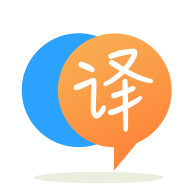
[英]System.NullReferenceException for .NET service running on windows server IIS but works locally when running in Visual Studio IIS Express
[英]Getting NullReferenceException on IIS Express while running from Visual Studio 2019
我正在尝试对来自数据库(托管在远程服务器上的 Oracle18c)中的表进行 CRUD 操作 - “项目”,其中包含项目和单位重量作为列。 这是我的第一个 ASP.NET 项目。 只需按照我在网上找到的教程( https://www.c-sharpcorner.com/blogs/crud-operation-in-asp-net-core30-with-oracle-database2 )。 我能够毫无问题地按照步骤操作。 但是当我尝试在 IIS Express 中运行该项目时,我在图片中出现以下错误。 我不确定我做错了什么。
我在下面给出我的片段。
Items.cs [模型]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace cs550.Models
{
public class Items
{
public string item { get; set; }
public int unitWeight { get; set; }
}
}
itemService.CS [服务]
using cs550.Interface;
using cs550.Models;
using Microsoft.Extensions.Configuration;
using Oracle.ManagedDataAccess.Client;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace cs550.Services
{
public class itemService : IItemService
{
private readonly string _connectionString;
public itemService(IConfiguration _configuration)
{
_connectionString = _configuration.GetConnectionString("OracleDBConnection");
}
public IEnumerable<Items> getAllItem()
{
List<Items> itemList = new List<Items>();
using (OracleConnection con = new OracleConnection(_connectionString))
{
using (OracleCommand cmd = con.CreateCommand())
{
con.Open();
cmd.BindByName = true;
cmd.CommandText = "Select item, unitWeight from Items";
OracleDataReader rdr = cmd.ExecuteReader();
while(rdr.Read())
{
Items item = new Items
{
item = rdr["item"].ToString(),
unitWeight = Convert.ToInt32(rdr["unitWeight"])
};
itemList.Add(item);
}
}
}
return itemList;
}
public Items getItemByItem(string it)
{
Items items = new Items();
using (OracleConnection con = new OracleConnection(_connectionString))
{
using (OracleCommand cmd = con.CreateCommand())
{
con.Open();
cmd.BindByName = true;
cmd.CommandText = "Select item, unitWeight from Items Where item="+it+"";
OracleDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
items.item = rdr["item"].ToString();
items.unitWeight = Convert.ToInt32(rdr["unitWeight"]);
}
}
return items;
}
}
public void AddItems(Items it)
{
try
{
using (OracleConnection con = new OracleConnection(_connectionString))
{
using (OracleCommand cmd = con.CreateCommand())
{
con.Open();
cmd.CommandText = "Insert into Items(item, unitWeight) Values('"+it.item+"',"+it.unitWeight+")";
cmd.ExecuteNonQuery();
}
}
}
catch (Exception)
{
throw;
}
}
public void EditItems(Items it)
{
try
{
using (OracleConnection con = new OracleConnection(_connectionString))
{
using (OracleCommand cmd = con.CreateCommand())
{
con.Open();
cmd.CommandText = "Update Items Set unitWeight="+it.unitWeight+" where item='"+it.item+"'";
cmd.ExecuteNonQuery();
}
}
}
catch (Exception)
{
throw;
}
}
}
}
IItemService.cs [接口]
using cs550.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace cs550.Interface
{
public interface IItemService
{
IEnumerable<Items> getAllItem();
Items getItemByItem(string it);
void AddItems(Items it);
public void EditItems(Items it);
}
}
结构体
using cs550.Interface;
using cs550.Services;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace cs550
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<IItemService, itemService>();
services.AddSingleton<IConfiguration>(Configuration);
services.AddMvc().AddRazorPagesOptions(options =>
{
options.Conventions.AddPageRoute("/Items/Index", "");
});
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Items}/{action=Index}/{item?}");
});
}
}
}
appsetting.JSON
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"ConnectionStrings": {
"OracleDBConnection": "User Id=myUserID;Password=myPassword; Data Source= remoteURL:1521/DB"
},
"AllowedHosts": "*"
}
项目控制器.cs
using cs550.Interface;
using cs550.Models;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace cs550.Controllers
{
public class ItemsController : Controller
{
IItemService itemService;
public ItemsController(IItemService _itemService)
