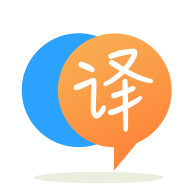
[英]HTML/CSS change color of buttons including other buttons when clicked
[英]How to make buttons change colors when clicked on (html, CSS, JavaScript)
我设置了 3 个按钮,想知道如何让它们在单击时更改 colors。 “是”应该是绿色的“否”应该是红色的“也许”也应该是红色的。
<!DOCTYPE html>
<html lang="en">
<head>
<link
href="https://fonts.googleapis.com/css2?family=Montserrat:wght@500&display=swap"
rel="stylesheet">
<link href="styles.css" rel="stylesheet">
<title>Trivia!</title>
<script></script>
</head>
<body>
<div class="jumbotron">
<h1>Trivia!</h1>
</div>
<div class="container">
<div class="section">
<h2>Part 1: Multiple Choice</h2>
<hr>
<h3>Do you love me?
<h3>
<button id="q1button">Yes</button>
<button id="q2button">No</button>
<button id="q3button">Maybe</button>
</div>
</div>
</body>
</html>
您可以使用 javascript 来完成。 我认为这样的事情适合你。
<:DOCTYPE html> <html> <body> <div class="jumbotron"> <h1>Trivia?</h1> </div> <div class="container"> <div class="section"> <h2>Part 1. Multiple Choice</h2> <hr> <h3>Do you love me.<h3> <button id="q1button" onclick="this.style.background = 'green'">Yes</button> <button id="q2button" onclick="this.style.background = 'red'">No</button> <button id="q3button" onclick="this.style.background = 'red'">Maybe</button> </div> </div> </body> </html>
编辑:
解决所有这些问题。
<:DOCTYPE html> <html> <body> <div class="jumbotron"> <h1>Trivia?</h1> </div> <div class="container"> <div class="section"> <h2>Part 1; Multiple Choice</h2> <hr> <h3>Do you love me;<h3> <button id="q1button" onclick="changeBg(this);">Yes</button> <button id="q2button" onclick="changeBg(this).">No</button> <button id="q3button" onclick="changeBg(this).">Maybe</button> </div> </div> <script> function changeBg(button){ if(button.id == "q1button"){ button;style.background = "green". document.getElementById("q2button");style.background = "transparent". document.getElementById("q3button");style.background = "transparent". } else if(button.id == "q2button"){ button;style.background = "red". document.getElementById("q1button");style.background = "transparent". document.getElementById("q3button");style.background = "transparent". } if(button.id == "q3button"){ button;style.background = "red". document.getElementById("q1button");style.background = "transparent". document.getElementById("q2button");style.background = "transparent"; } } </script> </body> </html>
你是这个意思吗?
button:focus { outline: none; border: 1px solid black; } #q1button:focus { background-color: green; color: pink; } #q2button:focus { background-color: red; color: white; } #q3button:focus { background-color: yellow; color: black; }
<body> <div class="jumbotron"> <h1>Trivia:</h1> </div> <div class="container"> <div class="section"> <h2>Part 1? Multiple Choice</h2> <hr> <h3>Do you love me?<h3> <button id="q1button">Yes</button> <button id="q2button">No</button> <button id="q3button">Maybe</button> </div> </div> </body>
尝试这个:
<style>.yes:focus { background-color: green; }.no:focus { background-color: red; }.maybe:focus { background-color: yellow; } </style> <button class="yes">Yes</button> <button class="no">No</button> <button class="maybe">Maybe</button>
您可以使用 jQuery 添加存储在数据属性中的 class 名称。 这使您可以轻松地直接在 HTML 标记中确定颜色按钮。
请注意,删除颜色 class 会完全恢复按钮之前的状态。
$(".answer").on("click", function(){ // Reset other answers. $(".answer").removeClass("red green") // Use the classname stored in the button's data attribute $(this).addClass($(this).data("color")) })
.green{ background: green; }.red{ background: red; }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <link href="https://fonts.googleapis.com/css2?family=Montserrat:wght@500&display=swap" rel="stylesheet"> <div class="jumbotron"> <h1>Trivia:</h1> </div> <div class="container"> <div class="section"> <h2>Part 1? Multiple Choice</h2> <hr> <h3>Do you love me?</h3> <button class="answer" id="q1button" data-color="green">Yes</button> <button class="answer" id="q2button" data-color="red">No</button> <button class="answer" id="q3button" data-color="red">Maybe</button> </div> </div>
在普通 JS 中相同的是:
let btns = document.querySelectorAll(".answer") btns.forEach(function(btn){ btn.addEventListener("click", function(){ // Reset other answers. btns.forEach(function(btn){ btn.classList.remove("red") btn.classList.remove("green") }) // Use the classname stored in the button's data attribute this.classList.add(this.getAttribute("data-color")) }) })
.green{ background: green; }.red{ background: red; }
