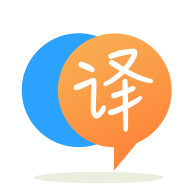
[英]How do I iterate through a array of object which is inside an object as a list in v-for
[英]How do I Iterate through an array inside an object?
<p v-for="quest in exam.questions" :key="quest">{{quest.text}}</p>
data() {
return {
message: "QUESTION DIPLAYS HERE",
name: "Ayoola",
exam: [{
id: 1,
name: "",
ref: "",
duration: 24000,
type: "cbt",
format: {
type: "random",
total: 20,
},
description: "In this exam do not cheat",
questions: [
{
id: 1,
text: "who is this"
},
"options": [
{
"code": "A",
"text": "Man was created in God's image",
"is_correct": true,
},
{
"code": "B",
"text": "Man was created to look like angels",
},
{
"code": "C",
"text": "Man created himself",
},
{
"code": "D",
"text": "Man was not created and cannot be destroyed",
},
]
]
}],
您在询问对象,但在您的示例中,您有包含父对象和子对象的数组。 如果exam
需要是一个数组,那么解决方案是:
v-for="quest in exam[0].questions"
你可以这样迭代。 (例如,在 COMPUTED 上,您可以在将来应用过滤器)
<template>
<ul>
<li v-for="quest in test_data" :key="quest.id">
{{quest.id}} <br>
{{quest.text}}
</li>
</ul>
</template>
<script>
export default {
data() {
return{
exam:
{ questions: [
{ id:1,
text:"who is this",
options:[
{
"code": "A",
"text": "Man was created in God's image",
"is_correct": true,
},
{
"code": "B",
"text": "Man was created to look like angels",
}
]
}
] }
}
},
computed: {
test_data() {
return this.exam.questions;
}
},
}
</script>
首先,您的数据对象not valid
(检查您的代码)。
"message": "Uncaught SyntaxError: Unexpected token ':'",
/* Throw error: "message": "Uncaught SyntaxError: Unexpected token ':'", */ var error = [ { name: "valid" }, name: "not valid" ] /* correct code */ var valid = [ { name: "valid" }, { name: "valid two" } ]
接下来,您没有正确抛出嵌套对象(在使用 v-for 之前尝试手动打印一项)。
exam.questions[0].text
(不正确)。
应该是: exam.questions[0].options[0].text
最好的办法是遵循这个基本的“Hello World”示例并编辑您的代码:
new Vue({ el: "#app", data(){ return{ msg: "world", exam: { name: "Exam name", questions: [ { id: 10, title: 'The capital of France?', "options": [ { "code": "A", "text": "Paris", "is_correct": true, }, { "code": "B", "text": "Lyon", } ] }, { id: 11, title: 'The capital of Italy?', "options": [ { "code": "A", "text": "Verona", "is_correct": true, }, { "code": "B", "text": "Roma", "is_correct": true } ] }, ] } } } })
<main id="app"> <ul> <li v-for="question in exam.questions" :key="question.id"> <h3>{{ question.title }}</h3> <ul> <li v-for="option in question.options" :key="option.code"> {{ option.text }} </li> </ul> </li> </ul> </main> <script src="https://cdnjs.cloudflare.com/ajax/libs/vue/2.6.11/vue.min.js"></script>
相关文档: https ://v2.vuejs.org/v2/guide/list.html
首先,您需要指定要循环通过的考试,或者如果您想要有多个考试元素,您可以在表单上方创建一个 v-for 为了简单地遍历数组,您可以在问题循环中放置一个 for 循环并遍历每个选项并打印出来
这是我实现它的方式:
<form>
<div v-for="quest in exam[0].questions" :key="quest.id">
<p>{{ quest.text }}</p>
<label v-for="(option, index) in quest.options" :key="index" :for="'answer'+quest.id+index">
<input type="radio" :name="'answer'+quest.id" :id="'answer'+quest.id+index">
{{ option.text }}
</label>
</div>
</form>
data() {
return {
message: "QUESTION DIPLAYS HERE",
name: "Ayoola",
exam: [
{
id: 1,
name: "",
ref: "",
duration: 24000,
type: "cbt",
format: {
type: "random",
total: 20
},
description: "In this exam do not cheat",
questions: [
{
id: 1,
text: "who is this",
options: [
{
code: "A",
text: "Man was created in God's image",
is_correct: true
},
{
code: "B",
text: "Man was created to look like angels"
},
{
code: "C",
text: "Man created himself"
},
{
code: "D",
text:
"Man was not created and cannot be destroyed"
}
]
},
{
id: 2,
text: "who is this",
options: [
{
code: "A",
text: "Man was created in God's image",
is_correct: true
},
{
code: "B",
text: "Man was created to look like angels"
},
{
code: "C",
text: "Man created himself"
},
{
code: "D",
text:
"Man was not created and cannot be destroyed"
}
]
}
]
}
]
};
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.