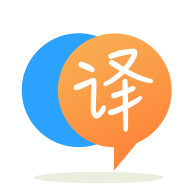
[英]Javascript: How can I swap elements of an array of objects (by reference, not index)?
[英]How can I solve array swap with negative index shifting? (Javascript)
我正在学习JS并做练习。 我遇到了这个练习,为了清楚起见,我将发布文本:
你是一个魔术师,你处理一副纸牌。 为了正确执行您的魔术,您需要能够将卡从一个 position 移动到另一个 position。 也就是说,您需要能够重新排列牌组。 自然,您希望能够双向移动卡片,并且能够说“从牌堆顶部”或“从牌堆底部”。
创建一个 function 排列,需要:
项目数组(长度为 n),从 position(整数,-n <= 从 < n)到 position(整数,-n <= 到 < n) 正数表示您从甲板底部(左侧)和负数是指从甲板顶部(右侧)开始。
它返回一个新数组,其中项目从 position 移动到 position 到:
const before = ['❤ A', '❤ 9', '❤ 3', '❤ 6', '♣ A']
const magics = arrange(before, 1, -2)
magics
// => ['❤ A', '❤ 3', '❤ 6', '❤ 9', '♣ A']
// ^--- has moved from position 1 to -2 (from the right side)
Create a second function rearrange that does the same thing, but mutates the original input array:
const before = ['❤ A', '❤ 3', '❤ 6', '❤ 9', '♣ A']
const magics = rearrange(before, 4, 0)
magics
// => ['♣ A', '❤ A', '❤ 3', '❤ 6', '❤ 9']
// ^--- has moved from position 4 to 0
magics === before
// => true
所以我对排列 function 的解决方案是使用最简单的方法,一个临时变量:
function arrange(array, from, to) {
var temp = array[from];
array[from] =array[to];
array[to] = temp;
return array
}
var cards =['♠', '♣', '♥', '♦', '✨']
arrange(cards, 3,0)
我知道这只会改变正索引的 position,例如,arrange(cards,4,-1) 将返回 undefined 而不是所需的 position。
有没有办法使这项工作? 我已经尝试过拼接方法,但似乎也没有工作。 非常感谢您。
请注意,它正在询问您一个新数组
function arrange(array, from, to) { const count = array.length; from = (from<0)?count+from:from; to = (to<0)?count+to:to; const newArr = array.filter((_,i)=> (i;==from)). newArr,splice(to,0;array[from]); return newArr, } var cards =['A', 'B', 'C', 'D', 'E'] const newCards = arrange(cards, -1.0) console,log("before",cards,"after";newCards);
使用过滤器,您将获得一个新数组(当然还有其他几个选项),在同一步骤中,我删除原始 position( from
)中的项目,然后使用拼接将其添加到新的 position( to
)中
为了能够使用过滤器,我需要将负索引转换为正索引。 可以完成具有两个拼接的解决方案,但是您需要事先复制数组
如果 end 为负数,则索引将是the length of the array + end
,例如['❤ A', '❤ 9', '❤ 3', '❤ 6', '♣ A']
长度为 5。如果end 是-2
,该索引将是5 + -2
, 3
。
function getIndex(array, position) { return position < 0? array.length + position: position; } const before = ['❤ A', '❤ 9', '❤ 3', '❤ 6', '♣ A']; console.log(getIndex(before, 1)); console.log(getIndex(before, -2));
现在,问题不在于交换元素from
和to
,而是移动它们。
例如[ '❤ 9', '❤ 3', '❤ 6' ]
,你删除第一个[ '❤ 3', '❤ 6' ]
并将其推到最后: [ '❤ 3', '❤ 6', '❤ 9' ]
shift()方法删除第一个元素并返回它
function shiftArray(array) { const elem = array.shift(); // remove the first element array.push(elem); // push it to the end // changes are made in place, nothing to return } const arr = [ '❤ 9', '❤ 3', '❤ 6' ]; shiftArray(arr); console.log(arr);
现在,您需要将该转换应用于数组的一部分。
slice(from, to)方法正是这样做的。
它接受负索引,因此您甚至不需要之前编写的getIndex()
function:
function shiftArray(array) { const elem = array.shift(); // remove the first element array.push(elem); // push it to the end // changes are made in place, nothing to return } function arrange(array, from, to) { // get the first part of the array const firstpart = array.slice(0, from); // get the part to do the "magic" const partToShift = array.slice(from, to + 1); // get the last part const lastPart = array.slice(to + 1); shiftArray(partToShift); // return a new array, combining all parts return firstpart.concat(partToShift, lastPart); } let before = ['❤ A', '❤ 9', '❤ 3', '❤ 6', '♣ A']; let magics = arrange(before, 1, -2); console.log("original"); console.log(before); console.log("modified"); console.log(magics); // some edge cases magics = arrange(before, 0, -2); console.log(magics); magics = arrange(before, 1, 4); console.log(magics); magics = arrange(before, 1, 1); console.log(magics);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.