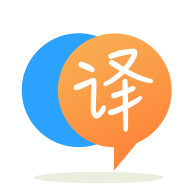
[英]INSERT INTO multiple rows from a dynamic html table to MYsql using PHP
[英]Dynamic table in HTML using MySQL and php
I've seen many posts about how to build a table in HTML with PHP and Mysql, but my problem is, that I often change the headers of MySQL columns. 有什么方法可以让 PHP 自动更新代码,这样我就可以输入表格名称并打印表格,而无需输入所有标签?
<?php
$table = "user";
$database = "database";
$conn = mysqli_connect("localhost", "username", "password", "database", "3306");
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM $table";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "<tr><td>" . $row["id"] . "</td><td>" . $row["first_name"] . "</td><td>" . $row["last_name"] . "</td><td>" . $row["birthday"] . "</td></tr>";
}
echo "</table>";
} else {
echo "0 result";
}
$conn->close();
如果您想将数据库表的全部内容显示为 HTML 表,我建议您创建一个函数,为您动态完成所有这些操作。 此函数应检查表是否存在,获取所有数据,并获取带有标题的输出 HTML 表。
这是我使用 MySQLi 的建议。 首先,您必须确保该表确实存在。 然后您可以从表中获取所有数据。 mysqli::query()
返回的对象将包含有关列名的所有元数据信息,您可以使用这些信息显示标题行。 您可以使用fetch_fields()
迭代每个列元数据。 可以使用fetch_all()
方法获取数据。
<?php
// create global connection using mysqli
mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT);
$conn = mysqli_connect("localhost", "username", "password", "database", "3306");
$mysqli->set_charset('utf8mb4'); // always set the charset
function outputMySQLToHTMLTable(mysqli $mysqli, string $table)
{
// Make sure that the table exists in the current database!
$tableNames = array_column($mysqli->query('SHOW TABLES')->fetch_all(), 0);
if (!in_array($table, $tableNames, true)) {
throw new UnexpectedValueException('Unknown table name provided!');
}
$res = $mysqli->query('SELECT * FROM '.$table);
$data = $res->fetch_all(MYSQLI_ASSOC);
echo '<table>';
// Display table header
echo '<thead>';
echo '<tr>';
foreach ($res->fetch_fields() as $column) {
echo '<th>'.htmlspecialchars($column->name).'</th>';
}
echo '</tr>';
echo '</thead>';
// If there is data then display each row
if ($data) {
foreach ($data as $row) {
echo '<tr>';
foreach ($row as $cell) {
echo '<td>'.htmlspecialchars($cell).'</td>';
}
echo '</tr>';
}
} else {
echo '<tr><td colspan="'.$res->field_count.'">No records in the table!</td></tr>';
}
echo '</table>';
}
outputMySQLToHTMLTable($mysqli, 'user');
使用 PDO 非常相似,但您必须注意 API 的差异。
要获取表名,您可以使用fetchAll(PDO::FETCH_COLUMN)
而不是array_column()
。 要获取列元数据,您需要使用getColumnMeta()
函数。
<?php
$pdo = new PDO("mysql:host=localhost;dbname=test;charset=utf8mb4", 'username', 'password', [
\PDO::ATTR_ERRMODE => \PDO::ERRMODE_EXCEPTION,
\PDO::ATTR_EMULATE_PREPARES => false
]);
function outputMySQLToHTMLTable(pdo $pdo, string $table)
{
// Make sure that the table exists in the current database!
$tableNames = $pdo->query('SHOW TABLES')->fetchAll(PDO::FETCH_COLUMN);
if (!in_array($table, $tableNames, true)) {
throw new UnexpectedValueException('Unknown table name provided!');
}
$stmt = $pdo->query('SELECT * FROM '.$table);
$data = $stmt->fetchAll(PDO::FETCH_ASSOC);
$columnCount = $stmt->columnCount();
echo '<table>';
// Display table header
echo '<thead>';
echo '<tr>';
for ($i = 0; $i < $columnCount; $i++) {
echo '<th>'.htmlspecialchars($stmt->getColumnMeta($i)['name']).'</th>';
}
echo '</tr>';
echo '</thead>';
// If there is data then display each row
if ($data) {
foreach ($data as $row) {
echo '<tr>';
foreach ($row as $cell) {
echo '<td>'.htmlspecialchars($cell).'</td>';
}
echo '</tr>';
}
} else {
echo '<tr><td colspan="'.$columnCount.'">No records in the table!</td></tr>';
}
echo '</table>';
}
outputMySQLToHTMLTable($pdo, 'user');
PS可以使用以下代码优化表存在性检查:
$tableNames = $pdo->prepare('SELECT COUNT(1) FROM information_schema.TABLES WHERE TABLE_SCHEMA = SCHEMA() AND TABLE_NAME=?');
$tableNames->execute([$table]);
if (!$tableNames->fetchColumn()) {
throw new UnexpectedValueException('Unknown table name provided!');
}
我刚刚阅读了您的问题,并进行了编码以满足您的需求,但是我使用了 PDO 而不是 MYSQLI,也许您最好理解代码,因为使用 PDO 一切都更简单。 而我做的方式,你不需要把所有的标签,PHP会自动更新,并且知道文件必须以扩展名.php结尾!
<?php
$conn = new PDO("mysql:host=localhost;dbname=YOUR_DATABASE_HERE", "USERNAME", "PASSWORD");
if(!$conn){
echo "Could not connect!";
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
table, th, td {
border: 1px solid black;
}
</style>
</head>
<body>
<table style="width:100%;">
<thead>
<tr>
<th>ID</th>
<th>FirstName</th>
<th>LastName</th>
<th>Birthday</th>
</tr>
</thead>
<tbody>
<?php
$sql = "SELECT * FROM user";
$result = $conn->prepare($sql);
$result->execute();
if($result->rowCount() > 0):
$rows = $result->fetchAll();
foreach($rows as $row):
?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['first_name']; ?></td>
<td><?php echo $row['last_name']; ?></td>
<td><?php echo $row['birthday']; ?></td>
</tr>
</tbody>
<?php
endforeach;
endif;
?>
</table>
</body>
</html>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.