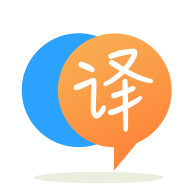
[英]How do I write a wrapper function with templated function parameters which can take overloaded member functions?
[英]How to define a function, parameters of which take variables from main() function?
我遇到需要定义一个function,它的参数从main()function中取arguments。代码如下:
#include <iostream>
using namespace std;
void unravel(char arr[][column], int field[][column], int x, int y) { //revealing adjoined cells
for (int minusrows = -1; minusrows < 2; minusrows++){
for (int minuscolumns = -1; minuscolumns < 2; minuscolumns++){
arr[x + minusrows][y + minuscolumns] = field[x + minusrows][y + minuscolumns] + '0';
}
}
}
int main (){
int row, column;
cin >> row >> column;
char a[row][column];
int field[row][column];
for (int i = 0; i < row; i++){ //filling a with asterisks, field with integers
for (int j = 0; j < column; j++){
a[i][j] = '*';
field[i][j] = i + j;
}
}
int x = 2, y = 3;
unravel(a, field, x, y);
for (int i = 0; i < 5; i++){ //printing out the array a
for (int j = 0; j < 5; j++){
cout << a[i][j] << " ";
}
cout << endl;
}
return 0;
}
这是我得到的错误:
main.cpp:4:25: error: ‘column’ was not declared in this scope
void unravel(char arr[][column], int field[][column], int x, int y) {
^~~~~~
main.cpp:4:32: error: expected ‘)’ before ‘,’ token
void unravel(char arr[][column], int field[][column], int x, int y) {
^
main.cpp:4:34: error: expected unqualified-id before ‘int’
void unravel(char arr[][column], int field[][column], int x, int y) {
^~~
因此,目标是将最初由星号 (*) 组成的二维数组 a[ ][ ] 中单元格周围的值更改为具有相同索引的 int 二维数组字段 [ ][ ] 的值。 为此负责 function unravel () 。 当我使用 integer 5 而不是row和column时,代码运行良好。
#include <iostream>
using namespace std;
void unravel(char arr[][5], int field[][5], int x, int y) { //revealing adjoined cells
for (int minusrows = -1; minusrows < 2; minusrows++){
for (int minuscolumns = -1; minuscolumns < 2; minuscolumns++){
arr[x + minusrows][y + minuscolumns] = field[x + minusrows][y + minuscolumns] + '0';
}
}
}
int main (){
char a[5][5];
int field[5][5];
for (int i = 0; i < 5; i++){ //filling a with asterisks, field with integers
for (int j = 0; j < 5; j++){
a[i][j] = '*';
field[i][j] = i + j;
}
}
int x = 2, y = 3;
unravel(a, field, x, y);
for (int i = 0; i < 5; i++){ //printing out the array a
for (int j = 0; j < 5; j++){
cout << a[i][j] << " ";
}
cout << endl;
}
return 0;
}
因此问题是:是否可以从 main() function 中存储一些数据,以便 function unravel ()能够像我预期的那样工作? 我知道变量是在 scope 中定义的。我看到的关于我得到的错误的任何其他内容都与我的问题没有太大关系。 对不起,如果帖子看起来很长。 提前致谢。
main()
中的这些行:
int row, column;
cin >> row >> column;
char a[row][column];
int field[row][column];
导致尝试使用运行时值声明数组。 这无效 C++ 。
C++ 中的 Arrays 的大小必须由编译时表达式表示,而不是运行时值。
最简单的解决方案是使用std::vector
。 使用typedef
或using
可简化此操作:
#include <vector>
using Char1D = std::vector<char>;
using Char2D = std::vector<Char1D>;
using Int1D = std::vector<int>;
using Int2D = std::vector<Int1D>;
int main()
{
int row, column;
cin >> row >> column;
Char2D a(row, Char1D(column,'*'));
Int2D field(row, Int1D(column));
for (int i = 0; i < row; i++)
{
for (int j = 0; j < column; j++)
field[i][j] = i + j;
}
//...
int x = 2, y = 3;
unravel(a, field, x, y);
}
然后unravel
function,当使用这些定义时,也变得更容易处理:
void unravel(Char2D& arr, Int2D& field, int x, int y)
{
//...
}
请注意,我们传递引用,模仿您实际使用 2D arrays 时的行为。
基本上其他一切都保持不变。
动态二维数组可以像这样声明为指向数组的指针数组
int** a = new int*[rowCount];
for(int i = 0; i < rowCount; ++i)
a[i] = new int[colCount];
有关详细信息,请参阅: How do I declare a 2d array in C++ using new?
因此,如果您使用 new 关键字声明二维数组,您的代码将正常工作。
#include <iostream>
using namespace std;
void unravel(char **arr, int **field, int x, int y) { //revealing adjoined cells
for (int minusrows = -1; minusrows < 2; minusrows++){
for (int minuscolumns = -1; minuscolumns < 2; minuscolumns++){
arr[x + minusrows][y + minuscolumns] = field[x + minusrows][y + minuscolumns] + '0';
}
}
}
int main (){
int row, column;
cin >> row >> column;
char **a = new char*[row];
int **field = new int*[row];
for (int i = 0; i < row; i++){ //filling a with asterisks, field with integers
a[i]=new char[column];
field[i] = new int[column];
for (int j = 0; j < column; j++){
a[i][j] = '*';
field[i][j] = i + j;
}
}
int x = 2, y = 3;
unravel(a, field, x, y);
for (int i = 0; i < row; i++){ //printing out the array a
for (int j = 0; j < column; j++){
cout << a[i][j] << " ";
}
cout << endl;
}
for(int i=0; i<row; i++){
delete [] a[i];
delete [] field[i];
}
delete [] a;
delete [] field;
return 0;
}
请注意:memory 是使用 new 关键字在堆上分配的。 为了防止 memory 泄漏,我们应该在最后免费分配 memory。 在这个程序中可以这样做。
for(int i=0; i<row; i++){
delete [] a[i];
delete [] field[i];
}
delete [] a;
delete [] field;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.