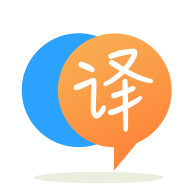
[英]Parent-Child model (multiple model) in one view (ASP.NET Core (C#) + MVC + Entity Framework)
[英]ASP.NET MVC Parent-Child with ViewModel and Entity Framework
这是我的代码。
家长:
public class Parent
{
[Key]
public int Id { get; set; }
[Required]
[MaxLength(50), MinLength(3)]
[Display(Name = "Parent Name")]
public string Name { get; set; }
[Required]
[MaxLength(50), MinLength(3)]
[Display(Name = "Parent Surname")]
public string Surname { get; set; }
public List<Child> Children { get; set; } = new List<Child>();
}
孩子:
public class Child
{
[Key]
public int Id { get; set; }
[Required]
[MaxLength(50), MinLength(3)]
[Display(Name = "Child Name")]
public string Name { get; set; }
[Required]
[MaxLength(50), MinLength(3)]
[Display(Name = "Child Surname")]
public string Surname { get; set; }
[Required]
[Display(Name = "Parent")]
public int ParentId { get; set; }
[ForeignKey("ParentId")]
public virtual Parent Parent { get; set; }
}
视图模型:
public class ParentChildViewModel
{
public Parent Parent { get; set; }
public IEnumerable<Child> Children { get; set; }
}
Controller:
public class DemoController : Controller
{
private readonly ApplicationDbContext _db;
public DemoController(ApplicationDbContext db)
{
_db = db;
}
public async Task<IActionResult> Index(int id)
{
var parent = await _db.Parents.AsNoTracking().FirstOrDefaultAsync(i => i.Id == id);
var child = await _db.Children.AsNoTracking().Where(i => i.ParentId == id).ToListAsync();
var model = new ParentChildViewModel
{
Parent = parent,
Children = child
};
return View(model);
}
}
看法:
@model TwoModels.Models.ParentChildViewModel
<h2> Demo Table Parent</h2>
<table style="border:solid 2px;">
<tr>
<td>
Parent Name:
</td>
<td>
@Html.DisplayFor(m => m.Parent.Name) <br />
</td>
</tr>
<tr>
<td>
Parent Surname:
</td>
<td>
@Html.DisplayFor(m => m.Parent.Surname) <br />
</td>
</tr>
</table>
<h2>Demo Table Child</h2>
<table style="border:solid 2px;">
@foreach (var item in Model.Children)
{
<tr>
<td>
Child Name:
</td>
<td>
@Html.DisplayFor(modelItem => item.Name) <br />
</td>
</tr>
<tr>
<td>
Child Surname:
</td>
<td>
@Html.DisplayFor(modelItem => item.Surname) <br />
</td>
</tr>
}
</table>
正如代码很容易理解的那样,我使用: _db.Parents.AsNoTracking().FirstOrDefaultAsync(i => i.Id == id)
作为父级(我不想要一个列表,而只想要一个列表)和: _db.Children.AsNoTracking().Where(i => i.ParentId == id).ToListAsync()
(这是一个列表)
但是以这种方式,对数据库的请求被发出了两次。 所以,我想修改代码,保持 ViewModel 的使用,但只访问一次数据库。 我想到了一件事:
_db.Parents.AsNoTracking().Include(c=>c.Children).Where(i => i.Id == id).ToListAsync();
如何更改我的 Controller 代码和视图?
您的操作可能是这样的:
public async Task<IActionResult> Index(int id)
{
var item = await _db.Parents.AsNoTracking().
.Include(c=>c.Children)
.Where(i => i.Id == id).
FirstOrDefaultAsync();
var model = new ParentChildViewModel
{
Parent = item,
Children = item.Children
};
item.Children=null; // only if you want to use view model alone.
return View(model);
}
并从 Children 属性中删除 new List()。 它应该是这样的:
public List<Child> Children { get; set; }
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.