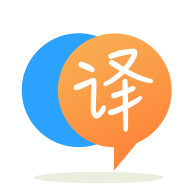
[英]Websocket - Error during WebSocket handshake: Unexpected response code: 404
[英]WebSocket EndPoint not getting resolved: Error Code: 404
我正在尝试创建一个非常基本的网络套接字应用程序。 步骤如下:
import javax.websocket.OnMessage;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/echo")
public class EchoServer {
@OnMessage
public String echo(String incomingMessage) {
return "I got this (" + incomingMessage + ") so I am sending it back";
}
}
使用 javascript 调用上述端点创建 html
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Web Socket JavaScript Echo Client</title> <script language="javascript" type="text/javascript"> var echo_websocket; function init() { output = document.getElementById("output"); } function send_echo() { var wsUri = "ws://localhost:7003/wspractice/echo"; writeToScreen("Connecting to " + wsUri); echo_websocket = new WebSocket(wsUri); echo_websocket.onopen = function (evt) { writeToScreen("Connected !"); doSend(textID.value); }; echo_websocket.onmessage = function (evt) { writeToScreen("Received message: " + evt.data); echo_websocket.close(); }; echo_websocket.onerror = function (evt) { writeToScreen('<span style="color: red;">ERROR:</span> ' + evt.data); echo_websocket.close(); }; } function doSend(message) { echo_websocket.send(message); writeToScreen("Sent message: " + message); } function writeToScreen(message) { var pre = document.createElement("p"); pre.style.wordWrap = "break-word"; pre.innerHTML = message; output.appendChild(pre); } window.addEventListener("load", init, false); </script> </head> <body> <h1>Echo Server</h1> <div style="text-align: left;"> <form action=""> <input onclick="send_echo()" value="Press to send" type="button"> <input id="textID" name="message" value="Hello Web Sockets" type="text"> <br> </form> </div> <div id="output"></div> </body> </html>
在 WebLogic Server 版本:14.1.1.0.0 服务器中将上述项目部署为 war 文件(上下文路径:wspractice)。
weblogic 上的命名服务器配置(侦听端口 7003)
部署在命名服务器上的应用程序
尝试上述 html 时,出现“WebSocket connection to 'ws://localhost:7003/wspractice/echo' failed: Error during WebSocket handshake: Unexpected response code: 404”错误。
当我尝试在 WEB-INF 中访问另一个普通的 jsp 时,它得到了正确的解析,但只有 web-socket 没有得到解析。 结果如下图所示。
我理解 404 发生在服务器无法找到服务(在这种情况下是回声)时,但我不明白为什么会在这种情况下发生,即使“回声”端点可用。
结果在浏览器中。
在 chrome、firefox 中尝试过,但结果相同。 请帮助我理解为什么会发生这种情况。
我试图对您的代码进行尽可能少的更改。 将其用作参考。
import java.io.IOException;
import javax.websocket.*;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint(value = "/echo")
public class EchoServer {
@OnMessage
public void onMessage(final Session session, String msg) {
System.out.println("Msg: " + msg);
try {
session.getBasicRemote().sendText(msg);
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
<html>
<head>
<meta charset="UTF-8">
<title>Web Socket JavaScript Echo Client</title>
<script language="javascript" type="text/javascript">
var echo_websocket;
function init() {
output = document.getElementById("output");
}
function send_echo() {
var wsUri = "ws://localhost:8080/test1/echo";
writeToScreen("Connecting to " + wsUri);
echo_websocket = new WebSocket(wsUri);
echo_websocket.onopen = function (evt) {
writeToScreen("Connected !");
doSend(document.getElementById("textID").value);
};
echo_websocket.onmessage = function (evt) {
writeToScreen("Received message: " + evt.data);
echo_websocket.close();
};
echo_websocket.onerror = function (evt) {
writeToScreen('<span style="color: red;">ERROR:</span> ' + evt);
console.log(evt)
echo_websocket.close();
};
}
function doSend(message) {
echo_websocket.send(message);
writeToScreen("Sent message: " + message);
}
function writeToScreen(message) {
var pre = document.createElement("p");
pre.style.wordWrap = "break-word";
pre.innerHTML = message;
output.appendChild(pre);
}
window.addEventListener("load", init, false);
</script>
</head>
<body>
<div style="text-align: left;">
<form action="">
<input onclick="send_echo()" value="Press to send" type="button">
<input id="textID" name="message" value="Hello Web Sockets" type="text">
<br>
</form>
</div>
<div id="output"></div>
</body>
</html>
mvn package embedded-glassfish:run
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.smirnov</groupId>
<artifactId>test1</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<name>test1</name>
<properties>
<endorsed.dir>${project.build.directory}/endorsed</endorsed.dir>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-web-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.7</source>
<target>1.7</target>
<compilerArguments>
<endorseddirs>${endorsed.dir}</endorseddirs>
</compilerArguments>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.3</version>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>2.6</version>
<executions>
<execution>
<phase>validate</phase>
<goals>
<goal>copy</goal>
</goals>
<configuration>
<outputDirectory>${endorsed.dir}</outputDirectory>
<silent>true</silent>
<artifactItems>
<artifactItem>
<groupId>javax</groupId>
<artifactId>javaee-endorsed-api</artifactId>
<version>7.0</version>
<type>jar</type>
</artifactItem>
</artifactItems>
</configuration>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.glassfish.embedded</groupId>
<artifactId>maven-embedded-glassfish-plugin</artifactId>
<version>4.0</version>
<configuration>
<goalPrefix>embedded-glassfish</goalPrefix>
<app>${basedir}/target/${project.artifactId}-${project.version}.war</app>
<autoDelete>true</autoDelete>
<port>8080</port>
<name>test1</name>
<contextRoot>test1</contextRoot>
</configuration>
<executions>
<execution>
<goals>
<goal>deploy</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
你可以在这里看到这个小项目。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.