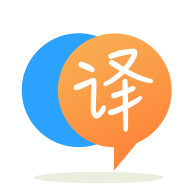
[英]Access TextField value from another composable function in Jetpack Compose
[英]Jetpack Compose: Launch ActivityResultContract request from Composable function
从androidx.activity:activity-ktx
的1.2.0-beta01 androidx.activity:activity-ktx
,不能再launch
使用Activity.registerForActivityResult()
创建的请求,如上面“行为更改”下的链接中突出显示的那样,并在此处的Google 问题中看到。
应用程序现在应该如何通过@Composable
函数启动这个请求? 以前,应用程序可以通过使用Ambient
将MainActivity
的实例向下传递链,然后轻松启动请求。
例如,可以通过以下方式解决新行为:在 Activity 的onCreate
函数之外实例化后,将注册活动结果的类传递到链中,然后在Composable
启动请求。 但是,无法通过这种方式注册完成后要执行的回调。
可以通过创建自定义ActivityResultContract
来解决这个问题,该自定义ActivityResultContract
在启动时接受回调。 但是,这意味着几乎所有内置的ActivityResultContracts
都不能与 Jetpack Compose 一起使用。
TL; 博士
应用程序如何从@Composable
函数启动ActivityResultsContract
请求?
从androidx.activity:activity-compose:1.3.0-alpha06
, registerForActivityResult()
API 已重命名为rememberLauncherForActivityResult()
以更好地指示返回的ActivityResultLauncher
是代表您记住的托管对象。
val result = remember { mutableStateOf<Bitmap?>(null) }
val launcher = rememberLauncherForActivityResult(ActivityResultContracts.TakePicturePreview()) {
result.value = it
}
Button(onClick = { launcher.launch() }) {
Text(text = "Take a picture")
}
result.value?.let { image ->
Image(image.asImageBitmap(), null, modifier = Modifier.fillMaxWidth())
}
Activity Result 有两个 API 界面:
ActivityResultRegistry
。 这才是真正的底层工作。ComponentActivity
和Fragment
实现的ActivityResultCaller
中的便捷接口,将 Activity Result 请求与 Activity 或 Fragment 的生命周期联系起来 Composable 具有与 Activity 或 Fragment 不同的生命周期(例如,如果您从层次结构中删除 Composable,它应该在其自身之后进行清理),因此使用ActivityResultCaller
API(例如registerForActivityResult()
永远不是正确的做法。
相反,您应该直接使用ActivityResultRegistry
API,直接调用register()
和unregister()
。 这最好与rememberUpdatedState()
和DisposableEffect
搭配使用,以创建一个可与 Composable 一起使用的registerForActivityResult
版本:
@Composable
fun <I, O> registerForActivityResult(
contract: ActivityResultContract<I, O>,
onResult: (O) -> Unit
) : ActivityResultLauncher<I> {
// First, find the ActivityResultRegistry by casting the Context
// (which is actually a ComponentActivity) to ActivityResultRegistryOwner
val owner = ContextAmbient.current as ActivityResultRegistryOwner
val activityResultRegistry = owner.activityResultRegistry
// Keep track of the current onResult listener
val currentOnResult = rememberUpdatedState(onResult)
// It doesn't really matter what the key is, just that it is unique
// and consistent across configuration changes
val key = rememberSavedInstanceState { UUID.randomUUID().toString() }
// Since we don't have a reference to the real ActivityResultLauncher
// until we register(), we build a layer of indirection so we can
// immediately return an ActivityResultLauncher
// (this is the same approach that Fragment.registerForActivityResult uses)
val realLauncher = mutableStateOf<ActivityResultLauncher<I>?>(null)
val returnedLauncher = remember {
object : ActivityResultLauncher<I>() {
override fun launch(input: I, options: ActivityOptionsCompat?) {
realLauncher.value?.launch(input, options)
}
override fun unregister() {
realLauncher.value?.unregister()
}
override fun getContract() = contract
}
}
// DisposableEffect ensures that we only register once
// and that we unregister when the composable is disposed
DisposableEffect(activityResultRegistry, key, contract) {
