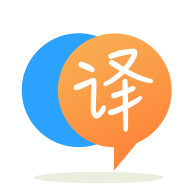
[英]Create a form with input controls dynamically from JSON using Angular 2
[英]How create dynamic form controls and fetch data from it in Angular
我对 Angular 很陌生,遇到了一个问题。
当组件加载时,我有两个输入字段作为终端 ID(文本字段)和终端类型(下拉)。 从下拉列表中选择值后,onChange 方法会调用 API 并为我获取数据。 数据格式如下:
data={
"productResults":[
{
"productId":"1",
"productName":"credit"
},
{
"productId":"2",
"productName":"debit"
}
],
"metaResultList":[
{
"customizationType":"time",
"customizationValue":"0420",
"longDescription":"Item1"
},
{
"customizationType":"code",
"customizationValue":"N",
"longDescription":"Auto Close",
"customizationCodeDetail":{
"customizationCodeDetails":[
{
"value":"N",
"shortDescription":"None"
},
{
"value":"H",
"shortDescription":"host Auto Close"
}
]
}
},
{
"customizationType":"bool",
"customizationValue":"Y",
"longDescription":"Block Account"
},
{
"customizationType":"bool",
"customizationValue":"N",
"longDescription":"Block Sales"
},
{
"customizationType":"number",
"customizationValue":"55421",
"longDescrption":"Max Value"
}
]
}
我所做的是当我从下拉列表中选择后获取数据时,我在这两个文件下面有两个部分,它们将与 ngIf 我获取数据一起显示:
第一个:产品部分- 我使用 ngFor 像这样迭代 data.productResults 并显示切换
<div *ngFor="let product of data.productResultResults">
<label>{{product.productName}}</label>
<ion-toggle></ion-toggle>
</div>
第 2 部分:其他部分:这里我遍历 metaResultList 以显示文本字段,如果自定义类型是时间或数字,如果自定义类型是代码,则下拉,如果自定义类型是布尔,则切换。
<div *ngFor="let other of data.metaResultList">
<div *ngIf="other.customizationType==='time'||other.customizationType==='number'">
<label>{{other.longDescription}}</label>
<input type="text" value="other.customizationValue"/>
</div>
<div *ngIf="other.customizationType==='bool'">
<label>{{other.longDescription}}</label>
<ion-toggle></ion-toggle>
</div>
<div *ngIf="other.customizationType==='code'">
<label>{{other.longDescription}}</label>
<dropdown [datalist]="autoCloseDropDown"></dropdown>
</div>
</div>
这个下拉菜单是我的自定义下拉菜单,我将值作为主机自动关闭的 H 和 N 的值传递,并且我在下拉菜单中获得选项(我在获得结果后执行了该逻辑)。
单击下面的提交按钮后,我希望我的数据采用这种格式
{
"tid":"3",
"terminalType":"gateway",
"products":[
{
"productId":"1",
"productname":"credit"
}
],
"customizations":[
{
"customizationName":"Item1",
"customizationValue":"0420"
},
{
"customizationName":"Block Account",
"customizationValue":"Y"
},
{
"customizationName":"Block Sales",
"customizationValue":"N"
},
{
"customizationName":"Max Value",
"customizationValue":"54556"
}
]
}
现在我的问题是如何制作表单控件,因为这些字段是在我在终端类型下拉列表中做出决定后生成的,但我已经在 ngOnInit 中制作了表单组。我应该如何使表单控件名称动态化。 我将如何仅在 products 数组中插入那些值为 Y 形式的值。 有什么方法可以在下拉列表中进行更改后实例化表单组?另外,有时我可能不会从 api 获得某些字段,例如 type=bool,所以我不会在 FrontEnd 中显示
任何帮助,将不胜感激。
如果要通过 object 或数组生成动态 formControl,可以使用下面的方法:
public builder(data: any): FormGroup | FormArray {
if (Array.isArray(data)) {
const formGroup = [];
for (const d of data) {
formGroup.push(this.builder(d));
}
return this.formBuilder.array(formGroup);
} else {
const formGroup = {};
Object.keys(data).forEach((key) => {
if (typeof data[key] === 'object' && data[key] && !Array.isArray(data[key])) {
Object.assign(formGroup, {
[key]: this.builder(data[key]),
});
} else if (Array.isArray(data[key])) {
Object.assign(formGroup, {
[key]: this.formBuilder.array([]),
});
data[key].forEach((newData: any) => {
formGroup[key].push(this.builder(newData));
});
} else {
Object.assign(formGroup, { [key]: [data[key]] });
}
});
return this.formBuilder.group(formGroup);
}
}
现在,如果您想生成 object 或数组动态,您可以使用参数数据作为 object 或数组调用该方法。 例如:
ngOnInit(): void {
// will be generate a form array generatedForm in formGroup
const dynamicArray = [{ id: 1, name: 'John Doe'}, { id: 2, name: 'Jane Doe'}];
this.formGroup = this.formBuilder.group({
generatedForm: this.builder(dynamicArray)
});
// look at the form array
console.log(this.formGroup.get('generatedForm'));
// will be generated a formGroup by an object
const dynamicObject = { id: 1, name: 'John Doe'};
this.formGroup = <FormGroup>this.builder(dynamicObject)
// dynamic formGroup by an object
console.log(this.formGroup);
}
例如:您可以查看: https://stackblitz.com/edit/angular-ivy-7fm4d1
好的,现在您可以尝试使用 that's builder。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.