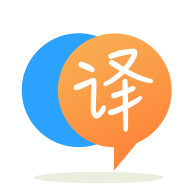
[英]Recieving a java.lang.NullPointerException when running my project in Page object model with selenium webdriver
[英]java.lang.NullPointerException exception is occurred when running the test script Page Object Model using Selenium Web Driver
package com.qa.base;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import com.qa.util.TestUitl;
public class TestBase {
public static WebDriver driver;
public static Properties prop;
// Creating a Constructor
public TestBase() {
prop = new Properties();
try {
File file = new File("/APIAutomation/CRMTest/src/main/java/com/qa/config/config.properties");
FileInputStream fis = new FileInputStream(file);
prop.load(fis);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void initializtion() {
String browser = prop.getProperty("browser");
if(browser.equals("chrome")) {
System.setProperty("webdriver.chrome.driver", "C:/APIAutomation/chromedriver.exe");
driver = new ChromeDriver();
}
else
{
System.out.println("Quit Running Script");
}
driver.manage().window().maximize();
driver.manage().deleteAllCookies();
driver.manage().timeouts().implicitlyWait(TestUitl.PAGE_LOAD_TIMEOUT, TimeUnit.SECONDS);
driver.manage().timeouts().implicitlyWait(TestUitl.IMPLICIT_WAIT, TimeUnit.SECONDS);
driver.get(prop.getProperty("url"));
}
}
Page Factory
package com.qa.pages;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
import com.qa.base.TestBase;
public class loginPage extends TestBase{
//Page Factory
@FindBy(name="email")
WebElement email;
@FindBy(name="password")
WebElement password;
@FindBy(xpath=".//div[@class='ui fluid large blue submit button']")
WebElement loginButton;
@FindBy(xpath="//div[@class ='column']/div[2][@class='ui message']")
WebElement OldLogin;
//Initialization the Page Object
public loginPage() {
PageFactory.initElements(driver, this);
}
//Actions
public String validateLoginPageTitle() {
return driver.getTitle();
}
public String validateUserName() {
return OldLogin.getText();
}
public HomePage login(String user, String pass) {
email.sendKeys(user);
password.sendKeys(pass);
loginButton.click();
return new HomePage();
}
}
Page Factory HomePage
package com.qa.pages;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
import com.qa.base.TestBase;
public class HomePage extends TestBase{
//PageFactory
@FindBy(xpath = ".//div[@id='main-nav']/a[@href='/home']")
WebElement Homepage;
@FindBy(xpath =".//div/span[@class='user-display']")
WebElement username;
@FindBy(xpath =".//span[contains(text(),'Contacts')]")
WebElement contactlink;
@FindBy(xpath="//a[5][contains(@href,'/deals')]")
WebElement dealslink;
//Initializing Page Object
public HomePage() {
PageFactory.initElements(driver, this);
}
//Actions
public String validateHomePage() {
return driver.getTitle();
}
public boolean validateHomePageDisplayed() {
return Homepage.isDisplayed();
}
public String validateUser() {
return username.getText();
}
public boolean verifyUsername() {
return username.isDisplayed();
}
public ContactPage clickContactLink() {
contactlink.click();
return new ContactPage();
}
public DealsPage clickDeals() {
dealslink.click();
return new DealsPage();
}
}
Test Case Class
package com.crm.qa.TestCases;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import com.qa.base.TestBase;
import com.qa.pages.ContactPage;
import com.qa.pages.HomePage;
import com.qa.pages.loginPage;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedCondition;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.Assert;
public class HomePageTest extends TestBase {
loginPage loginpage;
HomePage homepage;
ContactPage contactpage;
//Create a constructor of HomePageTest and call the super class i.e the TestBase class constructor will be called
public HomePageTest() {
super();
}
// test case should be separated - independent with each other
// before each test cases - launch the browser and login
// after each test cases - close the browser
@BeforeMethod
public void setUp() {
initializtion();
loginpage = new loginPage();
//Login method is returning homepage object
homepage = loginpage.login(prop.getProperty("username"), prop.getProperty("password"));
}
@Test
public void verifyHomePageTitleTest() {
String homepageTitle = homepage.validateHomePage();
System.out.println("Print HomePage Title:::::::::::::::::" +homepageTitle);
Assert.assertEquals(homepageTitle, "Cogmento CRM","Homepage title is not matching");
}
@Test
public void verifyUsernameTest() {
Assert.assertTrue(homepage.verifyUsername(), "Username is not matching");
}
@Test
public void verifyUserTest() {
String Username = homepage.validateUser();
Assert.assertEquals(Username, "Gokul Kuppusamy","Username text is not matching");
}
@Test
public void verifyContactPageTest() {
System.out.println("Clicking Contact Page");
contactpage = homepage.clickContactLink();
}
@AfterMethod
public void tearDown() {
driver.quit();
}
}
package com.crm.qa.TestCases;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.Assert;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import com.qa.base.TestBase;
import com.qa.pages.ContactPage;
import com.qa.pages.HomePage;
import com.qa.pages.loginPage;
public class ContactPageTest extends TestBase {
loginPage loginpage;
HomePage homepage;
ContactPage contactpage;
public ContactPageTest() {
super();
}
@BeforeMethod
public void setUp() throws Exception {
initializtion();
loginpage = new loginPage();
// Login method is returning homepage object
homepage = loginpage.login(prop.getProperty("username"), prop.getProperty("password"));
driver.manage().timeouts().implicitlyWait(5000, TimeUnit.SECONDS);
Thread.sleep(3000);
homepage.validateHomePageDisplayed();
/*
* Thread.sleep(3000); homepage.clickContactLink(null);
*/
}
@Test
public void verifyContactsPageNewButtonTest() {
Assert.assertTrue(contactpage.verifyNewButton());
}
@Test(priority = 1)
public void verifyContactsPageLableTest() {
driver.manage().timeouts().implicitlyWait(5000, TimeUnit.SECONDS);
Assert.assertTrue(contactpage.verifyContactlable(), "Contacts is missing on the page");
}
@Test(priority = 2)
public void selectContactDetailsTest() {
contactpage.selectContactDetails();
}
@AfterMethod
public void tearDown() {
driver.quit();
}
}
java.lang.NullPointerException
at com.crm.qa.TestCases.ContactPageTest.verifyContactsPageNewButtonTest(ContactPageTest.java:49)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
请确认您是否为所有页面仅使用一个驱动程序实例,基本为此发生空点异常,解决方案使用全局变量作为驱动程序实例
公共静态 Webdriver 驱动程序;
initializtion();
loginpage = new loginPage();
//below line is missing please add in HomePageTest class
**homepage=new Homepage();**
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.