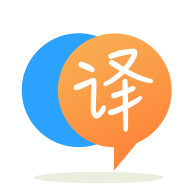
[英]Insert date into SQL Server database via TableAdapter vb.net
[英]Date wise data retrieval from SQL Server to Excel using VB.NET form
我想创建一个报告软件,在该软件中,通过用户表单中的 datepicker 工具在用户指定的日期检索日期。 我想知道如何实现代码以获得结果。
这是我的代码...请帮助我。 我是新手
Imports System.Data
Imports System.Data.SqlClient
Public Class Form1
Private conn As New SqlConnection
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Try
conn.ConnectionString = "Data Source=ROG\SQLEXPRESS;Initial Catalog=GKEAPL;Integrated Security=True;"
conn.Open()
MsgBox("Connected")
Catch ex As Exception
MsgBox("Could Not connect")
End Try
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim officeexcel As New Microsoft.Office.Interop.Excel.Application
officeexcel = CreateObject("Excel.Application")
Dim workbook As Object = officeexcel.Workbooks.Add("D:\GKEAPL\project 1\gk format.xltx")
officeexcel.Visible = True
Dim da As New SqlDataAdapter
Dim ds As New DataSet
da = New SqlDataAdapter("SELECT FeedWaterTankLevelFWST101,
FeedFlowFT101,
ClearWaterTankLevelCWST201,
TMFilPressurePT201,
TMFolPressurePT202,
HPPilPressurePT203,
MembraneilPressurePT204,
MembraneolPressurePT205,
PermeateFlowFT201,
RejectFlowFT202
FROM DATA1 WHERE(DATEnTIME >='2020-12-18 11:06:30.000' AND DATEnTIME <= '2020-12-19 10:07:31.000')", conn)
da.Fill(ds, "DATA1")
For i As Integer = 0 To ds.Tables("DATA1").Rows.Count - 1
With officeexcel
.Range("Sheet2!B" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(0).ToString
.Range("Sheet2!C" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(1).ToString
.Range("Sheet2!D" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(2).ToString
.Range("Sheet2!E" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(3).ToString
.Range("Sheet1!F" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(4).ToString
.Range("Sheet1!G" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(5).ToString
.Range("Sheet1!H" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(6).ToString
.Range("Sheet1!I" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(7).ToString
.Range("Sheet1!J" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(8).ToString
.Range("Sheet1!K" + (i + 7).ToString).Value = ds.Tables("DATA1").Rows(i).Item(9).ToString
End With
Next
officeexcel = Nothing
workbook = Nothing
Catch ex As Exception
End Try
End Sub
Private Sub Report_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
DateTimePicker1.CustomFormat = "YYYY-MMMM-DD"
End Sub
End Class
不要在Form.Load
打开连接。 连接是宝贵的资源。 将它们保留在使用它们的方法的本地。 在.Execute...
行之前打开并尽快关闭。
如果您查看 MS 文档https://docs.microsoft.com/en-us/dotnet/api/system.data.sqlclient.sqlconnection?view=dotnet-plat-ext-3.1中的SQLConnection
类,您将看到一个.Dispose
方法。 当你看到它时,这意味着应该调用它来释放类使用的非托管资源。 幸运的是,vb.net 提供了Using...End Using
块来为我们处理这个问题。
我将您的数据访问代码移到了单独的函数中。 一个方法应该尽可能只做一件事。
在您的 sql 字符串 WHERE 子句中,我将您的文字更改为参数并使用了 BETWEEN。
我认为您的 Excel 代码也有问题,但您的问题是关于数据访问。 简而言之,不要将工作簿变暗为对象,范围不是Excel.Application
的成员,并始终Excel.Application
Option Strict 。
Private Function GetTankData() As DataTable
Dim dt As New DataTable
Dim strSql = "SELECT FeedWaterTankLevelFWST101,
FeedFlowFT101,
ClearWaterTankLevelCWST201,
TMFilPressurePT201,
TMFolPressurePT202,
HPPilPressurePT203,
MembraneilPressurePT204,
MembraneolPressurePT205,
PermeateFlowFT201,
RejectFlowFT202
FROM DATA1 WHERE DATEnTIME BETWEEN @StartDate AND @EndDate"
Using conn As New SqlConnection("Data Source=ROG\SQLEXPRESS;Initial Catalog=GKEAPL;Integrated Security=True;"),
cmd As New SqlCommand(strSql, conn)
cmd.Parameters.Add("@StartDate", SqlDbType.DateTime).Value = DateTimePicker1.Value
cmd.Parameters.Add("@EndDate", SqlDbType.DateTime).Value = DateTimePicker2.Value
conn.Open()
dt.Load(cmd.ExecuteReader)
End Using 'Closes and disposes the connection and command
Return dt
End Function
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim officeexcel As New Microsoft.Office.Interop.Excel.Application
officeexcel = CreateObject("Excel.Application")
Dim workbook As Object = officeexcel.Workbooks.Add("D:\GKEAPL\project 1\gk format.xltx")
Try
officeexcel.Visible = True
Dim dt = GetTankData()
For i As Integer = 0 To dt.Rows.Count - 1
With officeexcel
.Range("Sheet2!B" + (i + 7).ToString).Value = dt.Rows(i).Item(0).ToString
.Range("Sheet2!C" + (i + 7).ToString).Value = dt.Rows(i).Item(1).ToString
.Range("Sheet2!D" + (i + 7).ToString).Value = dt.Rows(i).Item(2).ToString
.Range("Sheet2!E" + (i + 7).ToString).Value = dt.Rows(i).Item(3).ToString
.Range("Sheet1!F" + (i + 7).ToString).Value = dt.Rows(i).Item(4).ToString
.Range("Sheet1!G" + (i + 7).ToString).Value = dt.Rows(i).Item(5).ToString
.Range("Sheet1!H" + (i + 7).ToString).Value = dt.Rows(i).Item(6).ToString
.Range("Sheet1!I" + (i + 7).ToString).Value = dt.Rows(i).Item(7).ToString
.Range("Sheet1!J" + (i + 7).ToString).Value = dt.Rows(i).Item(8).ToString
.Range("Sheet1!K" + (i + 7).ToString).Value = dt.Rows(i).Item(9).ToString
End With
Next
Finally
officeexcel = Nothing
workbook = Nothing
End Try
End Sub
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.