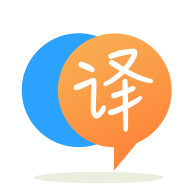
[英]Using java Runtime (on a windows machine) to send a linux command to a linux server
[英]How to send file from linux server to windows server using Java?
我在使用 Java 将文件从 Linux 服务器发送到 windows 服务器时遇到问题。 我使用 jsch 库来发送文件。 当我从 linux 服务器对 windows 服务器的 990 端口进行远程登录时,一切正常。 这是它的外观:
我写了这段代码来发送文件:
protected boolean sendFileToRemoteServer() {
String fileName = redExpressConfigurationProperties.getUploadPath() + "test.txt";
JSch jsch = new JSch();
Session jschSession = null;
boolean returnedValue = false;
try {
jschSession = getSessionOfRemoteServer(jsch);
log.debug("Got Session!");
ChannelSftp channelSftp = getSftpChannelFromSession(jschSession);
channelSftp.put(fileName, redExpressConfigurationProperties.getRemoteServerFilePath());
channelSftp.exit();
log.debug("File sent!");
returnedValue = true;
} catch (JSchException | SftpException e) {
log.error("Exception: ", e);
} finally {
if (jschSession != null) {
jschSession.disconnect();
}
}
return returnedValue;
}
protected Session getSessionOfRemoteServer(JSch jsch) throws JSchException {
Properties properties = new Properties();
properties.put("StrictHostKeyChecking", "no");
Session session = jsch.getSession(redExpressConfigurationProperties.getRemoteServerUser(),
redExpressConfigurationProperties.getRemoteServerAddress(),
redExpressConfigurationProperties.getRemoteServerSshPort()); // port is 990
session.setConfig(properties);
session.setPassword(redExpressConfigurationProperties.getRemoteServerPassword());
log.debug("password: {}", redExpressConfigurationProperties.getRemoteServerPassword());
log.debug("server address: {}", redExpressConfigurationProperties.getRemoteServerAddress());
return session;
}
protected ChannelSftp getSftpChannelFromSession(Session jschSession) throws JSchException {
jschSession.connect(redExpressConfigurationProperties.getRemoteSessionTimeout()); // timeout is 10000
log.debug("Server Connected!");
Channel channel = jschSession.openChannel("sftp");
channel.connect(redExpressConfigurationProperties.getRemoteChannelTimeout());
log.debug("Got sftp channel for session!");
return (ChannelSftp) channel;
}
我的 gradle 依赖是这样的:
implementation 'com.jcraft:jsch:0.1.55'
这是我的 application.properties 文件的一部分:
但是当我运行代码时,我在尝试连接 windows 服务器时遇到异常。 这是日志:
2020-12-21 17:48:07:976 [main] ERROR c.e.d.DemoApplication:78 - Exception:
com.jcraft.jsch.JSchException: Session.connect: java.net.SocketTimeoutException: Read timed out
at com.jcraft.jsch.Session.connect(Session.java:565)
at com.example.demo.DemoApplication.getSftpChannelFromSession(DemoApplication.java:55)
at com.example.demo.DemoApplication.sendFileToRemoteServer(DemoApplication.java:71)
at com.example.demo.DemoApplication.test(DemoApplication.java:31)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04.CGLIB$test$3(<generated>)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04$$FastClassBySpringCGLIB$$22565bf1.invoke(<generated>)
at org.springframework.cglib.proxy.MethodProxy.invokeSuper(MethodProxy.java:244)
at org.springframework.context.annotation.ConfigurationClassEnhancer$BeanMethodInterceptor.intercept(ConfigurationClassEnhancer.java:331)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04.test(<generated>)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.springframework.beans.factory.support.SimpleInstantiationStrategy.instantiate(SimpleInstantiationStrategy.java:154)
at org.springframework.beans.factory.support.ConstructorResolver.instantiate(ConstructorResolver.java:653)
at org.springframework.beans.factory.support.ConstructorResolver.instantiateUsingFactoryMethod(ConstructorResolver.java:486)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.instantiateUsingFactoryMethod(AbstractAutowireCapableBeanFactory.java:1336)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBeanInstance(AbstractAutowireCapableBeanFactory.java:1179)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:571)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:531)
at org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0(AbstractBeanFactory.java:335)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:234)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:333)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:208)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:944)
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:923)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:588)
at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:767)
at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:759)
at org.springframework.boot.SpringApplication.refreshContext(SpringApplication.java:426)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:326)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1309)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1298)
at com.example.demo.DemoApplication.main(DemoApplication.java:25)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.springframework.boot.loader.MainMethodRunner.run(MainMethodRunner.java:49)
at org.springframework.boot.loader.Launcher.launch(Launcher.java:107)
at org.springframework.boot.loader.Launcher.launch(Launcher.java:58)
at org.springframework.boot.loader.JarLauncher.main(JarLauncher.java:88)
这是我遇到异常的行:
jschSession.connect(redExpressConfigurationProperties.getRemoteSessionTimeout());
我做错了什么? 我是否选择了错误的图书馆来达到我的目的? 是否有任何身份验证问题?或任何其他问题?
