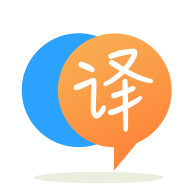
[英]How to append text to the richTextBox from another class in C# Winform?
[英]How to use a string from another class in c# winform application?
所以我在 Visual Studio C# 中编写了一个 winform 应用程序,它使用生成的密钥锁定用户类型的消息。 用户需要知道密钥的创建日期,该日期是从用户键入“textBox1”时程序生成的文件中收集的。 我正在做的是使用字符串来验证“password.txt”文件的创建日期,如果不是用户输入的日期,则消息将不会打开。 问题虽然我在使用 static 属性时遇到问题,但此错误:
错误 CS0120 非静态字段、方法或属性“Encryptor1.Encrypt(string)”UniveralWindowsTextPGP C:\Users\keife\source\repos\UniveralWindowsTextPGP\Univeral\UniveralWindowsTextPGP\UniveralWindowsTextPGP 需要 object 引用
这是我实现加密和解密方法的地方:
namespace UniveralWindowsTextPGP
{
class Encryptor1
{
public static string IV = "1a1a1a1a1a1a1a1a";
public static string Key = "1a1a1a1a1a1a1a1a1a1a1a1a1a1a1a13";
public static string Encrypt(string decrypted)
{
byte[] textbytes = ASCIIEncoding.ASCII.GetBytes(decrypted);
AesCryptoServiceProvider endec = new AesCryptoServiceProvider();
endec.BlockSize = 128;
endec.KeySize = 256;
endec.IV = ASCIIEncoding.ASCII.GetBytes(IV);
endec.Key = ASCIIEncoding.ASCII.GetBytes(Key);
endec.Padding = PaddingMode.PKCS7;
endec.Mode = CipherMode.CBC;
ICryptoTransform icrypt = endec.CreateEncryptor(endec.Key, endec.IV);
byte[] enc = icrypt.TransformFinalBlock(textbytes, 0, textbytes.Length);
icrypt.Dispose();
return Convert.ToBase64String(enc);
}
public static string Decrypted(string encrypted)
{
DateTime creation = File.GetCreationTime(@"C:\password.txt");
FileInfo fi = new FileInfo(@"C:\password.txt");
var created = fi.CreationTime;
if (fi.CreationTime != Form2.keyhere)
// 上面一行是这部分发生错误的地方。
{
string message = "That is incorrect, access is denied.";
MessageBox.Show(message);
}
else
{
byte[] textbytes = Convert.FromBase64String(encrypted);
AesCryptoServiceProvider endec = new AesCryptoServiceProvider();
endec.BlockSize = 128;
endec.KeySize = 256;
endec.IV = ASCIIEncoding.ASCII.GetBytes(IV);
endec.Key = ASCIIEncoding.ASCII.GetBytes(Key);
endec.Padding = PaddingMode.PKCS7;
endec.Mode = CipherMode.CBC;
ICryptoTransform icrypt = endec.CreateDecryptor(endec.Key, endec.IV);
byte[] enc = icrypt.TransformFinalBlock(textbytes, 0, textbytes.Length);
icrypt.Dispose();
return System.Text.ASCIIEncoding.ASCII.GetString(enc);
}
}
} }
// 这里是表格:
namespace UniveralWindowsTextPGP
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void Form2_Load(object sender, EventArgs e)
{
}
private void richTextBox1_TextChanged(object sender, EventArgs e)
{
}
private void label2_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
}
private void passwordbox_TextChanged(object sender, EventArgs e)
{
}
private void richTextBox1_TextChanged_1(object sender, EventArgs e)
{
}
private void Decrypt_Click(object sender, EventArgs e)
{
string dir = richTextBox1.Text;
StreamReader sr = new StreamReader("encryptedmessagehere.txt");
string line = sr.ReadLine();
richTextBox1.Text = Encryptor1.Decrypted(Convert.ToString(line));
}
private void Form2_Load_1(object sender, EventArgs e)
{
}
private void label3_Click(object sender, EventArgs e)
{
}
private void button1_Click_1(object sender, EventArgs e)
{
string dir = richTextBox2.Text;
string enctxt = Encryptor1.Encrypt(richTextBox2.Text);
System.IO.File.WriteAllText(@"C:\encryptedmessagehere.txt", enctxt);
}
private void label4_Click(object sender, EventArgs e)
{
}
private void richTextBox2_TextChanged(object sender, EventArgs e)
{
richTextBox2.EnableContextMenu();
}
private void button2_Click(object sender, EventArgs e)
{
string dir = richTextBox2.Text;
StreamReader sr = new StreamReader(@"C:\encryptedmessagehere.txt");
string line = sr.ReadLine();
richTextBox2.Text = Encryptor1.Decrypted(Convert.ToString(line));
System.IO.File.WriteAllText(@"C:\decryptedmessagehere.txt", line);
}
private void button3_Click(object sender, EventArgs e)
{
Form3 f3 = new Form3();
f3.Show();
}
private void button4_Click(object sender, EventArgs e)
{
Form f4 = new Form4();
f4.Show();
}
public void passwordbox1_TextChanged(object sender, EventArgs e)
{
}
private void richTextBox3_TextChanged(object sender, EventArgs e)
{
string richTextBox3 = "";
}
public void textBox1_TextChanged(object sender, EventArgs e)
{
TextWriter txt = new StreamWriter("C:/password.txt");
txt.Write(textBox1.Text);
txt.Close();
public string keyhere = textBox1.Text;
// 这是这里发生错误的地方。
非常感谢,感谢您的想法。
这一行在这里:
public string keyhere = textBox1.Text;
在textBox1_TextChanged
方法中。
您在此行中使用访问 static 变量的语法:
if (fi.CreationTime.= Form2.keyhere)
没有 static 变量Form2.keyhere
。 这就是编译器抱怨的原因。
您可以通过创建一个来解决这两个问题:
public static string keyhere
public void textBox1_TextChanged(object sender, EventArgs e)
{
TextWriter txt = new StreamWriter("C:/password.txt");
txt.Write(textBox1.Text);
keyhere = textBox1.Text;
txt.Close(); // write this as last call, otherwise textBox1 might already be disposed when you try to access textBox1.Text;
}
免责声明:这是一个临时解决方案,它违反了干净编码的某些规则。 特别是单一责任原则。 最好将所有必要的信息传递给Decrypted
方法并在那里使用它。 class Encryptor1
不应该知道有关Form
或其他 UI 元素的任何信息。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.