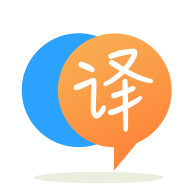
[英]Problem in a program to convert upper case string to lowercase using function in C
[英]C program to convert to upper case using fork
我需要创建一个具有子进程和父进程的程序。 子进程必须将父进程发送的行转换为大写,父进程必须将行发送给子进程进行转换,并通过标准输入显示转换后的行。 我已经有了这个,但是当我在终端上执行时,大写行没有显示。
任何建议
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/wait.h>
#include <string.h>
#include <ctype.h>
int main(void) {
int p1[2];
int p2[2];
pid_t pid;
char buffer[1024];
FILE *fp1;
FILE *fp2;
pipe(p1);
pipe(p2);
pid = fork();
if (pid < 0) {
fprintf(stderr, "Error fork\n");
exit(1);
}
if (pid == 0) {
// Close pipes entrances that aren't used
close(p1[1]);
close(p2[0]);
// Open file descriptors in used entrances
fp1 = fdopen(p1[0], "r");
fp2 = fdopen(p2[1], "w");
// Read from the corresponding file descriptor (pipe extreme)
while (fgets(buffer, 1024, fp1) != NULL) {
for (int i = 0; i < strlen(buffer); i++) {
buffer[i] = toupper(buffer[i]);
}
fputs(buffer, fp2);
}
// Once finished, close the reaming pipes entrances
fclose(fp1);
fclose(fp2);
exit(1);
}
// Close unused pipes entrances
close(p1[0]);
close(p2[1]);
// Open dile descriptors
fp1 = fdopen(p1[1], "w");
fp2 = fdopen(p2[0], "r");
while (fgets(buffer, 1024, stdin) != NULL) {
fputs(buffer, fp1); // Send buffer to write line pipe
fgets(buffer, 1024, fp2); // Get buffer readed from read line pipe
printf("%s", buffer); // Print in stdout the buffer
}
// Once finished, close the reaming pipes entrances
fclose(fp1);
fclose(fp2);
// Wait fork
wait(NULL);
return 0;
}
When using a FILE *
stream and the C library stream API, it's important to keep in mind that I/O operations can be "buffered". 在大多数情况下,默认情况下,当通过fputs(...)
执行写入时,字节实际上不会被发送到底层文件 object(在这种情况下是 pipe 结束),直到缓冲区被刷新。 在上面的代码中,您可以在两次调用fputs(...)
之后添加对fflush(fpN)
的调用(其中 N 与代码中的数字匹配)。 这应该有助于解决您的问题。
请注意,另外还有一些方法可以手动更改给定文件 stream 的缓冲模式。 此信息可以在man setbuf
中找到。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.