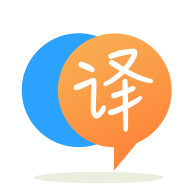
[英]ASP.NET Core - How to update existing record and insert a new one at the same time using Entity Framework
[英]How to insert a record or multiple records using entity framework core using dbcontext (soap ui sends data to asp.net core via api call)
当实体框架收到来自 soap ui 的 post 请求形式的输入时,它应该将数据记录或多个数据记录(行)插入 BdpMigrationList 表并返回确认。 使用 sccafold 命令将 BdpMigrationList 添加到实体。
Model:
public class BDPHMMigModel
{
public string CmNo { get; set; }
public DateTime CmDate { get; set; }
public string Ipact { get; set; }
public string Site { get; set; }
public string Version { get; set; }
public string Circuit { get; set; }
public string Customer { get; set; }
public string SourceDevice { get; set; }
public string SourceInterface { get; set; }
public string SourceInterfaceLag { get; set; }
public string DestDevice { get; set; }
public string DestInterface { get; set; }
public string DestInterfaceLag { get; set; }
public int Id { get; set; }
}
这是我的数据访问层代码:还请推荐我应该在 DAL 代码中使用哪种 class 类型。
public async Task<int> AddBdpHmMigData(BdpMigrationList migrationList)
{
var context = new IpReservationContext();
object newcircuit = new BdpMigrationList
{
CmNo = migrationList.CmNo,
CmDate = migrationList.CmDate,
Ipact = migrationList.Ipact,
Site = migrationList.Site,
Version = migrationList.Version,
Circuit = migrationList.Circuit,
Customer = migrationList.Customer,
SourceDevice = migrationList.SourceDevice,
SourceInterface = migrationList.SourceInterface,
SourceInterfaceLag = migrationList.SourceInterfaceLag,
DestDevice = migrationList.DestDevice,
DestInterface = migrationList.DestInterface,
DestInterfaceLag = migrationList.DestInterfaceLag
};
context.Add(newcircuit);
context.SaveChanges();
}
Controller:
[HttpPost]
public async Task<IActionResult> AddBdpHmMigData([FromBody]BDPHMMigModel model)
{
if (ModelState.IsValid)
{
try
{
var miglistID = await BdpHmDao.AddBdpHmMigData(model);
if (miglistID > 0)
{
return Ok(miglistID);
}
else
{
return NotFound();
}
}
catch (Exception)
{
return BadRequest();
}
}
return BadRequest();
BdpMigrationList class 在实体:
[Table("BDP_Migration_List")]
public partial class BdpMigrationList
{
[Required]
[Column("CM_No")]
[StringLength(50)]
public string CmNo { get; set; }
[Column("CM_date", TypeName = "datetime")]
public DateTime CmDate { get; set; }
[Column("IPACT")]
[StringLength(50)]
public string Ipact { get; set; }
[StringLength(10)]
public string Site { get; set; }
[StringLength(10)]
public string Version { get; set; }
[Required]
[StringLength(60)]
public string Circuit { get; set; }
[StringLength(150)]
public string Customer { get; set; }
[Column("Source_Device")]
[StringLength(50)]
public string SourceDevice { get; set; }
[Column("Source_Interface")]
[StringLength(50)]
public string SourceInterface { get; set; }
[Column("Source_Interface_Lag")]
[StringLength(50)]
public string SourceInterfaceLag { get; set; }
[Column("Dest_Device")]
[StringLength(50)]
public string DestDevice { get; set; }
[Column("Dest_Interface")]
[StringLength(50)]
public string DestInterface { get; set; }
[Column("Dest_Interface_Lag")]
[StringLength(50)]
public string DestInterfaceLag { get; set; }
[Key]
[Column("ID")]
public int Id { get; set; }
}
数据库上下文:
public partial class IpReservationContext : DbContext
{
public IpReservationContext()
{
}
public IpReservationContext(DbContextOptions<IpReservationContext> options)
: base(options)
{
}
public virtual DbSet<BdpMigrationList> BdpMigrationList { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<BdpMigrationList>(entity =>
{
entity.Property(e => e.Circuit).IsUnicode(false);
entity.Property(e => e.CmNo).IsUnicode(false);
entity.Property(e => e.Customer).IsUnicode(false);
entity.Property(e => e.DestDevice).IsUnicode(false);
entity.Property(e => e.DestInterface).IsUnicode(false);
entity.Property(e => e.DestInterfaceLag).IsUnicode(false);
entity.Property(e => e.Ipact).IsUnicode(false);
entity.Property(e => e.Site).IsUnicode(false);
entity.Property(e => e.SourceDevice).IsUnicode(false);
entity.Property(e => e.SourceInterface).IsUnicode(false);
entity.Property(e => e.SourceInterfaceLag).IsUnicode(false);
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
收到以下错误:
Error CS1503 Argument 1: cannot convert from 'homing_matrix_api.Model.BDPHMMigModel' to 'homing_matrix_api.Entity.IpReservation.BdpMigrationList' homing-matrix-api C:\Repositories\HomingMatrix\HomingMatrixAPI\Controllers\BdpHmController.cs 55 Active
错误 CS0161 'BdpHmDao.AddBdpHmMigData(BdpMigrationList)':并非所有代码路径都返回值 homing-matrix-api C:\Repositories\HomingMatrix\HomingMatrixAPI\Data\BdpHmDao.cs 51 Active
警告 CS1998 此异步方法缺少“等待”运算符,将同步运行。 考虑使用“await”运算符来等待非阻塞 API 调用,或“await Task.Run(...)”在后台线程上执行 CPU 密集型工作。 归位矩阵 API C:\Repositories\HomingMatrix\HomingMatrixAPI\Data\BdpHmDao.cs 51 活动
试试这个代码:
修复您的 controller 代码:
using ... Data Access layer name space
....
......
var bdp=new bdpHmDao();
var miglistID = await bdp.AddBdpHmMigData(model);
修复您的操作代码
public async Task<int> AddBdpHmMigData(BDPHMMigModel model)
{
using (var context = new IpReservationContext())
{
var newcircuit = BdpMigrationList
{
CmNo = model.CmNo,
CmDate = model.CmDate,
Ipact = model.Ipact,
Site = model.Site,
Version = model.Version,
Circuit = model.Circuit,
Customer = model.Customer,
SourceDevice = model.SourceDevice,
SourceInterface = model.SourceInterface,
SourceInterfaceLag = model.SourceInterfaceLag,
DestDevice = model.DestDevice,
DestInterface = model.DestInterface,
DestInterfaceLag = model.DestInterfaceLag
};
context.Set<BdpMigrationList>().Add(newcircuit);
return await context.SaveChangesAsync();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.