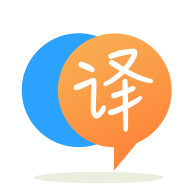
[英]How to format all HttpExceptions as json in symfony5?
[英]How to send uploded document via an url using symfony5
我正在尝试通过 symfony 发送上传到 maarch 签名的文档。 我已经用纯 php 编写了我的代码,我想在 symfony 上调整它们,但我不知道该怎么做。 下面是用php编写的代码。
表单.php
<?php
$url = "http://ggrand:maarch@169.176.61.102:5600/rest/users";
$response = file_get_contents($url);
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Envoi d'un document dans le parapheur</title>
</head>
<script type="text/javascript">
var jsonObj =<?php echo $response;?>
</script>
<body>
<form action="processJson_tableau.php" method="post" enctype="multipart/form-data">
<table>
<tr>
<td>Titre du document</td>
<td><input type="text" name="title" /></td>
</tr>
<tr>
<td>Référence du document</td>
<td><input type="text" name="reference" /></td>
</tr>
<tr>
<td>Description du document</td>
<td><input type="text" name="description" /></td>
</tr>
<tr>
<td>Type d'action</td>
<td>
<input type="radio" id="sign" name="mode" value="sign" />
<label for="male">Signature</label><br />
<input type="radio" id="note" name="mode" value="note" />
<label for="female">Anotation</label><br />
<input type="radio" id="visa" name="mode" value="visa" />
<label for="female">Visa</label><br />
</td>
</tr>
<tr>
<td>Joindre le fichier</td>
<td><input type="file" name="encodedDocument" /></td>
</tr>
<tr>
<td>Prénom et Nom de l'expéditeur</td>
<td><input type="text" name="sender" /></td>
</tr>
<tr>
<td>Nom et prénom du destinataire</td>
<td>
<select id="dropDown" name="processingUser"></select>
<script type="text/javascript">
var myDDL = document.getElementById("dropDown");
var str1 = " ";
for (i = 0; i < jsonObj.users.length; i++) {
var option = document.createElement("option");
option.text = jsonObj.users[i].firstname.concat(str1, jsonObj.users[i].lastname);
option.value = jsonObj.users[i].login;
try {
myDDL.options.add(option);
} catch (e) {
alert(e);
}
}
</script>
</td>
</tr>
<tr>
<td colspan="2"><input type="submit" name="submit" value="Start Upload"></td>
</tr>
</table>
</form>
</body>
</html>
processJson_tableau.php
<?php
$currentDirectory = getcwd();
$uploadDirectory = "/";
$errors = []; // Store errors here
$fileExtensionsAllowed = ['jpeg','jpg','png','pdf']; // These will be the only file extensions allowed
$fileName = $_FILES['encodedDocument']['name'];
$fileSize = $_FILES['encodedDocument']['size'];
$fileTmpName = $_FILES['encodedDocument']['tmp_name'];
$fileType = $_FILES['encodedDocument']['type'];
$fileExtension = strtolower(end(explode('.',$fileName)));
$uploadPath = $currentDirectory . $uploadDirectory . basename($fileName);
if (isset($_POST['submit'])) {
if (! in_array($fileExtension,$fileExtensionsAllowed)) {
$errors[] = "This file extension is not allowed. Please upload a JPEG or PNG file";
}
if ($fileSize > 4000000) {
$errors[] = "File exceeds maximum size (4MB)";
}
if (empty($errors)) {
$didUpload = move_uploaded_file($fileTmpName, $uploadPath);
if ($didUpload) {
echo "The file " . basename($fileName) . " has been uploaded";
} else {
echo "An error occurred. Please contact the administrator.";
}
} else {
foreach ($errors as $error) {
echo $error . "These are the errors" . "\n";
}
}
}
$title = $_POST['title'];
$reference = $_POST['reference'];
$desc = $_POST['description'];
$encodedDoc = $fileName;
$sender = $_POST['sender'];
$processingUser = $_POST['processingUser'];
$mode = $_POST['mode'];
$zip = new ZipArchive;
if ($zip->open('doc1.zip', ZipArchive::CREATE) === TRUE)
{
// Add files to the zip file
$zip->addFile($encodedDoc);
// All files are added, so close the zip file.
$zip->close();
}
// Get the file and convert into string
$zip_f = file_get_contents('doc1.zip');
// Encode the zip file string data into base64
$data = base64_encode($zip_f);
// Display the output
//echo $data;
$puser = array('processingUser' =>$processingUser, 'mode' => $mode);
$document = array('title' =>$title, 'reference' =>$reference, 'description' =>$desc, 'encodedDocument' =>$data, 'sender' =>$sender,
'workflow'=>array($puser)
);
$json_response = json_encode($document);
//API Url
$url = 'http://ggrand:maarch@169.176.61.102:5600/rest/documents';
//Initiate cURL.
$ch = curl_init($url);
//Encode the array into JSON.
$jsonDataEncoded = $json_response;
//Tell cURL that we want to send a POST request.
curl_setopt($ch, CURLOPT_POST, 1);
//Attach our encoded JSON string to the POST fields.
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonDataEncoded);
//Set the content type to application/json
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json'));
//Execute the request
$result = curl_exec($ch);
echo $result;
unlink('doc1.zip');
unlink($fileName);
?>
这是我开始在 symfony 上写的控制器代码,但现在我卡住了,我不知道如何前进。
<?php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\Routing\Annotation\Route;
use App\Entity\Upload;
use App\Form\UploadType;
use Doctrine\Common\Persistence\ObjectManager;
use Doctrine\ORM\EntityManagerInterface;
use Symfony\Component\HttpFoundation\Request;
class FileController extends AbstractController
{
/**
* @Route("/home", name="accueil")
*/
public function index(Request $request, EntityManagerInterface $manager)
{
$upload = new Upload();
$form = $this->createForm(UploadType::class, $upload);
$form->handleRequest($request);
if($form->isSubmitted() && $form->isValid()) {
$manager->persist($upload);
$manager->flush();
//$enc = $upload->getDocument();
//$file = base64_encode($enc);
$file = $upload->getDocument();
$fileName = md5(uniqid()).'.'.$file->guessExtension();
$file->move($this->getParameter('upload_directory'), $fileName);
$enc = base64_encode($file);
$upload->setDocument($fileName);
return $this->redirectToRoute('accueil');
}
return $this->render('file/index.html.twig', array(
'form' => $form->createView(),
));
}
}
是否有必要使用 symfony 显示我显示表单的部分?
要上传和下载文件,您可以使用Vich 上传程序包,它允许您:
- 自动命名文件并将其保存到配置的目录
- 当文件从
数据存储作为 Symfony\\Component\\HttpFoundation\\File\\File 的一个实例- 从数据存储中删除实体或文档后,从文件系统中删除文件
- 模板助手以生成文件的公共 URL
完整文档在这里: Vich 上传器文档。
因此,要将这个包添加到您自己的代码中,您应该添加一个实体来存储文件属性和文件本身的引用。 该包提供了一个对象,可帮助您将文件存储在您想要的位置。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.