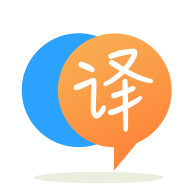
[英]Google oauth java client to get an access token fails with “400 Bad Request { ”error“ : ”invalid_request“ }”
[英]Google Calendar API Java 400 invalid_request
@Component
public class GoogleCalendarSyncListener implements ApplicationListener<CourseLessonGoogleCalendarNeedToBeUpdatedEvent> {
private String getEventId(Lesson parameter) { // encapsulator method
return "cita" + parameter.getId();
}
// TODO bu daha genel olabilir.
@Configuration
static class Configurator {
@Bean
public Calendar client() {
List<String> scopes = Lists.newArrayList(CalendarScopes.CALENDAR);
try {
Calendar client = CalendarUtils.createCalendarClient(scopes);
return client;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
private final Logger logger = LoggerFactory.getLogger(getClass());
@Autowired
private LessonService lessonService;
@Autowired
private LessonUserService lessonUserService;
@Autowired
private Calendar client; // bunu da confige taşımak lazım
@Override
public void onApplicationEvent(CourseLessonGoogleCalendarNeedToBeUpdatedEvent courseLessonGoogleCalendarNeedToBeUpdatedEvent) {
try {
ProductType productType = courseLessonGoogleCalendarNeedToBeUpdatedEvent.getCourseRegistration().getCourse().getCategory().getProductType();
Course course = courseLessonGoogleCalendarNeedToBeUpdatedEvent.getCourseRegistration().getCourse();
List<Lesson> lessons = lessonService.loadLessonsByCourse(course);
int lessonCount = lessons.size();
for (Lesson lesson : lessons) {
String description = "";
try {
String eventId = getEventId(lesson); // RFC2938 bu kurala uygun olmalı
DateTime start = new DateTime(lesson.getDate(), TimeZone.getTimeZone("UTC+03:00"));
java.util.Calendar cal = java.util.Calendar.getInstance();
cal.setTime(lesson.getDate());
cal.add(java.util.Calendar.MINUTE, lesson.getLessonPricingTemplate().getDurationMinutes());
cal.getTime();
DateTime end = new DateTime(cal.getTime(), TimeZone.getTimeZone("UTC+03:00"));
description += lesson.getCourse().getCatName();
String color = "";
for (LessonUser lessonuser : lessonUserService.loadByLesson(lesson)) {
description += " " + lessonuser.getUser().getPrettyName();
switch (lessonuser.getUser().getUsername()) {
case "bgor":
color = Constants.BGORCOLOR;
break;
}
}
// TODO error checdking
description += lessonService.lessonIndex(lesson) + "/" + lessonCount;
Event event = new Event();
event.setColorId(color);
event.setId(eventId);
event.setReminders(new Event.Reminders().setUseDefault(false));
event.setSummary(description);
event.setStart(new EventDateTime().setDateTime(start));
event.setEnd(new EventDateTime().setDateTime(end));
event.setDescription(lesson.getCourse().getCatName());
List<EventAttendee> attendees = new ArrayList<>();
EventAttendee admin = new EventAttendee();
admin.setEmail("mail@btmuzik.com");
attendees.add(admin);
List<LessonUser> lessonUsers = lessonUserService.loadByLesson(lesson);
lessonUsers.forEach(lu -> {
EventAttendee attendee = new EventAttendee();
if (lu.getUser().getEmail() != null) {
attendee.setEmail(lu.getUser().getEmail());
attendees.add(attendee);
}
});
event.setAttendees(attendees);
Event.Creator creator = new Event.Creator();
Event.Organizer organizer = new Event.Organizer();
creator.setEmail("mail@btmuzik.com");
organizer.setEmail("mail@btmuzik.com");
event.setCreator(creator);
event.setOrganizer(organizer);
logger.info("event %s (%s)\n", event.getSummary(), start);
saveOrUpdateEvent(event);
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
}
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
}
// By existing conf tomcat/.store/bt-calendar-sync/StoredCredentials must exist
// These are copy of btmuzikevi-parent/tokens/StoredCredential created by CalendarReader
private Event saveOrUpdateEvent(Event event) {
try {
System.out.println("client = " + client.getBaseUrl());
System.out.println("client = " + client.getRootUrl());
System.out.println("event.getId() = " + event.getId());
List<String> scopes = Lists.newArrayList(CalendarScopes.CALENDAR);
client = CalendarUtils.createCalendarClient(scopes);
Calendar.Events.List request = client.events().list("primary");
System.out.println("--------------------------------- ");
event = client.events().update("primary", event.getId(), event).execute(); // updated event
System.out.println(event.getId()+ " HUHA = " + event.getStart());
return event;
} catch (GoogleJsonResponseException e) { // burası özel bir değer veriyor
try {
event = client.events().insert("primary", event).execute();
return event;
} catch (IOException ioException) { // bu çok özel değil ama tüm detayı içeriyor
throw new RuntimeException(ioException); // tamamını runtimeexception olarak dönüyoruz.
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
CalendarQuickstart 或 CalendarReader 很好,它们从日历中读取但是: 当我尝试写入日历时,我得到:
授权错误错误 400:invalid_request 缺少必需的参数:response_type
我错过了什么? 感谢您提前提供帮助
如果您收到该消息,那么您对库进行身份验证的方式存在重大问题。 我所知道的关于 Java 与谷歌 api java 客户端库的最佳教程是用于谷歌分析的
我已尝试将其翻译成日历,它应该是关闭的。
/**
* A simple example of how to access the Google Calendar API.
*/
public class HelloCalendar {
// Path to client_secrets.json file downloaded from the Developer's Console.
// The path is relative to HelloAnalytics.java.
private static final String CLIENT_SECRET_JSON_RESOURCE = "client_secrets.json";
// Replace with your view ID.
private static final String VIEW_ID = "<REPLACE_WITH_VIEW_ID>";
// The directory where the user's credentials will be stored.
private static final File DATA_STORE_DIR = new File(
System.getProperty("user.home"), ".store/hello_calendar");
private static final String APPLICATION_NAME = "Hello Calendar";
private static final JsonFactory JSON_FACTORY = GsonFactory.getDefaultInstance();
private static NetHttpTransport httpTransport;
private static FileDataStoreFactory dataStoreFactory;
public static void main(String[] args) {
try {
Calendar service = initializeCalendarReporting();
// make call here
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Initializes an authorized Calendar service object.
*
* @return The Calendar service object.
* @throws IOException
* @throws GeneralSecurityException
*/
private static Calendar initializeCalendar() throws GeneralSecurityException, IOException {
httpTransport = GoogleNetHttpTransport.newTrustedTransport();
dataStoreFactory = new FileDataStoreFactory(DATA_STORE_DIR);
// Load client secrets.
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY,
new InputStreamReader(HelloCalendar.class
.getResourceAsStream(CLIENT_SECRET_JSON_RESOURCE)));
// Set up authorization code flow for all authorization scopes.
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow
.Builder(httpTransport, JSON_FACTORY, clientSecrets,
CalendarScopes.all()).setDataStoreFactory(dataStoreFactory)
.build();
// Authorize.
Credential credential = new AuthorizationCodeInstalledApp(flow,
new LocalServerReceiver()).authorize("user");
// Construct the Calendar service object.
return new Calendar.Builder(httpTransport, JSON_FACTORY, credential)
.setApplicationName(APPLICATION_NAME).build();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.