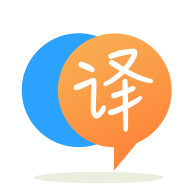
[英]Zoom on a layer in Photoshop with a button using CEP/JavaScript
[英]Draw circle on a new layer in Photoshop using cep/Javascript
对于一个 Photoshop 插件项目,我想创建一个新图层,给它一个名字,画一个特定颜色的圆圈。 我在 Javascript 中编码并使用框架 CEP。
要绘制的圆的坐标如下所示:
linesStr: "2287,3474 | 2268,3430 | 2255,3398 | 2255,3360 | 2255,3315 | 2255,3264 | 2255,3207 | 2261,3162 | 2331,3047 | 2389,3003 | 2433.2977 | 2484.2965 | 2541.2946 | 2580.2946 | 2618.2946 | 2650.2952 | 2688.2971 | 2720.2990 | 2745.3022 | 2764.3054 | 2777.3086 | 2790, 3124 | 2803.3162 | 2803.3207 | 2803.3251 | 2803.3296 | 2783.3360 | 2752.3411 | 2726.3449 | 2707.3493 | 2688.3519 | 2637.3557 | 2611.3570 | 2592.3576 | 2573.3576 | 2554.3589 | 2548.3589 | 2541.3589 | 2535.3589 | 2529.3589 | 2516.3589 | 2510.3589 | 2503.3583 | 2497.3576 | 2497.3557 | 2497.3551 | 2497, 3525 | 2497.3512 | 2497.3493 | 2490.3481 | 2490.3455 | 2478.3442 | 2478.3423 | 2478.3417 | 2471.3398 | 2465.3391 | 2459.3372 | 2452.3366 | 2446.3366 * 2401.3328 | 2389.3321 | 2382.3321 | 2376.3321 | 2369.3315 | 2350.3309 | 2344.3302"。
所以结果应该是这样的:
我很乐意接受任何帮助!
通过稍微调整您提供的脚本,可以调整您的坐标。 您只需要为形状提供一个将创建路径的数组。
您提供的坐标中有一个无关的星号,而不是分隔的 pipe。 以及点而不是逗号。 它们也是颠倒的。 Photoshop 从左上角开始,y 向下增加图像。 我已经对此进行了补偿。
将像素与矢量坐标匹配也取决于分辨率。 目前图像为 72 dpi。 如果发生变化,它将抵消路径大小。
我用画线替换了填充。
function DrawShape(arr)
{
var doc = app.activeDocument;
var y = arr.length;
var i = 0;
// get original height
var imageHeight = app.activeDocument.height.value;
var lineArray = [];
for (i = 0; i < y; i++)
{
lineArray[i] = new PathPointInfo;
lineArray[i].kind = PointKind.CORNERPOINT;
// invert Y
arr[i][1] = imageHeight - arr[i][1];
lineArray[i].anchor = arr[i];
lineArray[i].leftDirection = lineArray[i].anchor;
lineArray[i].rightDirection = lineArray[i].anchor;
}
var lineSubPathArray = new SubPathInfo();
lineSubPathArray.closed = true;
lineSubPathArray.operation = ShapeOperation.SHAPEADD;
lineSubPathArray.entireSubPath = lineArray;
var myPathItem = doc.pathItems.add("myPath", [lineSubPathArray]);
// =======================================================
var idsetd = charIDToTypeID( "setd" );
var desc100 = new ActionDescriptor();
var idnull = charIDToTypeID( "null" );
var ref24 = new ActionReference();
var idChnl = charIDToTypeID( "Chnl" );
var idfsel = charIDToTypeID( "fsel" );
ref24.putProperty( idChnl, idfsel );
desc100.putReference( idnull, ref24 );
var idT = charIDToTypeID( "T " );
var ref25 = new ActionReference();
var idPath = charIDToTypeID( "Path" );
var idOrdn = charIDToTypeID( "Ordn" );
var idTrgt = charIDToTypeID( "Trgt" );
ref25.putEnumerated( idPath, idOrdn, idTrgt );
desc100.putReference( idT, ref25 );
var idVrsn = charIDToTypeID( "Vrsn" );
desc100.putInteger( idVrsn, 1 );
var idvectorMaskParams = stringIDToTypeID( "vectorMaskParams" );
desc100.putBoolean( idvectorMaskParams, true );
executeAction( idsetd, desc100, DialogModes.NO );
// draw a pixel line in red around the path
stroke_line(2, 215, 5, 5);
// remove selectrion path to pixels
deselect_path();
// select nothing
app.activeDocument.selection.deselect();
// var desc88 = new ActionDescriptor();
// var ref60 = new ActionReference();
// ref60.putClass(stringIDToTypeID("contentLayer"));
// desc88.putReference(charIDToTypeID("null"), ref60);
