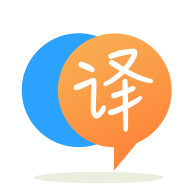
[英]Using readAllBytes to read a file's bytes, but output is not changing when I change the file
[英]How to read bytes from a file into a single string using readAllBytes?
我正在使用算法 class,我们必须在 Java 中实现 LZW 压缩。 我决定为此使用 Trie 数据结构,并且我已经实现了 Trie 并让它工作。
现在,我想从文件中读取字节,将它们转换为填充二进制(00000001 而不是 01),然后将它们存储在我的 Trie 中。 我不需要 Trie 的帮助,而是读取文件的内容。
我尝试使用 readAllBytes 来读取内容,并将每个转换后的字节附加到 StringBuilder,但是当我这样做时,我得到了一个充满 48 和 49 的 StringBuilder。 我认为我的二进制数据正在转换为我不想要的 ASCII。 我只是想要一个带有 1 和 0 的字符串。
我已经得到了下面的方法,但它依赖于 ArrayList 而不是字符串。 它也不使用 readAllBytes,而且速度很慢。 (我无法让 readAllBytes 工作,它只是给了我一个无限循环)。 我们将根据表现进行评分。
File file = new File(path);
ArrayList<String> codes = new ArrayList<String>();
try (FileInputStream fileInputStream = new FileInputStream(file)) {
int singleCharInt;
while ((singleCharInt = fileInputStream.read()) != -1) {
codes.add(Integer.toBinaryString((singleCharInt & 0xFF) + 0x100).substring(1));
}
}
return codes;
感谢您的时间!
我所有填充的二进制值都转换为 ASCII,所以我的字符串用 48、49 等填充,而不是原始日期。
这听起来就像你只想读取一个文件。 在您阅读它之前,您需要知道它采用什么字符集编码。您无法从文件或扩展名中分辨出来(至少,通常不会),您必须知道。 如果您不知道,UTF-8 是一个不错的选择,这是文件 API 的默认设置。
Path p = Paths.get("/path/to/file.txt");
String string = Files.readString(p);
这就是你需要做的。 无需涉及readAllBytes
。 如果必须(只有在根本没有文件但 InputStream 形式的其他文件时才应该这样做):
String s;
try (InputStream in = ...) {
s = new String(in.readAllBytes(), StandardCharsets.UTF_8);
}
您可以使用StringBuffer
或StringBuilder
class 来生成最终的字符串结果。
StringBuffer buffer = new StringBuffer();
try (FileInputStream fileInputStream = new FileInputStream(file)) {
int singleCharInt;
while ((singleCharInt = fileInputStream.read()) != -1) {
buffer.append(Integer.toBinaryString((singleCharInt & 0xFF) + 0x100));
}
}
// Result: buffer.toString()
您可以从这样的文件中获取 btyeCode;
public static byte[] readFileNio(String physicalPath) throwsFileNotFoundException, IOException{
RandomAccessFile aFile = new RandomAccessFile(physicalPath,"r");
FileChannel inChannel = aFile.getChannel();
ByteBuffer buffer = ByteBuffer.allocate((int)inChannel.size());
inChannel.read(buffer);
buffer.flip();
inChannel.close();
aFile.close();
return buffer.array();
}
然后你可以将字节码写入任何特定的地方
Files.write(newPath, readFileNio(fromPath), StandardOpenOption.CREATE_NEW);
用于压缩使用ZipOutputStream
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.