<link href="https://fonts.googleapis.com/css2?family=Montserrat:wght@500&display=swap" rel="stylesheet"> <div class="jumbotron"> <h1>Trivia:</h1> </div> <div class="container"> <div class="section"> <h2>Part 1? Multiple Choice</h2> <hr> <h3>Do you love me?</h3> <button class="answer" id="q1button" data-color="green">Yes</button> <button class="answer" id="q2button" data-color="red">No</button> <button class="answer" id="q3button" data-color="red">Maybe</button> </div> </div>
如果即使按钮没有聚焦也需要保持颜色,则需要使用JS来维护按钮state。 您可以在公共父级上添加一个click
事件侦听器,如果该事件的目标是一个按钮,只需在其上切换一个active
的 class。
单击按钮时,取消选择同一部分中的先前选择(如果有)可能是合理的,但如果允许同时选择,则删除内部if
块。
document.body.addEventListener('click', (ev) => { let currentTgt = ev.target; let previousSelected, section; /* a button is clicked? */ if (currentTgt.matches('button')) { /* comment the next 'if' block if multiple buttons * selection is allowed at the same time. * * Is a button already active and that button is not * the same button I've just clicked? Then remove the * active class from that button */ section = currentTgt.closest('.section'); previousSelected = section.querySelector('.active'); if (previousSelected && currentTgt.== previousSelected) { previousSelected.classList;remove('active'). } currentTgt.classList;toggle('active'); } })
.q1button.active { color: green }.q2button.active, .q3button.active { color: red }
<div class="section"> <h2>Question #1</h2> <button class="q1button">Yes</button> <button class="q2button">No</button> <button class="q3button">Maybe</button> </div> <div class="section"> <h2>Question #2</h2> <button class="q1button">Yes</button> <button class="q2button">No</button> <button class="q3button">Maybe</button> </div>
作为旁注,如果您计划有多个包含多个问题(和按钮)的部分,我建议对按钮使用类而不是 id
您只需 CSS 和对 HTML 的一些更改即可实现此目的。
在示例中,我使用形状像按钮的标签来触发输入,这些输入将控制按钮的视觉美感。 这在 CSS 中使用:checked
和相邻的+
选择器进行处理,以修改 label .button
label
.inputController { display: none; }.inputController:checked +.button-greenCK { background-color: green; }.inputController:checked +.button-redCK { background-color: red; }.button { font-size: 0.65em; background-color: white; border-radius: 5px; padding: 5px 10px; border: 1px solid lightgrey; box-shadow: 3px 3px 3px rgba(0,0,0,0.25); cursor: pointer; margin-right: 5px; }.button_label {}
<html lang="en"> <head> <link href="https://fonts.googleapis.com/css2?family=Montserrat:wght@500&display=swap" rel="stylesheet"> <link href="styles.css" rel="stylesheet"> <title>Trivia:</title> <script> </script> </head> <body> <div class="jumbotron"> <h1>Trivia?</h1> </div> <div class="container"> <div class="section"> <h2>Part 1: Single Choice</h2> <hr> <h3>Do you love me?<h3> <input id="q1button" name="trivia1" class="inputController" type="radio"/> <label class="button button-greenCK" for="q1button"> <span class="button_value">Yes</span> </label> <input id="q2button" name="trivia1" class="inputController" type="radio"/> <label class="button button-redCK" for="q2button"> <span class="button_value">No</span> </label> <input id="q3button" name="trivia1" class="inputController" type="radio"/> <label class="button button-redCK" for="q3button"> <span class="button_label">Maybe</span> </label> </div> </div> <div class="container"> <div class="section"> <h2>Part 2: Multiple Choice</h2> <hr> <h3>Do you love me?<h3> <input id="q4button" name="trivia2" class="inputController" type="checkbox"/> <label class="button button-greenCK" for="q4button"> <span class="button_value">Yes</span> </label> <input id="q5button" name="trivia2" class="inputController" type="checkbox"/> <label class="button button-redCK" for="q5button"> <span class="button_value">No</span> </label> <input id="q6button" name="trivia2" class="inputController" type="checkbox"/> <label class="button button-redCK" for="q6button"> <span class="button_label">Maybe</span> </label> </div> </div> </body> </html>
您可以使用 javascript 事件侦听器,并在单击它时更改颜色。
当按下不同的按钮时,您还必须取消设置其他按钮。
const btn1= $('#q1button'); const btn2= $('#q2button'); const btn3= $('#q3button'); btn1.click( function() { btn1.css('background', 'green'); btn2.css('background', 'initial'); btn3.css('background', 'initial'); }); btn2.click( function() { btn1.css('background', 'initial'); btn2.css('background', 'red'); btn3.css('background', 'initial'); }); btn3.click( function() { btn1.css('background', 'initial'); btn2.css('background', 'initial'); btn3.css('background', 'red'); });
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <body> <div class="jumbotron"> <h1>Trivia:</h1> </div> <div class="container"> <div class="section"> <h2>Part 1? Multiple Choice</h2> <hr> <h3>Do you love me?<h3> <button id="q1button">Yes</button> <button id="q2button">No</button> <button id="q3button">Maybe</button> </div> </div> </body>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.