// var desc89 = new ActionDescriptor();
// var desc90 = new ActionDescriptor();
// var desc91 = new ActionDescriptor();
// desc91.putDouble(charIDToTypeID("Rd "), 0.000000); // R
// desc91.putDouble(charIDToTypeID("Grn "), 0.000000); // G
// desc91.putDouble(charIDToTypeID("Bl "), 0.000000); // B
// var id481 = charIDToTypeID("RGBC");
// desc90.putObject(charIDToTypeID("Clr "), id481, desc91);
// desc89.putObject(charIDToTypeID("Type"), stringIDToTypeID("solidColorLayer"), desc90);
// desc88.putObject(charIDToTypeID("Usng"), stringIDToTypeID("contentLayer"), desc89);
// executeAction(charIDToTypeID("Mk "), desc88, DialogModes.NO);
// myPathItem.remove();
}
function stroke_line(strokewidth, R, G, B)
{
// =======================================================
var idStrk = charIDToTypeID( "Strk" );
var desc2613 = new ActionDescriptor();
var idWdth = charIDToTypeID( "Wdth" );
desc2613.putInteger( idWdth, strokewidth );
var idLctn = charIDToTypeID( "Lctn" );
var idStrL = charIDToTypeID( "StrL" );
var idInsd = charIDToTypeID( "Insd" );
desc2613.putEnumerated( idLctn, idStrL, idInsd );
var idOpct = charIDToTypeID( "Opct" );
var idPrc = charIDToTypeID( "#Prc" );
desc2613.putUnitDouble( idOpct, idPrc, 100.000000 ); // opacity
var idMd = charIDToTypeID( "Md " );
var idBlnM = charIDToTypeID( "BlnM" );
var idNrml = charIDToTypeID( "Nrml" );
desc2613.putEnumerated( idMd, idBlnM, idNrml );
var idClr = charIDToTypeID( "Clr " );
var desc2614 = new ActionDescriptor();
var idRd = charIDToTypeID( "Rd " );
desc2614.putDouble( idRd, R ); // RED
var idGrn = charIDToTypeID( "Grn " );
desc2614.putDouble( idGrn, G ); // GREEN
var idBl = charIDToTypeID( "Bl " );
desc2614.putDouble( idBl, B ); // BLUE
var idRGBC = charIDToTypeID( "RGBC" );
desc2613.putObject( idClr, idRGBC, desc2614 );
executeAction( idStrk, desc2613, DialogModes.NO );
}
function deselect_path()
{
//deselect path
// =======================================================
var id630 = charIDToTypeID( "Dslc" );
var desc154 = new ActionDescriptor();
var id631 = charIDToTypeID( "null" );
var ref127 = new ActionReference();
var id632 = charIDToTypeID( "Path" );
ref127.putClass( id632 );
desc154.putReference( id631, ref127 );
executeAction( id630, desc154, DialogModes.NO );
}
var myArr = [
[2287,3474],
[2268,3430],
[2255,3398],
[2255,3360],
[2255,3315],
[2255,3264],
[2255,3207],
[2261,3162],
[2331,3047],
[2389,3003],
[2433,2977],
[2484,2965],
[2541,2946],
[2580,2946],
[2618,2946],
[2650,2952],
[2688,2971],
[2720,2990],
[2745,3022],
[2764,3054],
[2777,3086],
[2790,3124],
[2803,3162],
[2803,3207],
[2803,3251],
[2803,3296],
[2783,3360],
[2752,3411],
[2726,3449],
[2707,3493],
[2688,3519],
[2637,3557],
[2611,3570],
[2592,3576],
[2573,3576],
[2554,3589],
[2548,3589],
[2541,3589],
[2535,3589],
[2529,3589],
[2516,3589],
[2510,3589],
[2503,3583],
[2497,3576],
[2497,3557],
[2497,3551],
[2497,3525],
[2497,3512],
[2497,3493],
[2490,3481],
[2490,3455],
[2478,3442],
[2478,3423],
[2478,3417],
[2471,3398],
[2465,3391],
[2459,3372],
[2452,3366],
[2446,3366],
[2401,3328],
[2389,3321],
[2382,3321],
[2376,3321],
[2369,3315],
[2350,3309],
[2344,3302],
]
DrawShape(myArr);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.