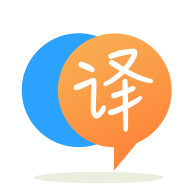
[英]I'm new in firebase and I'm trying to get id document for parent collection but I can't figure it out
[英]I'm trying to get my firestore document id
我有一个需要连接到我的帖子(“想法”)的评论子集合,但是我无法将文档 ID 动态添加到我的 doc() 中。 由于没有其他解决方案,我目前将其留空。 因此,它正在生成一个新的文档 ID,而不是加入其父文档。
doc(post.id) 不起作用,因为 post 没有在我的 addComments 或 getComments 中定义,而只是 doc(id) 由于同样的原因而不起作用。
这是它目前正在做的事情
这就是我想要它做的。 出于演示目的,我手动输入了这篇文章的文档 ID:
这是我的 fire.js
import FirebaseKeys from "./config/FirebaseKeys";
import firebase from "firebase/app";
import '@firebase/auth';
import 'firebase/database';
import '@firebase/firestore';
import "firebase/storage";
require("firebase/firestore");
class Fire {
constructor() {
firebase.initializeApp(FirebaseKeys);
}
getPosts = async (displayLatestPost) => {
const post = await this.firestore.collection('thoughts').orderBy('timestamp', 'desc').limit(10).get()
let postArray =[]
post.forEach((post) => {
postArray.push({id: post.id, ...post.data()})
})
displayLatestPost(postArray)
}
getComments = async (latestComment) => {
const fetchedComment = await this.firestore.collection('thoughts').doc().collection('comments').orderBy('timestamp', 'desc').limit(10).get()
let commentArray = []
fetchedComment.forEach((comment) => {
commentArray.push({id: comment.id, ...comment.data()})
})
console.log(" post id: " + post.id + "comment id: " + fetchedComment.id)
latestComment(commentArray)
}
addPost = async ({ thoughtTitle, thoughtText, localUri, photoURL, displayName}) => {
const remoteUri = await this.uploadPhotoAsync(localUri, `photos/${this.uid}/${Date.now()}`);
return new Promise((res, rej) => {
this.firestore
.collection("thoughts")
.add({
uid: this.uid,
displayName,
photoURL,
thoughtTitle,
thoughtText,
image: remoteUri,
timestamp: this.timestamp,
})
.then(ref => {
res(ref);
})
.catch(error => {
rej(error);
});
});
};
//comments
addComments = async ({ displayName, photoURL, commentText}) => {
console.log("add a comment - post id:" )
return new Promise((res, rej) => {
this.firestore
.collection("thoughts")
.doc()
.collection('comments')
.add({
uid: this.uid,
displayName,
photoURL,
commentText,
timestamp: this.timestamp,
})
.then(ref => {
res(ref);
})
.catch(error => {
rej(error);
});
});
};
//comments
uploadPhotoAsync = (uri, filename) => {
return new Promise(async (res, rej) => {
const response = await fetch(uri);
const file = await response.blob();
let upload = firebase
.storage()
.ref(filename)
.put(file);
upload.on(
"state_changed",
snapshot => {},
err => {
rej(err);
},
async () => {
const url = await upload.snapshot.ref.getDownloadURL();
res(url);
}
);
});
};
createUser = async user => {
let remoteUri = null;
try {
await firebase.auth().createUserWithEmailAndPassword(user.email, user.password);
let db = this.firestore.collection("users").doc(this.uid);
db.set({
displayName: user.displayName,
email: user.email,
photoURL: null
});
if (user.photoURL) {
remoteUri = await this.uploadPhotoAsync(user.photoURL, `avatars/${this.uid}`);
db.set({ photoURL: remoteUri }, { merge: true });
}
} catch (error) {
alert("Error: ", error);
}
};
updateProfile = async user => {
let remoteUri = null;
try {
let db = this.firestore.collection("users").doc(this.uid);
db.update({
displayName: user.displayName,
photoURL: user.photoURL
});
if (user.photoURL) {
remoteUri = await this.uploadPhotoAsync(user.photoURL, `avatars/${this.uid}`);
db.set({ photoURL: remoteUri }, { merge: true });
}
} catch (error) {
alert("Error: ", error);
}
}
signOut = () => {
firebase.auth().signOut();
};
get firestore() {
return firebase.firestore();
}
get uid() {
return (firebase.auth().currentUser || {}).uid;
}
get timestamp() {
return Date.now();
}
}
Fire.shared = new Fire();
export default Fire;
这是添加评论的屏幕。
import React from "react";
import { View, StyleSheet, Text, Image, ScrollView, TextInput, FlatList,
TouchableOpacity} from "react-native";
import Fire from '../../Fire';
import CreatedAtText from '../../components/Text/CreatedAtText';
import Colors from '../../constants/Colors';
import moment from 'moment';
import ThoughtCommentItem from '../../components/Thought/ThoughtCommentItem';
import FloatingButton from '../../components/UI/FloatingButton';
export default class ThoughtDetailsScreen extends React.Component {
state = {
commentText: "",
latestComments: [],
user: {
photoURL: "",
displayName: ""
}
};
handleComment = () => {
Fire.shared
.addComments({ commentText: this.state.commentText.trim(), photoURL: this.state.user.photoURL, displayName: this.state.user.displayName })
.then(ref => {
this.setState({ commentText: ""});
})
.catch(error => {
alert(error);