realLauncher.value = activityResultRegistry.register(key, contract) {
currentOnResult.value(it)
}
onDispose {
realLauncher.value?.unregister()
}
}
return returnedLauncher
}
然后可以通过代码在您自己的 Composable 中使用它,例如:
val result = remember { mutableStateOf<Bitmap?>(null) }
val launcher = registerForActivityResult(ActivityResultContracts.TakePicturePreview()) {
// Here we just update the state, but you could imagine
// pre-processing the result, or updating a MutableSharedFlow that
// your composable collects
result.value = it
}
// Now your onClick listener can call launch()
Button(onClick = { launcher.launch() } ) {
Text(text = "Take a picture")
}
// And you can use the result once it becomes available
result.value?.let { image ->
Image(image.asImageAsset(),
modifier = Modifier.fillMaxWidth())
}
从Activity Compose 1.3.0-alpha03
及更高版本开始,有一个新的实用函数registerForActivityResult()
可以简化此过程。
@Composable
fun RegisterForActivityResult() {
val result = remember { mutableStateOf<Bitmap?>(null) }
val launcher = registerForActivityResult(ActivityResultContracts.TakePicturePreview()) {
result.value = it
}
Button(onClick = { launcher.launch() }) {
Text(text = "Take a picture")
}
result.value?.let { image ->
Image(image.asImageBitmap(), null, modifier = Modifier.fillMaxWidth())
}
}
(来自此处给出的示例)
对于那些没有通过@ianhanniballake 提供的要点返回结果的人,在我的情况下, returnedLauncher
实际上捕获了realLauncher
的已经处置的值。
因此,虽然删除间接层应该可以解决问题,但这绝对不是执行此操作的最佳方法。
这是更新的版本,直到找到更好的解决方案:
@Composable
fun <I, O> registerForActivityResult(
contract: ActivityResultContract<I, O>,
onResult: (O) -> Unit
): ActivityResultLauncher<I> {
// First, find the ActivityResultRegistry by casting the Context
// (which is actually a ComponentActivity) to ActivityResultRegistryOwner
val owner = AmbientContext.current as ActivityResultRegistryOwner
val activityResultRegistry = owner.activityResultRegistry
// Keep track of the current onResult listener
val currentOnResult = rememberUpdatedState(onResult)
// It doesn't really matter what the key is, just that it is unique
// and consistent across configuration changes
val key = rememberSavedInstanceState { UUID.randomUUID().toString() }
// TODO a working layer of indirection would be great
val realLauncher = remember<ActivityResultLauncher<I>> {
activityResultRegistry.register(key, contract) {
currentOnResult.value(it)
}
}
onDispose {
realLauncher.unregister()
}
return realLauncher
}
添加以防万一有人开始新的外部意图。 就我而言,我想在单击 jetpack compose 中的按钮时启动谷歌登录提示。
宣布你的意图发射
val startForResult =
rememberLauncherForActivityResult(ActivityResultContracts.StartActivityForResult()) { result: ActivityResult ->
if (result.resultCode == Activity.RESULT_OK) {
val intent = result.data
//do something here
}
}
启动您的新活动或任何意图。
Button(
onClick = {
//important step
startForResult.launch(googleSignInClient?.signInIntent)
},
modifier = Modifier
.fillMaxWidth()
.padding(start = 16.dp, end = 16.dp),
shape = RoundedCornerShape(6.dp),
colors = ButtonDefaults.buttonColors(
backgroundColor = Color.Black,
contentColor = Color.White
)
) {
Image(
painter = painterResource(id = R.drawable.ic_logo_google),
contentDescription = ""
)
Text(text = "Sign in with Google", modifier = Modifier.padding(6.dp))
}
#googlesignin
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.