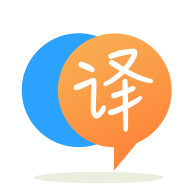
[英]How do I reference a variable in redux store from a component index.js (react.js)
[英]exporting a variable from index.js and importing it into another class - react.js
我正在尝试在另一个 class util.js 中使用我的索引中的变量。 在 index.js 的构造函数中,我将两个变量设置为 0,然后使用this.setState({ startTime: Date.now() })
和this.setState({ endTime: Date.now() })
。 我看到一个建议使用export const difference = true;
在我的 class 上方的 index.js 上,然后在 render() 之后,有const difference = this.state.endTime - this.state.startTime;
在返回()之前。 从那里我想将它导入到我的 util.js 中,这样我就可以将它放在我的数据库中。
实用程序.js:
import "isomorphic-fetch"
import { difference } from "../pages/index.js";
export function addName(name) {
return fetch('http://localhost:3001/addtime', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ name, time: difference }) // getting errors with this
})
}
应用程序.js
import React from 'react';
import { addName } from "./util";
function App() {
const [name, setName] = React.useState("")
function handleUpdate(evt) {
setName(evt.target.value);
}
async function handleAddName(evt) {
await addName(name);
}
return <div>
<p><input type='text' value={name} onChange={handleUpdate} /></p>
<button className='button-style' onClick={handleAddName}>Add Name</button>
</div>
}
export default App;
这样做会给我两个错误:UnhandledPromiseRejectionWarning 和 DeprecationWarning。 index.js:
export const difference = true;
export default class Home extends React.Component {
constructor(props) {
super(props);
this.state = {
startTime: 0,
endTime: 0,
};
}
handleStart = () => {
...
this.setState({
startTime: Date.now()
})
...
}
handleStop = () => {
...
this.setState({
endTime: Date.now()
})
...
}
render() {
const difference = this.state.endTime - this.state.startTime;
return (
...
)
}
}
我的主要问题是,如何从我在构造函数中设置的主要 class 导出变量,然后将其导入另一个 class,然后从那里将其放入数据库?
我认为您无法导出该变量。 最好将该变量作为道具传递或将其保存到 redux 中。
export
是顶级的,这意味着您不能从块内导出。 您也无法重新初始化const
,因此您阅读的建议不起作用。 您可以将其初始化为index.js
顶部的export let difference
,然后在您的渲染中 function, difference = this.state.endTime - this.state.startTime
但是以这种方式传递 class 组件 state 非常尴尬。 一个好的解决方案是考虑使用 Redux 或 React.Context。 这将允许您存储difference
,然后将其提取到您调用App.js
的addName
中。
虽然您尝试做的事情是可能的,但它会出现错误且难以维护。
在 React 中,所有数据都应该作为 props 传递。 这保证了组件将被正确更新并相应地重新渲染。 访问普通的共享变量将在渲染周期之外,并可能导致问题或意外结果。
在 react 中有两种方法可以做到这一点:道具传递和上下文。 上下文可能不是这里的正确工具。 上下文通常用于防止道具钻探(即,将道具从顶部组件向下传递到几个不同的组件)或者如果有多个具有特定值的消费者。 请参阅此处的注意事项。
在这种情况下,您可以相当简单地传递它,我建议将 function 传递给 StopWatch class (在您的示例中为 Home)
应用程序.js
import React from 'react';
import StopWatch from './StopWatch';
import { addName } from "./util";
function App() {
const [name, setName] = React.useState("")
const [duration, setDuration] = React.useState(0)
function handleUpdate(evt) {
setName(evt.target.value);
}
async function handleAddName(evt) {
await addName(name, duration);
}
return <div>
<StopWatch onStop={(d) => setDuration(d)} />
<p><input type='text' value={name} onChange={handleUpdate} /></p>
<button className='button-style' onClick={handleAddName}>Add Name</button>
</div>
}
export default App;
秒表.js
export default class StopWatch extends React.Component {
constructor(props) {
super(props);
this.state = {
startTime: 0,
};
}
handleStart = () => {
...
this.setState({
startTime: Date.now()
})
...
}
handleStop = () => {
const difference = Date.now() - this.state.startTime;
this.props.onStop(difference);
...
}
render() {
return (...)
}
}
注意:您还必须修改addName
function 以接受差异作为参数。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.