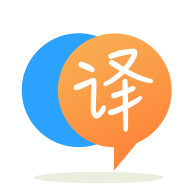
[英]ASP.NET Core MVC application insights stopped working after upgrade to .NET Core 1.1.1
[英]My ASP.NET Core MVC application stopped saving changes
我的 ASP.NET 核心 MVC 应用程序(用 C# 编写)由于某种原因停止在数据库中保存更改。
它读起来很完美,没有任何与连接本身相关的错误。 它昨天工作得很好,但今天它不再工作了。 这是怎么回事? 它可能与“刷新”问题有关。
这是我的 controller 代码:
// POST: Approver/Edit/5
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit(int id, [Bind] Approver approver)
{
try
{
if (ModelState.IsValid)
{
dbContext.UpdateApprover(approver);
return RedirectToAction("Index");
}
return View(dbContext);
}
catch
{
return View();
}
}
这是我的 CONTEXT 更新方法:
public void UpdateApprover(Approver approver)
{
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("Stored_procedure_update", con)
{
CommandType = System.Data.CommandType.StoredProcedure
};
cmd.Parameters.AddWithValue("@CODE", approver.CODE);
cmd.Parameters.AddWithValue("@MAIL", approver.MAIL);
cmd.Parameters.AddWithValue("@NAME", approver.NAME);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
我错过了什么吗?
连接文件:
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using MyApplication.Models;
namespace MyApplication.Context
{
public class Connection
{
string connectionString = "Data Source=xxxxxxx;Initial Catalog = ''; Persist Security Info=True;User ID = ''; Password='xxxxxx'";
/// <summary>
/// </summary>
/// <returns></returns>
public IEnumerable<Approver> GetAllApprovers()
{
var ApproversList = new List<Approver>();
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_GetAllApprovers", con)
{
CommandType = System.Data.CommandType.StoredProcedure
};
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
while(dr.Read())
{
var Approver = new Approver
{
CODE = Convert.ToInt32(dr["CODE"].ToString()),
MAIL = dr["MAIL"].ToString(),
FIRSTNAME = dr["FIRSTNAME"].ToString(),
LASTNAME = dr["LASTNAME"].ToString()
};
ApproversList.Add(Approver);
}
con.Close();
}
return ApproversList;
}
public void UpdateApprover(Approver Approver)
{
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_Update_Approver", con)
{
CommandType = System.Data.CommandType.StoredProcedure
};
cmd.Parameters.AddWithValue("@CODE", Approver.CODE);
cmd.Parameters.AddWithValue("@MAIL", Approver.MAIL);
cmd.Parameters.AddWithValue("@FIRSTNAME", Approver.FIRSTNAME);
cmd.Parameters.AddWithValue("@LASTNAME", Approver.LASTNAME);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
public Approver GetApproverById(int? id)
{
var Approver = new Approver();
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_GetapproverById", con);
cmd.CommandType = System.Data.CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@CODE", id);
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
while(dr.Read())
{
Approver.CODE = Convert.ToInt32(dr["CODE"].ToString());
Approver.MAIL = dr["MAIL"].ToString();
Approver.FIRSTNAME = dr["FIRSTNAME"].ToString();
Approver.LASTNAME = dr["LASTNAME"].ToString();
}
con.Close();
}
return Approver;
}
}
}
CONTROLLER 文件:
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using MyApplication.Context;
using MyApplication.Models;
namespace MyApplication.Controllers
{
public class ControllerApprover : Controller
{
readonly Connection dbContext = new Connection();
// GET: Approver
public ActionResult Index()
{
List<Approver> ApproversList = dbContext.GetAllApprovers().ToList();
return View(ApproversList);
}
// GET: Approver/Details/5
public ActionResult Details(int id)
{
Approver approver = dbContext.GetApproverById(id);
return View(approver);
}
// GET: Approver/Create
public ActionResult Create()
{
return View();
}
// POST: Approver/Create
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(int id, [Bind] Approver approver)
{
try
{
if (ModelState.IsValid)
{
dbContext.UpdateApprover(Approver);
return RedirectToAction("Index");
}
return View(dbContext);
}
catch
{
return View();
}
}
// GET: Approver/Edit/5
public ActionResult Edit(int id)
{
Approver Approver = dbContext.GetApproverById(id);
return View(approver);
}
// POST: Approver/Edit/5
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit(int id, [Bind] Approver approver)
{
try
{
if (ModelState.IsValid)
{
dbContext.UpdateApprover(approver);
return RedirectToAction("Index");
}
return View(dbContext);
}
catch
