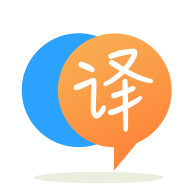
[英]kotlin / java sharedPreferences meaning in android studio
[英]SharedPreferences Java Android Studio
我试图在他们登录/注册时创建一个用户会话,以便他们在关闭应用程序后自动进入应用程序。
我不断收到此错误:
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'android.content.Context
android.content.Context.getApplicationContext()' on a null object reference
at com.example.myapplication.BackgroundWorker.<init>(BackgroundWorker.java:32)
at com.example.myapplication.LoginActivity.OnLogin(LoginActivity.java:73)
at java.lang.reflect.Method.invoke(Native Method)
at androidx.appcompat.app.AppCompatViewInflater$DeclaredOnClickListener
我正在关注这个答案https://stackoverflow.com/a/11027631/14270746
我已经在 php 和 Java 中创建了登录/注册脚本,所以我试图在我已经创建的代码中实现这些东西。 我在我的 BackgroundWorker.Java 类中执行此操作。 我尝试在我的 LoginActivity 中执行此操作,但遇到了同样的错误。 有人能帮我吗 ?
public class BackgroundWorker extends AsyncTask<String, Void, String> {
Context context;
AlertDialog alertDialog;
BackgroundWorker(Context ctx) {
context = ctx;
}
private SharedPreferences sharedpreferences;
SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(context.getApplicationContext());
SharedPreferences.Editor editor = preferences.edit();
@Override
protected String doInBackground(String... params) {
String type = params [0];
String login_url = "http://192.168.1.37/byebye/login_handler.php";
String register_url = "http://192.168.1.37/byebye/register_handler.php";
if (type.equals("login")) {
try {
String username = params [1];
String pw = params [2];
editor.putString("username", String.valueOf(username));
editor.apply();
URL url = new URL(login_url);
HttpURLConnection httpURLConnection = (HttpURLConnection)url.openConnection();
httpURLConnection.setRequestMethod("POST");
httpURLConnection.setDoOutput(true);
httpURLConnection.setDoInput(true);
OutputStream outputStream = httpURLConnection.getOutputStream();
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(outputStream, "UTF-8"));
String post_data = URLEncoder.encode("username", "UTF-8")+"="+URLEncoder.encode(username, "UTF-8")+"&"
+URLEncoder.encode("pw", "UTF-8")+"="+URLEncoder.encode(pw, "UTF-8");
bufferedWriter.write(post_data);
bufferedWriter.flush();
bufferedWriter.close();
outputStream.close();
InputStream inputStream = httpURLConnection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream, "iso-8859-1"));
String result = "";
String line = "";
while ((line = bufferedReader.readLine()) != null){
result += line;
}
bufferedReader.close();
inputStream.close();
httpURLConnection.disconnect();
return result;
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
} else if (type.equals("register")) {
try {
String name = params [1];
String username = params [2];
String email = params [3];
String pw = params [4];
URL url = new URL(register_url);
HttpURLConnection httpURLConnection = (HttpURLConnection)url.openConnection();
httpURLConnection.setRequestMethod("POST");
httpURLConnection.setDoOutput(true);
httpURLConnection.setDoInput(true);
OutputStream outputStream = httpURLConnection.getOutputStream();
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(outputStream, "UTF-8"));
String post_data = URLEncoder.encode("name", "UTF-8")+"="+URLEncoder.encode(name, "UTF-8")+"&"
+URLEncoder.encode("username", "UTF-8")+"="+URLEncoder.encode(username, "UTF-8")+"&"
+ URLEncoder.encode("email", "UTF-8")+"="+URLEncoder.encode(email, "UTF-8")+"&"
+ URLEncoder.encode("pw", "UTF-8")+"="+URLEncoder.encode(pw, "UTF-8");
bufferedWriter.write(post_data);
bufferedWriter.flush();
bufferedWriter.close();
outputStream.close();
InputStream inputStream = httpURLConnection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream, "iso-8859-1"));
String result = "";
String line = "";
while ((line = bufferedReader.readLine()) != null){
result += line;
}
bufferedReader.close();
inputStream.close();
httpURLConnection.disconnect();
return result;
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
@Override
protected void onPreExecute() {
alertDialog = new AlertDialog.Builder(context).create();
alertDialog.setTitle("Status");
}
@Override
protected void onPostExecute(String result) {
alertDialog.setMessage(result);
alertDialog.show();
Intent intent = new Intent(context.getApplicationContext(), ProfileActivity.class);
context.startActivity(intent);
}
@Override
protected void onProgressUpdate(Void... values) {
super.onProgressUpdate(values);
}
}
这是第一个错误行(BackgroundWorker.java:32):
SharedPreferences preferences =
PreferenceManager.getDefaultSharedPreferences(context.getApplicationContext());
这是第二个:
BackgroundWorker backgroundWorker = new BackgroundWorker(this);
我的猜测是变量没有保存到 sharedpref。
内联变量初始值设定项在构造函数体运行之前被调用。 因此,当您尝试初始化preferences
, context
的值仍然为 null,因为尚未调用构造函数。 将初始化程序放在文件中的构造函数之后并不重要,它仍然首先被调用。 您应该在构造函数内部初始化它。
此外,不要只保留对SharedPreferences.Editor
的引用。 需要时在本地创建它。
public class BackgroundWorker extends AsyncTask<String, Void, String> {
Context context;
SharedPreferences preferences;
AlertDialog alertDialog;
BackgroundWorker(Context ctx) {
context = ctx;
preferences = PreferenceManager.getDefaultSharedPreferences(ctx.getApplicationContext());
}
@Override
protected String doInBackground(String... params) {
SharedPreferences.Editor editor = preferences.edit();
String type = params [0];
String login_url = "http://192.168.1.37/byebye/login_handler.php";
String register_url = "http://192.168.1.37/byebye/register_handler.php";
// ...
}
// ...
}
您应该在您的活动类中初始化您的SharedPreference
。 因为 AsyncTask 在后台工作。 因此,当您的应用程序关闭时,AsyncTask 仍然存在,但您的应用程序不在前台。 这就是getApplicationContext()
提供 null 的原因。 因此,为了防止此错误,请在活动onCreate()
方法中初始化SharedPreference
然后使用它。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.