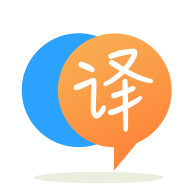
[英]How to EnumerateFiles with all subdirectories with C# DirectoryInfo?
[英]How to EnumerateFiles to only 3 files directories in C#?
目前我只能 Enumeratefiles 方法所有文件或源目录。 我希望它深入 3 个子目录,然后只检查那里。
例如,第二个片段将只检查 K:\\SourceFolder
第一个示例将检查 K:\\SourceFolder\\JobName\\Batches\\Folder1\\Folder11\\Images 它将检查所有文件夹,从而降低应用程序的性能和效率。
我只需要它来检查 K:\\SourceFolder\\JobName\\Batches
这段代码走得太远了:
List<string> validFiles = new List<string>();
List<string> files = Directory.EnumerateFiles(folderPath, "*.*", SearchOption.AllDirectories).ToList();
foreach (var file in files)
这段代码还不够:
List<string> files = Directory.Enumeratefiles(directory)
我们可以使用几种不同的方法来处理此任务,因此我将列出一些示例以帮助开始。
使用循环迭代所有文件夹并仅返回目标深度的文件夹:
List<string> ListFilesAtSpecificDepth(string rootPath, int targetDepth)
{
// Set the root folder before we start iterating through subfolders.
var foldersAtCurrentDepth = Directory.EnumerateDirectories(rootPath).ToList(); // calling ToList will make the enumeration happen now.
for (int currentDepth = 0; currentDepth < targetDepth; currentDepth++)
{
// Using select many is a clean way to select the subfolders for multiple root folders into a flat list.
foldersAtCurrentDepth = foldersAtCurrentDepth.SelectMany(x => Directory.EnumerateDirectories(x)).ToList();
}
// After the loop we have a list of folders for the targetDepth only.
// Select many again to get all the files for all the folders.
return foldersAtCurrentDepth.SelectMany(x => Directory.EnumerateFiles(x, "*.*", SearchOption.TopDirectoryOnly)).ToList();
}
另一种选择是使用递归来调用相同的方法,直到我们达到所需的深度。 这可用于仅返回目标深度处的结果或沿途的所有结果,由于上面的示例仅针对目标深度,因此我决定使用自定义对象来跟踪深度来为这个结果做所有结果:
class FileSearchResult
{
public string FilePath {get;set;}
public int FolderDepthFromRoot {get;set;}
}
List<FileSearchResult> ListFilesUntilSpecificDepth(string rootPath, int maxDepth, int currentDepth = 0)
{
// Add all the files at the current level along with extra details like the depth.
var iterationResult = Directory.EnumerateFiles(rootPath, "*.*", SearchOption.TopDirectoryOnly)
.Select(x => new FileSearchResult
{
FilePath = x,
FolderDepthFromRoot = currentDepth
}).ToList();
if (currentDepth < maxDepth) // we need to go deeper.
{
var foldersUnderMe = Directory.EnumerateDirectories(rootPath);
// Add all the results for subfolders recursively by calling the same method again.
foreach (var subFolder in foldersUnderMe)
{
iterationResult.AddRange(ListFilesUntilSpecificDepth(subFolder, maxDepth, currentDepth + 1))
}
}
return iterationResult;
}
有了递归和循环的例子,我想谈的最后一个是使用文件系统本身的结构来帮助完成这个任务。 因此,我们可以使用原始方法递归获取所有文件,然后使用所有结果的列表来确定深度,而不是管理我们自己的循环或递归。 例如,我们知道“/”是文件系统用来分隔文件夹的字符,并且在文件夹或文件名中使用它是一个非法字符,因此使用此标记来有效地计算文件夹应该是非常安全的。 在这个例子中,我将使用另一个自定义类来跟踪结果,因此它应该有效地返回与递归方法相同但具有无限深度的结果。
class FileSearchResult
{
public string FilePath { get; set; }
public int FolderDepthFromRoot { get; set; }
}
List<FileSearchResult> ListFiles(string rootPath)
{
var allFiles = Directory.EnumerateFiles(rootPath, "*.*", SearchOption.AllDirectories).ToList();
int numberOfFoldersInRootPath = rootPath.Count(c => c == '\\'); // count how many backslashes in root path as a base.
return allFiles.Select(filePath => new FileSearchResult
{
FilePath = filePath,
FolderDepthFromRoot = filePath.Count(c => c == '\\') - numberOfFoldersInRootPath
}).ToList();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.