.h>
#include <string.h>
#define MAXCHAR 1000
int main() {
FILE *fp, *fp1;
char str[MAXCHAR];
char str2[MAXCHAR];
char *result;
fp = fopen("blacklist.txt", "r");
fp1 = fopen("email.txt", "r");
if (fp == NULL || fp1 == NULL) {
printf("\n Cannot open one of the files %s %s \n", fp, fp1);
//return = 0;
if (fp != NULL) fclose(fp);
if (fp1 != NULL) fclose(fp1);
}
while (fgets(str, MAXCHAR, fp) != NULL || fgets(str2, MAXCHAR, fp1) != NULL)
//printf("%s, %s", str,str2);
fclose(fp);
fclose(fp1);
result = strstr(str, str2);
printf("The substring starting from the given string: %s", result);
return 0;
}
以下是对您的代码的一些说明:
FILE*
指针而不是文件名。while
循环的主体。strstr()
的匹配意味着来自第二个文件的行 sa 来自第一个文件的行的后缀。 如果返回值是缓冲区的开头,则为域匹配;如果前一个字符为 ,则为子域的匹配.
.这是修改后的版本:
#include <stdio.h>
#include <string.h>
#define MAXCHAR 1000
int main() {
FILE *fp, *fp2;
char str[MAXCHAR];
char str2[MAXCHAR];
// open and check blacklist.txt
fp = fopen("blacklist.txt", "r");
if (fp == NULL) {
printf("Cannot open %s\n", "blacklist.txt");
return 1;
}
// open and check email.txt
fp2 = fopen("email.txt", "r");
if (fp2 == NULL) {
printf("Cannot open %s\n", "email.txt");
fclose(fp);
return 1;
}
// for each line in blacklist.txt
while (fgets(str, MAXCHAR, fp) != NULL) {
// restart from the beginning of email.txt
rewind(fp2);
// for each line of email.txt
while (fgets(str2, MAXCHAR, fp2) != NULL) {
// check for a domain match
char *p = strstr(str, str2);
if (p != NULL && (p == str || p[-1] == '.')) {
// compute the length of the domains (excluding the newline)
int n = strcspn(str, "\n");
int n2 = strcspn(str2, "\n");
// output the message with the matching domains
printf("domain match on %.*s for %.*s\n", n2, str2, n, str);
}
}
}
fclose(fp);
fclose(fp2);
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.