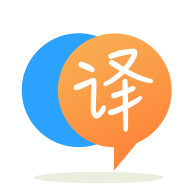
[英]Passing axios fetched prop from Parent to Child returns “TypeError: Cannot read property 'x' of undefined”
[英]'TypeError: Cannot read property of x of undefined' when passing data from parent to child component but others are working
美好的一天,我有一个项目得到 API 的响应,然后将数据从父级传递给子级。 问题是,我可以轻松访问顶层的响应,但是当我尝试进入 API 的内部时(在这种情况下, price={statistics.quotes.USD.price}
),我得到了TypeError: Cannot read property 'USD' of undefined
错误。 我试过控制台。记录价格以检查我的路径是否正确。 当我可以正确访问其他数据时,为什么会发生这种情况?
概述.js
import React, { useState, useEffect } from 'react';
import Statistics from './Statistics';
import axios from 'axios';
export default function Overview(props) {
const id = props.match.params.currency;
//some other states here
const [statistics, setStatistics] = useState({});
//some code
const fetchBasicData = async () => {
// Retrieves a coin's basic information
const apiCall = await axios.get('https://api.coinpaprika.com/v1/coins/' + id);
let basicData = await apiCall.data;
setCoin(basicData);
// Retrieves coin statistics
const fetchedData = await axios.get('https://api.coinpaprika.com/v1/tickers/' + id);
const coinStats = await fetchedData.data;
setStatistics(coinStats);
}
useEffect(function () {
if (Object.keys(coin).length === 0 && Object.keys(statistics).length === 0) {
fetchBasicData();
}
})
//some code
return (
<div>
//some other stuff
<Statistics
statistics={statistics}
lastUpdate={statistics.last_updated}
price={statistics.quotes.USD.price} // <----- this is where the error occurs
/>
</div>
);
}
Statistics.js
import React from 'react';
export default function Statistics(props) {
return (
<div>
<h1>Statistics</h1>
<p>Last updated: {props.lastUpdate}</p>
<p>Price: {props.price}</p>
<p>Market Rank: {props.marketRank}</p>
<h2>Supply</h2>
<p>Circulating supply: {props.circulatingSupply}</p>
<p>Max supply: {props.maxSupply}</p>
</div>
);
}
嗨,可能是您的数据中的某些行没有引号尝试进行以下更改,它应该由此修复
改变
price={statistics.quotes.USD.price}
至
price={statistics?.quotes?.USD?.price}
? 检查给定变量是否存在,如果不存在则返回 null 并且不抛出错误
由于您正在使用axios
调用,这是异步的,并且该调用的数据在初始渲染时不可用,但稍后将可用。 因此,要处理此问题,您必须有条件渲染(仅在满足特定条件时渲染)
尝试这个:
price={statistics?.quotes?.USD?.price}
或者您也可以将Object.hasOwnProperty('key')
与ternary
一起使用并进行条件渲染。
错误: TypeError: Cannot read property 'USD' of undefined
是说statistics.quotes
未定义。
有2个可能的原因:
statistics
都不会更新为您所期望的。我的猜测是您的数据获取和 state 更新很好,这只是初始渲染问题。
初始statistics
state 是一个空的 object ( {}
),因此访问任何属性都可以。 正是当您将 go 嵌套到更深的结构中时,才会导致问题。
<Statistics
statistics={statistics} // OK: statistics => {}
lastUpdate={statistics.last_updated} // OK: statistics.last_updated => undefined
price={statistics.quotes.USD.price} // Error: can't access USD of undefined statistics.quotes
/>
const statistics = {}; console.log(statistics); // {} console.log(statistics.quotes); // undefined console.log(statistics.qoutes.USD); // error!!
您可以使用可选链接运算符( ?.
) 或保护子句(空检查)来保护“未定义的访问 X”错误。
<Statistics
statistics={statistics}
lastUpdate={statistics.last_updated}
price={statistics.quotes?.USD?.price}
/>
<Statistics
statistics={statistics}
lastUpdate={statistics.last_updated}
price={statistics.quotes && statistics.quotes.USD && statistics.quotes.USD.price}
/>
如果您的statistics
state 可以更新为undefined
的可能性很小,那么应用与上述相同的修复,但只是更浅的级别,即statistics?.quotes?.USD?.price
。
或者,您可以应用Statistics
组件的一些条件渲染,其中条件是statistics
state 上存在的嵌套属性。
return (
<div>
//some other stuff
{statistics.last_updated && statistics.quotes && (
<Statistics
statistics={statistics}
lastUpdate={statistics.last_updated}
price={statistics.quotes.USD?.price}
/>
)}
</div>
);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.