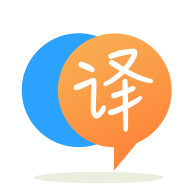
[英]Calling child functional component method from parent functional component in React
[英]Calling a children method of a functional component from parent
我没有使用 class,我想学习如何手动操作。 我正在处理登录屏幕。 https://snack.expo.io/@ericsia/call-function-from-child-component如果你想给我看你的代码,你需要保存并分享链接。 所以我想要一个用于显示文本框的功能组件(假设ChildComponent
为 function 名称,所以export ChildComponent
)。
所以在 Parent/Screen1 我有这样的事情吗?
import * as React from 'react';
import { Text, View, StyleSheet, TouchableOpacity } from 'react-native';
import Constants from 'expo-constants';
// You can import from local files
import ChildComponent from './components/ChildComponent';
export default function App() {
function checkSuccess()
{
// call helloWorld from here
}
return (
<View style={styles.container}>
<ChildComponent />
<TouchableOpacity style={styles.button}
onPress={ checkSuccess } >
<Text>helloWorld ChildComponent</Text>
</TouchableOpacity>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: Constants.statusBarHeight,
backgroundColor: '#ecf0f1',
padding: 8,
},
button: {
alignItems: "center",
backgroundColor: "#DDDDDD",
padding: 10
},
});
所以如果结果是无效的,我想显示一个小小的红色错误消息。 我的方法是,如果我可以从ChildComponent
调用 function,那么我仍然可以解决它。
我用谷歌搜索了它,提供的大多数解决方案都是针对 class 的。 我尝试useEffect
React.createRef
useImperativeHandle
但我没有得到它的工作。
首先,我只是想调用helloWorld()
import * as React from 'react';
import { TextInput , View, StyleSheet, Image } from 'react-native';
export default function ChildComponent() {
function helloWorld()
{
alert("Hello World");
}
return (<TextInput placeholder="Try to call helloWorld from App.js"/>);
}
另一个问题,如果我的 ChildComponent 中有一个文本框,我如何从父级检索文本/值?
您可以将helloWorld
function 向上移动到父组件,并将其作为道具传递给子组件。 这样两个组件都可以调用它。 当您要绕过 function 时,我建议使用箭头 function ,尽管在这种情况下并不重要。
家长
export default function App() {
const helloWorld = () => {
alert('Hello World');
}
const checkSuccess = () => {
helloWorld();
}
return (
<View style={styles.container}>
<ChildComponent helloWorld={helloWorld} />
<TouchableOpacity style={styles.button} onPress={checkSuccess}>
<Text>helloWorld ChildComponent</Text>
</TouchableOpacity>
</View>
);
}
孩子
const ChildComponent = ({ helloWorld }) => {
// could do anything with helloWorld here
return <TextInput placeholder="Try to call helloWorld from App.js" />;
};
如果要将 function 保留在子组件中,则需要通过很多箍来 go 。 我不推荐这种方法。
您必须执行所有这些步骤:
ref
useRef
: const childRef = React.useRef();
<ChildComponent ref={childRef} />
?.
如果.current
尚未设置,以避免错误: childRef.current?.helloWorld();
forwardRef
接受孩子中的ref
道具: React.forwardRef( (props, ref) => {
useImperativeHandle
将helloWorld
function 公开为子组件的实例变量: React.useImperativeHandle(ref, () => ({helloWorld}));
家长:
export default function App() {
const childRef = React.useRef();
function checkSuccess() {
childRef.current?.helloWorld();
}
return (
<View style={styles.container}>
<ChildComponent ref={childRef} />
<TouchableOpacity style={styles.button} onPress={checkSuccess}>
<Text>helloWorld ChildComponent</Text>
</TouchableOpacity>
</View>
);
}
孩子:
const ChildComponent = React.forwardRef((props, ref) => {
function helloWorld() {
alert('Hello World');
}
React.useImperativeHandle(ref, () => ({ helloWorld }));
return <TextInput placeholder="Try to call helloWorld from App.js" />;
});
编辑: Expo 链接
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.