});
};
displayLatestComment = (latestComments) => {
this.setState({latestComments: latestComments});
console.log("Latest Comments Details" + JSON.stringify(latestComments));
}
componentDidMount(){
Fire.shared.getComments(this.displayLatestComment);
}
unsubscribe = null;
componentDidMount() {
const user = this.props.uid || Fire.shared.uid;
this.unsubscribe = Fire.shared.firestore
.collection("users")
.doc(user)
.onSnapshot(doc => {
this.setState({ user: doc.data() });
});
}
componentWillUnmount() {
this.unsubscribe();
}
comment = () => {
console.log("comment button clicked ")
}
like = () => {
console.log("like button clicked ")
}
fave = () => {
console.log("fave button clicked ")
}
render(){
const thought = this.props.navigation.getParam('thought');
return(
<View style={styles.container}>
<ScrollView style={{flex:1}}>
<Text>{thought.thoughtTitle}</Text>
<Image style={styles.userAvatar} source={{uri: thought.photoURL}}/>
<CreatedAtText style={styles.timestamp}> {moment(thought.timestamp).fromNow()}</CreatedAtText>
<Text> User: {thought.displayName} </Text>
<Image style={styles.headerPhoto} source={{uri: thought.image}}/>
<Text>Main text: {thought.thoughtText}</Text>
<View style={styles.commentsDisplay}>
<FlatList
showsVerticalScrollIndicator={false}
keyExtractor={item => item.id}
style={styles.feed}
data={this.state.latestComments}
renderItem={({item,index}) => {
return (
<ThoughtCommentItem
photoURL={item.photoURL}
displayName={item.displayName}
timestamp={item.timestamp}
commentText={item.commentText}
onSelect={() => this.props.navigation.navigate('CommentDetails', {comment : item})}
/>
);
}
}
/>
</View>
<View style={styles.commentContainer}>
<TextInput
style={styles.commentBox}
placeholder="Add your thoughts about this!"
onChangeText={commentText =>this.setState({commentText})}
defaultValue={this.state.commentText}
/>
<Text onPress={this.handleComment} style={styles.commentButton}> Comment
</Text>
</View>
</ScrollView>
<FloatingButton style={styles.floatingbutton}
onSelectComment={this.comment}
onSelectLike={this.like}
onSelectFavorite={this.fave}
/>
</View>
);
}
};
const styles = StyleSheet.create({
container: {
flex:1
},
commentContainer:{
flexDirection: "row"
},
headerPhoto : {
height: 250,
width: 200
},
userAvatar: {
backgroundColor: Colors.subheadings,
borderColor: Colors.accent,
borderWidth:3.5,
backgroundColor: Colors.maintext,
marginEnd: 15,
width: 35,
height: 35,
borderRadius: 20,
},
floatingbutton:{
bottom: 70,
right: 60
},
commentButton: {
color: "#788eec",
fontWeight: "bold",
fontSize: 16
},
commentsDisplay: {
flex: 1,
backgroundColor: Colors.background
},
feed : {
marginHorizontal: 16
}
});
正如您已经说过的,问题在于这段代码:
this.firestore
.collection("thoughts")
.doc()
.collection('comments')
.add({
uid: this.uid,
displayName,
photoURL,
commentText,
timestamp: this.timestamp,
为了添加子集合或文档,您首先需要一个有效的参考,在这种情况下.doc()
没有指定将包含子集合的文档。 这就是为什么你有上面的 output(截图),这就是为什么你的问题是“如何获取我的 Firestore 文档 ID”。
要动态获取 firestore 文档 id,您需要使用将生成文档 object 的查询,您需要遍历该文档以获取 id 属性,最后您将在.doc()
中使用此 id 属性。
不用说,查询中使用的过滤器必须产生一个结果; 从您共享的代码中,我看到您可以使用 uid 作为过滤器。 以下是有关如何实现此目的的代码:
const firebase = require("firebase/app");
require("firebase/firestore");
firebase.initializeApp({
projectId: 'project123'
});
var db = firebase.firestore();
function InsertSubcollection(collection,uid){
db.collection(collection).where("uid", "==", uid).get()
.then(function (querySnapshot) {
let id =0;
querySnapshot.forEach((doc) => id = doc.id);
const docRef = db.collection('thoughts').doc(id).collection('comments');
docRef.doc().add({
name: "Sample123",
test: true
});
})
.catch(function (error) {
console.log("Error getting documents: ", error);
});
}
我希望这能有所启发。
如果您想了解有关 Firestore 查询的更多信息,请访问此。
抱歉,我没有发布找到的解决方案。
需要将此行添加到添加评论的屏幕 -
handleComment = () => {
Fire.shared
.addComments({ commentText: this.state.commentText.trim(), photoURL: this.state.user.photoURL, displayName: this.state.user.displayName, postID: this.props.navigation.state.params.thought.id })
.then(ref => {
this.setState({ commentText: ""});
})
.catch(error => {
alert(error);
});
};
然后我必须将 postID 添加到我的 Fire.js addComments 中。
addComments = async ({ displayName, photoURL, commentText, postID}) => {
return new Promise((res, rej) => {
this.firestore
.collection("thoughts")
.doc(postID)
.collection('comments')
.add({
uid: this.uid,
displayName,
photoURL,
commentText,
timestamp: this.timestamp,
})
.then(ref => {
res(ref);
})
.catch(error => {
rej(error);
});
});
};
所以基本上添加的代码行是:
postID: this.props.navigation.state.params.thought.id
和 -
.doc(postID)
我真的希望这可以帮助有类似问题的人。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.