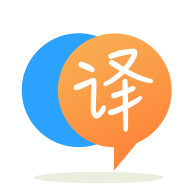
[英]Is there an elegant way to change what methods/variables an object of a class can use based on the constructor used to create this object?
[英]Can variables be used to create object in Java?
我怎么做这样的事情? 我想使用像Circle 10
或Rectangle 20 30
这样的命令行参数并创建相应类的对象。
class Circle{
//Some lines here
}
class Rectangle{
//Some lines here
}
public class Perimeter{
public static void main(String[] args) {
for(int i=0; i< args.length; i++){
String shape = args[0];
shape curr_shape = new shape();
System.out.println(curr_shape.getPerimeter( <arguments> ));
}
}
}
您可以使用Class.forName()
和反射来创建一个实例,就像@Milgo 建议的那样,但我建议创建一个简单的switch/case
。 您的任务看起来像是一些培训,所以这可能是您的最佳选择。
Object form;
switch (type) {
case "Circle":
form = new Circle();
break;
case "Rectangle":
form = new Rectangle();
break;
}
尽管您可以使用反射(即黑暗艺术),但如果所有形状都具有相同的构造函数签名,例如List<Double>
(其中包括像您的示例一样的整数),则可以使用最少的代码来完成:
private static Map<String, Function<List<Double>, Shape>> factories =
Map.of("Circle", Circle::new, "Rectangle", Rectangle::new); // etc
public static Shape createShape(String input) {
String[] parts = input.split(" ");
String name = parts[0];
List<Double> dimensions = Arrays.stream(parts)
.skip(1) // skip the name
.map(Double::parseDouble) // convert String terms to doubles
.collect(toList());
return factories.get(name).apply(dimensions); // call constructor
}
interface Shape {
double getPerimeter();
}
static class Rectangle implements Shape {
private double height;
private double width;
public Rectangle(List<Double> dimensions) {
height = dimensions.get(0);
width = dimensions.get(1);
}
@Override
public double getPerimeter() {
return 2 * (height + width);
}
}
static class Circle implements Shape {
double radius;
public Circle(List<Double> dimensions) {
radius = dimensions.get(0);
}
@Override
public double getPerimeter() {
return (double) Math.PI * radius * radius;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.