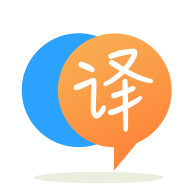
[英]How can I remove all HTML tags in a string except for the only 's' tag?
[英]Given a string, how can I use JavaScript to remove all 'HTML tags' except a specific 'tag' (and its 'children')?
给定一个类似于 HTML (但实际上不是 HTML)的字符串,我如何使用 JavaScript 删除除特定“标签”(及其“子项”)之外的所有“HTML 标签”?
例如,如果我有以下字符串:
'<p><span>Sample data: <math><msqrt><mo>y</mo></msqrt></math></span> <div><strong>hello world</strong><math><msqrt><mo>x</mo></msqrt></math></div></p>'
而且我只想保留原始文本和“数学标签”(以及每个“数学标签”中的所有内容),我该怎么做?
const html = '<p><span>Sample data: <math><msqrt><mo>y</mo></msqrt></math></span> <div><strong>hello world</strong><math><msqrt><mo>x</mo></msqrt></math></div></p>';
const result = stripNonSpecifiedHTML(html, 'math');
// expected result:
// 'Sample data: <math><msqrt><mo>y</mo></msqrt></math>hello world<math><msqrt><mo>x</mo></msqrt></math>'
function stripNonSpecifiedHTML(html, tagNameToKeep) {
// ...
}
你可以做一些类似的事情:
html.split('<math>')[1].split('</math>')[0]
它看起来很糟糕,但它确实有效(有一些限制):
<math>
和</math>
分割字符串<math>
和</math>
const html = '<p><span>Initial data: <math><msqrt><mo>y</mo></msqrt></math></span> <div><strong>hello world</strong><math><msqrt><mo>x</mo></msqrt></math></div></p>' var text = html.split('<math>') .map(t => t.split('</math>')).flat() .map((t, i) => {return (i % 2==0 ) ? t.replace(/<.+?>/g,''): t }) .map((t, i) => {return (i % 2==0 ) ? t : '<math>' + t + '</math>' }) .join(''); console.log(text); // OUTPUT: Initial data: <math><msqrt><mo>y</mo></msqrt></math> hello world<math><msqrt><mo>x</mo></msqrt></math>
虽然不是html
,但可以将此字符串解析为xml
。
const html = '<p><span>Initial data: <math><msqrt><mo>y</mo></msqrt></math></span> <div><math><msqrt><mo>x</mo></msqrt></math></div></p>';
let parser = new DOMParser(),
xmlDoc = parser.parseFromString(html, 'text/xml');
然后,您可以循环遍历所有<math>
标签。
let mathTags = xmlDoc.getElementsByTagName('math');
Array.from(mathTags).forEach(math => {
let data = math.innerHTML,
content = math.textContent;
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.