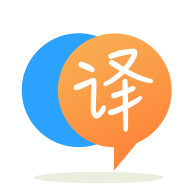
[英]Apply increment/decrement count on adding/removing divs with React Hooks
[英]React increment and decrement count
import {
ProductCycleContextProvider,
ProductContext,
} from '../contexts/productCycleContext';
class Cart extends Component {
static contextType = ProductContext;
constructor(props) {
super(props);
this.state = {
selectedForCart: [],
checkOut: true,
grandTotal: undefined,
};
}
async componentDidMount() {
await axios
.get('http://localhost:80/newItem/storeIntoCart')
.then((res) => {
res.data.map((i) => {
this.context.setCart(i);
});
})
.catch((e) => alert(e));
}
remove = () => {
console.log('remove');
};
increment = (v, i) => {
axios
.patch(`http://localhost:80/cart/cartCustomize/${i}`, {
quantity: v,
})
.then((res) => {
const ind = this.context.cart.findIndex((x) => x._id === res.data._id);
this.context.cart[ind] = res.data;
})
.catch((e) => console.log(e));
};
decrement = (v, i) => {};
render() {
return (
<div>
{this.context.cart &&
this.context.cart.map((v, i) => {
return (
<Container className="mt-5" key={i}>
<Row>
<Col lg={2} md={2} sm={12}>
<h5>
<b>{v.title}</b>
</h5>
<img
src={v.photo}
style={{
border: '2px solid black',
borderRadius: '5px',
width: '100px',
height: '80px',
}}
/>
</Col>
<Col lg={2} md={2} sm={12}>
<p>
Price: ₹
{v.priceTaxIncluded}
</p>
<p style={{fontSize: '10px'}}>Tax inclusive</p>
</Col>
<Col lg={2} md={2} sm={12}>
<Row>
<Button
value={v.quantity}
onClick={(e) => {
const val = e.target.value;
const id = v._id;
this.decrement(val, id);
}}>
-
</Button>
{v.quantity}
<Button
value={v.quantity}
onClick={(e) => {
const id = v._id;
let val = Number(e.target.value);
let q = val + 1;
this.increment(q, id);
}}>
+
</Button>
</Row>
</Col>
<Col lg={2} md={2} sm={12}>
<br></br>
<Button
className="btn btn-warning mt-2"
size="sm"
onClick={this.remove}>
Remove
</Button>
</Col>
<Col lg={2} md={2} sm={12}>
{this.state.checkOut && (
<>
<p>{v.priceTaxIncluded * v.quantity}</p>
</>
)}
</Col>
<Col lg={2} md={2} sm={12}></Col>
</Row>
</Container>
);
})}
</div>
);
}
}
在此代码中,单击增量时,我需要将产品数量增加一个。 这个数量应该乘以价格。 它正在发生但是如果我第一次增加那么它不会显示任何东西并且在第二次它增加并显示之前的值并且在第三次点击它显示第二次点击的值......就像它一样。 .. 如果我使用组件进行更新,我应该单击增量按钮 2 次...但是第一次渲染它?
如果手动更新ProductContext
,则不应更新购物车属性。 而是在ProductContext
中使用 function,它使用setState
通知 React 关于 state 的更改。
increment = (v, i) => {
axios
.patch(`http://localhost:80/cart/cartCustomize/${i}`, {
quantity: v,
})
.then((res) => {
this.context.setCartItem(res.data);
})
.catch((e) => console.log(e));
};
在您的提供者/上下文中:
setCartItem(cartItem) {
const ind = this.state.cart.findIndex((x) => x._id === cartItem._id);
const updatedCart = [...this.state.cart][ind] = cartItem;
this.setState(updatedCart);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.