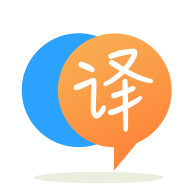
[英]I can't access the initial state of my useState hook inside of my method to change the state
[英]Why cant I take a value from the current state and use that inside of my useState hook?
目前,登录用户的 object 设置为当前 state,从下面的 doe 中您可以看到我是从 authContext 引入的。 我正在尝试获取 user.firstName 值并将其设置为 useState 挂钩内的作者。 当我在我的应用程序中运行它时,我查看存储日志 object 但作者值为空白的数据库。 我对编码很陌生,所以任何建议都将不胜感激!
import React, { useState, useContext } from "react";
import M from "materialize-css/dist/js/materialize.min.js";
import LogContext from "../context/logs/logContext";
import AuthContext from "../context/auth/authContext";
const AddLogModal = () => {
const logContext = useContext(LogContext);
const authContext = useContext(AuthContext);
const { user } = authContext;
const { addLog } = logContext;
const [log, setLog] = useState({
title: "",
description: "",
attention: "",
author: user.firstName,
date: new Date(),
});
const { title, description, attention, author } = log;
const onChange = (e) => setLog({ ...log, [e.target.name]: e.target.value });
const onSubmit = (e) => {
e.preventDefault();
if (title === "" || description === "" || attention === "") {
M.toast({ html: "Please enter a title and description.." });
} else {
setLog({ ...log, [e.target.name]: e.target.value });
}
addLog(log);
setLog({
title: "",
description: "",
attention: "",
author: user.firstName,
});
};
return (
<form id="add-log-modal" className="modal" style={modalStyle}>
<div className="modal-content">
<h4>Open New Issue</h4>
<div className="row">
<div className="input-field">
<input type="text" name="title" value={title} onChange={onChange} />
<label htmlFor="message" className="active">
Log Title
</label>
</div>
</div>
<div className="input-field">
<input
type="text"
name="description"
value={description}
onChange={onChange}
/>
<label htmlFor="message" className="active">
Log Description
</label>
</div>
<div className="input-field">
<input type="text" name="author" value={author} onChange={onChange} />
<label htmlFor="message" className="active">
Created By:
</label>
</div>
<div className="row">
<div className="input-field">
<p>
<label>
<input
type="radio"
name="attention"
checked={attention === "Needs Attention"}
value="Needs Attention"
onChange={onChange}
/>
<span>Needs Attention</span>
</label>
</p>
<p>
<label>
<input
type="radio"
name="attention"
checked={attention === "Issue Resolved"}
value="Issue Resolved"
onChange={onChange}
/>
<span>Issue Resolved</span>
</label>
</p>
</div>
</div>
</div>
<div className="modal-footer">
<a
href="#!"
onClick={onSubmit}
className="modal-close waves-effect waves-light blue btn"
>
Enter
</a>
</div>
</form>
);
};
const modalStyle = {
width: "75%",
height: "75%",
};
export default AddLogModal;
授权上下文
import React, { useReducer } from "react";
import axios from "axios";
import AuthContext from "./authContext";
import setAuthToken from "../setAuthToken";
import authReducer from "./authReducer";
import {
REGISTER_SUCCESS,
REGISTER_FAIL,
LOGIN_FAIL,
LOGIN_SUCCESS,
LOGOUT,
USER_LOADED,
AUTH_ERROR,
CLEAR_ERRORS,
} from "../Types";
const AuthState = (props) => {
const initialState = {
token: localStorage.getItem("token"),
isAuthenticated: false,
loading: false,
user: {},
error: null,
};
const [state, dispatch] = useReducer(authReducer, initialState);
// Load User
const loadUser = async () => {
if (localStorage.token) {
setAuthToken(localStorage.token);
}
try {
const res = await axios.get("/api/auth");
dispatch({ type: USER_LOADED, payload: res.data });
} catch (err) {
dispatch({ type: AUTH_ERROR });
}
};
// Register User
const register = async (formData) => {
const config = {
headers: {
"Content-type": "application/json",
},
};
try {
const res = await axios.post("/api/users", formData, config);
dispatch({
type: REGISTER_SUCCESS,
payload: res.data,
});
loadUser();
} catch (err) {
dispatch({
type: REGISTER_FAIL,
payload: err.response.data.msg,
});
}
};
// Login User
const login = async (formData) => {
const config = {
headers: {
"Content-type": "application/json",
},
};
try {
const res = await axios.post("/api/auth", formData, config);
dispatch({
type: LOGIN_SUCCESS,
payload: res.data,
});
loadUser();
} catch (err) {
dispatch({
type: LOGIN_FAIL,
payload: err.response.data.msg,
});
}
};
// Logout
const logout = () => {
dispatch({
type: LOGOUT,
});
};
// Clear Errors
const clearErrors = () => {
dispatch({
type: CLEAR_ERRORS,
});
};
return (
<AuthContext.Provider
value={{
token: state.token,
isAuthenticated: state.isAuthenticated,
loading: state.loading,
user: state.user,
error: state.error,
register,
login,
clearErrors,
loadUser,
logout,
}}
>
{props.children}
</AuthContext.Provider>
);
};
export default AuthState;
反应文档:
如果使用以前的 state 计算新的 state,则可以将 function 传递给 setState。 function 将接收先前的值,并返回更新的值。 下面是一个计数器组件的示例,它同时使用了 setState 的 forms
反应示例:
<button onClick={() => setCount(prevCount => prevCount - 1)}>-</button>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.