{
itemService = _itemService;
}
public ActionResult Index()
{
IEnumerable<Items> items = itemService.getAllItem();
return View();
}
public ActionResult Create()
{
return View();
}
[HttpPost]
public ActionResult Create(Items it)
{
itemService.AddItems(it);
return RedirectToAction(nameof(Index));
}
public ActionResult Edit(string item)
{
Items it = itemService.getItemByItem(item);
return View(it);
}
[HttpPost]
public ActionResult Edit(Items it)
{
itemService.EditItems(it);
return RedirectToAction(nameof(Index));
}
}
}
我的剃刀页面:
索引.cshtml
@model IEnumerable<cs550.Models.Items> @{ ViewData["Title"] = "Index"; } <h1>Index</h1> <p> <a asp-action="Create">Create New</a> </p> <table class="table"> <thead> <tr> <th> @Html.DisplayNameFor(model => model.item) </th> <th> @Html.DisplayNameFor(model => model.unitWeight) </th> <th></th> </tr> </thead> <tbody> @foreach (var item in Model) { <tr> <td> @Html.DisplayFor(modelItem => item.item) </td> <td> @Html.DisplayFor(modelItem => item.unitWeight) </td> <td> @Html.ActionLink("Edit", "Edit", new { id=item }) | @Html.ActionLink("Delete", "Delete", new { id=item }) </td> </tr> } </tbody> </table>
创建.cshtml
@model cs550.Models.Items @{ ViewData["Title"] = "Create"; } <h1>Create</h1> <h4>Items</h4> <hr /> <div class="row"> <div class="col-md-4"> <form asp-action="Create"> <div asp-validation-summary="ModelOnly" class="text-danger"></div> <div class="form-group"> <label asp-for="item" class="control-label"></label> <input asp-for="item" class="form-control" /> <span asp-validation-for="item" class="text-danger"></span> </div> <div class="form-group"> <label asp-for="unitWeight" class="control-label"></label> <input asp-for="unitWeight" class="form-control" /> <span asp-validation-for="unitWeight" class="text-danger"></span> </div> <div class="form-group"> <input type="submit" value="Create" class="btn btn-primary" /> </div> </form> </div> </div> <div> <a asp-action="Index">Back to List</a> </div> @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} }
编辑.cshtml
@model cs550.Models.Items @{ ViewData["Title"] = "Edit"; } <h1>Edit</h1> <h4>Items</h4> <hr /> <div class="row"> <div class="col-md-4"> <form asp-action="Edit"> <div asp-validation-summary="ModelOnly" class="text-danger"></div> <div class="form-group"> <label asp-for="item" class="control-label"></label> <input asp-for="item" class="form-control" /> <span asp-validation-for="item" class="text-danger"></span> </div> <div class="form-group"> <label asp-for="unitWeight" class="control-label"></label> <input asp-for="unitWeight" class="form-control" /> <span asp-validation-for="unitWeight" class="text-danger"></span> </div> <div class="form-group"> <input type="submit" value="Save" class="btn btn-primary" /> </div> </form> </div> </div> <div> <a asp-action="Index">Back to List</a> </div> @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} }
Change:
using (OracleConnection con = new OracleConnection(_connectionString))
{
using (OracleCommand cmd = new OracleCommand())
{
到:
using(OracleConnection con = new OracleConnection(_connectionString))
{
using(OracleCommand cmd = con.CreateCommand())
{
您必须在数据阅读器中使用 PL/SQL 文本,或者您可以创建一个存储过程并使用引用游标来处理数据
也改变:
public class itemController : Controller
到
public class ItemsController : Controller
更改您的操作索引代码:
public ActionResult Index()
{
IEnumerable<Items> items = itemService.getAllItem();
return View(items);
}
Error 404 Not Found
是一个客户端错误,表明Error 404 Not Found
您正在搜索的资源。 这里您要搜索的资源是Index
in items
。
在Startup.cs
您提到的路线为{controller=Items}/{action=Index}/{item?}
。 因此,在加载时,它将搜索名为Items
的控制器,然后将执行Index
操作。 这里控制器名称不同,它是item
,因此无法找到Index
操作。 因此,将其更改为正确的控制器名称为public class ItemsController : Controller
将解决404
问题。
如果您想在路由中使用它,请将 http 动词(Post、Get、Put、Delete)添加到操作中。 这里一些像Edit
这样的方法缺少这些动词。
还要注意的另一件事是,您没有添加任何剃刀页面,因此我希望您将直接在浏览器中调用路由或使用邮递员/提琴手之类的工具。 因此,当您启动 IISExpress 时,它将转到映射到默认路由的localhost:<port number>
。 因此,如果您想访问任何特定操作,请更改调用方法,例如 Get 或 Post,并添加适当的路由路径。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.