我没有通过谷歌搜索找到太多帮助。 所以贴在这里。 能得到帮助会很棒。 提前致谢。
我在使用 Java 将文件从 Linux 服务器发送到 windows 服务器时遇到问题。 我使用 jsch 库来发送文件。 当我从 linux 服务器对 windows 服务器的 990 端口进行远程登录时,一切正常。 这是它的外观:
我写了这段代码来发送文件:
protected boolean sendFileToRemoteServer() {
String fileName = redExpressConfigurationProperties.getUploadPath() + "test.txt";
JSch jsch = new JSch();
Session jschSession = null;
boolean returnedValue = false;
try {
jschSession = getSessionOfRemoteServer(jsch);
log.debug("Got Session!");
ChannelSftp channelSftp = getSftpChannelFromSession(jschSession);
channelSftp.put(fileName, redExpressConfigurationProperties.getRemoteServerFilePath());
channelSftp.exit();
log.debug("File sent!");
returnedValue = true;
} catch (JSchException | SftpException e) {
log.error("Exception: ", e);
} finally {
if (jschSession != null) {
jschSession.disconnect();
}
}
return returnedValue;
}
protected Session getSessionOfRemoteServer(JSch jsch) throws JSchException {
Properties properties = new Properties();
properties.put("StrictHostKeyChecking", "no");
Session session = jsch.getSession(redExpressConfigurationProperties.getRemoteServerUser(),
redExpressConfigurationProperties.getRemoteServerAddress(),
redExpressConfigurationProperties.getRemoteServerSshPort()); // port is 990
session.setConfig(properties);
session.setPassword(redExpressConfigurationProperties.getRemoteServerPassword());
log.debug("password: {}", redExpressConfigurationProperties.getRemoteServerPassword());
log.debug("server address: {}", redExpressConfigurationProperties.getRemoteServerAddress());
return session;
}
protected ChannelSftp getSftpChannelFromSession(Session jschSession) throws JSchException {
jschSession.connect(redExpressConfigurationProperties.getRemoteSessionTimeout()); // timeout is 10000
log.debug("Server Connected!");
Channel channel = jschSession.openChannel("sftp");
channel.connect(redExpressConfigurationProperties.getRemoteChannelTimeout());
log.debug("Got sftp channel for session!");
return (ChannelSftp) channel;
}
我的 gradle 依赖是这样的:
implementation 'com.jcraft:jsch:0.1.55'
这是我的 application.properties 文件的一部分:
但是当我运行代码时,我在尝试连接 windows 服务器时遇到异常。 这是日志:
2020-12-21 17:48:07:976 [main] ERROR c.e.d.DemoApplication:78 - Exception:
com.jcraft.jsch.JSchException: Session.connect: java.net.SocketTimeoutException: Read timed out
at com.jcraft.jsch.Session.connect(Session.java:565)
at com.example.demo.DemoApplication.getSftpChannelFromSession(DemoApplication.java:55)
at com.example.demo.DemoApplication.sendFileToRemoteServer(DemoApplication.java:71)
at com.example.demo.DemoApplication.test(DemoApplication.java:31)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04.CGLIB$test$3(<generated>)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04$$FastClassBySpringCGLIB$$22565bf1.invoke(<generated>)
at org.springframework.cglib.proxy.MethodProxy.invokeSuper(MethodProxy.java:244)
at org.springframework.context.annotation.ConfigurationClassEnhancer$BeanMethodInterceptor.intercept(ConfigurationClassEnhancer.java:331)
at com.example.demo.DemoApplication$$EnhancerBySpringCGLIB$$a080bb04.test(<generated>)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.springframework.beans.factory.support.SimpleInstantiationStrategy.instantiate(SimpleInstantiationStrategy.java:154)
at org.springframework.beans.factory.support.ConstructorResolver.instantiate(ConstructorResolver.java:653)
at org.springframework.beans.factory.support.ConstructorResolver.instantiateUsingFactoryMethod(ConstructorResolver.java:486)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.instantiateUsingFactoryMethod(AbstractAutowireCapableBeanFactory.java:1336)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBeanInstance(AbstractAutowireCapableBeanFactory.java:1179)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:571)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:531)
at org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0(AbstractBeanFactory.java:335)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:234)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:333)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:208)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:944)
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:923)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:588)
at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:767)
at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:759)
at org.springframework.boot.SpringApplication.refreshContext(SpringApplication.java:426)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:326)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1309)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1298)
at com.example.demo.DemoApplication.main(DemoApplication.java:25)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.springframework.boot.loader.MainMethodRunner.run(MainMethodRunner.java:49)
at org.springframework.boot.loader.Launcher.launch(Launcher.java:107)
at org.springframework.boot.loader.Launcher.launch(Launcher.java:58)
at org.springframework.boot.loader.JarLauncher.main(JarLauncher.java:88)
这是我遇到异常的行:
jschSession.connect(redExpressConfigurationProperties.getRemoteSessionTimeout());
我做错了什么? 我是否选择了错误的图书馆来达到我的目的? 是否有任何身份验证问题?或任何其他问题?
我没有通过谷歌搜索找到太多帮助。 所以贴在这里。 能得到帮助会很棒。 提前致谢。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.