{
return View();
}
}
}
}
我的 appsettings.json:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"}
这应该在评论部分,但我现在不能评论,需要 50 声望。 但我认为,你可以先试试你的 catch 块是否有错误,将其更改为catch (Exception e)
以查看是否有问题。 记录它,或者在调试模式下放置一个断点。
如果仍然无法正常工作,请正确选择您的连接字符串并将您的 CONTEXT 方法更改为类似的方法。
private IConfiguration Configuration;
public HomeController(IConfiguration _configuration)
{
Configuration = _configuration;
}
public void UpdateApprover(Approver approver)
{
string connectionString= Configuration.GetConnectionString("YOUR CONNECTION STRING NAME");
using (SqlConnection con = new SqlConnection(connectionString))
{
con.Open();
using (SqlCommand cmd = new SqlCommand("Stored_procedure_update", con))
{
CommandType = System.Data.CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@CODE", approver.CODE);
cmd.Parameters.AddWithValue("@MAIL", approver.MAIL);
cmd.Parameters.AddWithValue("@NAME", approver.NAME);
cmd.ExecuteNonQuery();
con.Close();
}
}
}
连接文件:
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using MyApplication.Models;
namespace MyApplication.Context
{
public class Connection
{
string connectionString = "Data Source=xxxxxxx;Initial Catalog = ''; Persist Security Info=True;User ID = ''; Password='xxxxxx'";
/// <summary>
/// </summary>
/// <returns></returns>
public IEnumerable<Approver> GetAllApprovers()
{
var ApproversList = new List<Approver>();
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_GetAllApprovers", con)
{
CommandType = System.Data.CommandType.StoredProcedure
};
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
while(dr.Read())
{
var Approver = new Approver
{
CODE = Convert.ToInt32(dr["CODE"].ToString()),
MAIL = dr["MAIL"].ToString(),
FIRSTNAME = dr["FIRSTNAME"].ToString(),
LASTNAME = dr["LASTNAME"].ToString()
};
ApproversList.Add(Approver);
}
con.Close();
}
return ApproversList;
}
public void UpdateApprover(Approver Approver)
{
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_Update_Approver", con)
{
CommandType = System.Data.CommandType.StoredProcedure
};
cmd.Parameters.AddWithValue("@CODE", Approver.CODE);
cmd.Parameters.AddWithValue("@MAIL", Approver.MAIL);
cmd.Parameters.AddWithValue("@FIRSTNAME", Approver.FIRSTNAME);
cmd.Parameters.AddWithValue("@LASTNAME", Approver.LASTNAME);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
public Approver GetApproverById(int? id)
{
var Approver = new Approver();
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("SP_GetapproverById", con);
cmd.CommandType = System.Data.CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@CODE", id);
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
while(dr.Read())
{
Approver.CODE = Convert.ToInt32(dr["CODE"].ToString());
Approver.MAIL = dr["MAIL"].ToString();
Approver.FIRSTNAME = dr["FIRSTNAME"].ToString();
Approver.LASTNAME = dr["LASTNAME"].ToString();
}
con.Close();
}
return Approver;
}
}
}
CONTROLLER 文件:
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using MyApplication.Context;
using MyApplication.Models;
namespace MyApplication.Controllers
{
public class ControllerApprover : Controller
{
readonly Connection dbContext = new Connection();
// GET: Approver
public ActionResult Index()
{
List<Approver> ApproversList = dbContext.GetAllApprovers().ToList();
return View(ApproversList);
}
// GET: Approver/Details/5
public ActionResult Details(int id)
{
Approver approver = dbContext.GetApproverById(id);
return View(approver);
}
// GET: Approver/Create
public ActionResult Create()
{
return View();
}
// POST: Approver/Create
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(int id, [Bind] Approver approver)
{
try
{
if (ModelState.IsValid)
{
dbContext.UpdateApprover(Approver);
return RedirectToAction("Index");
}
return View(dbContext);
}
catch
{
return View();
}
}
// GET: Approver/Edit/5
public ActionResult Edit(int id)
{
Approver Approver = dbContext.GetApproverById(id);
return View(approver);
}
// POST: Approver/Edit/5
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit(int id, [Bind] Approver approver)
{
try
{
if (ModelState.IsValid)
{
dbContext.UpdateApprover(approver);
return RedirectToAction("Index");
}
return View(dbContext);
}
catch
{
return View();
}
}
}
}
我的 appsettings.json:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.