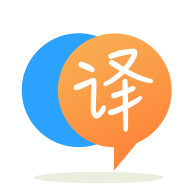
[英]Angular router.navigate() is not working after being redirected using AuthGaurd
[英]Why am I not redirected using router.navigate() in After successful registration and login of a user in Angular11?
这个问题可能看起来很长,但对我来说真的很重要。 我成功地在 firebase 中注册了一个新用户,但没有重定向到“/chatroomlist”路径。 我尝试了所有可能的方法请帮助我!
app.module.ts
import { AuthGuardService } from './auth-guard.service';
import { RouterModule } from '@angular/router';
import { environment } from './../environments/environment';
import { NgModule } from '@angular/core';
import { AngularFireModule } from '@angular/fire';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { AngularFireAuthModule } from '@angular/fire/auth';
import { AngularFireDatabaseModule } from '@angular/fire/database';
import { RegisterComponent } from './register/register.component'
import { AuthService } from './auth.service';
import { LoginComponent } from './login/login.component';
import { ChatroomlistComponent } from './chatroomlist/chatroomlist.component';
@NgModule({
declarations: [
AppComponent,
RegisterComponent,
LoginComponent,
ChatroomlistComponent
],
imports: [
BrowserModule,
AppRoutingModule,
AngularFireModule.initializeApp(environment.firebase),
AngularFireAuthModule,
AngularFireDatabaseModule,
RouterModule.forRoot([
{ path: '', component: RegisterComponent },
{ path: 'login', component: LoginComponent },
{ path: 'register', component: RegisterComponent },
{ path: 'chatroomlist', component: ChatroomlistComponent, canActivate: [AuthGuardService] }
])
],
providers: [
AuthService,
AuthGuardService
],
bootstrap: [AppComponent]
})
export class AppModule { }
auth.service.ts
import { AngularFireAuth } from '@angular/fire/auth';
import { Injectable } from '@angular/core';
import { ActivatedRoute, Router } from '@angular/router';
import { Observable } from 'rxjs';
import * as firebase from 'firebase';
@Injectable({
providedIn: 'root'
})
export class AuthService {
constructor(private afAuth: AngularFireAuth, private router: Router, private route: ActivatedRoute) { }
register(email: any, password: any){
return firebase.default.auth().createUserWithEmailAndPassword(email, password)
.then(() => {
this.router.navigate(['chatroomlist']); // here, .then() never works. It is not redirected
}).catch((error) => {
console.log(error);
})
}
login(email: any, password: any){
return firebase.default.auth().signInWithEmailAndPassword(email, password)
.then(() => {
this.router.navigate(['chatroomlist']); //this path is also not getting redirected. I tried ['/chatroomlist'] too. but it's not working
}).catch((error) =>
console.log(error)
)
}
}
注册组件.ts
import { AuthService } from './../auth.service';
import { AngularFireAuth } from '@angular/fire/auth';
import { Component } from '@angular/core';
@Component({
selector: 'register',
templateUrl: './register.component.html',
styleUrls: ['./register.component.css']
})
export class RegisterComponent {
constructor(private authService: AuthService) { }
register(email: any, password: any){
this.authService.register(email, password);
}
}
登录组件.ts
import { AuthService } from './../auth.service';
import { Component } from '@angular/core';
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.css']
})
export class LoginComponent {
constructor(public authService: AuthService) { }
login(email: any, password: any) {
this.authService.login(email, password);
}
}
register.component.html
<div class="container">
<h2>Registration</h2><br>
<form>
<div class="form-group">
<label for="inputEmail">Email address</label>
<input #email type="email" class="form-control" aria-describedby="emailHelp" placeholder="Enter email" required>
</div>
<div class="form-group">
<label for="inputPassword">Password</label>
<input #password type="password" class="form-control" placeholder="Password" required>
</div>
<div>
<button type="submit" class="btn btn-primary" (click)="register(email.value, password.value)">Register</button><br>
<a routerLink="/login">Already a user? Please Login here!</a>
</div>
</form>
</div>
login.component.html
<div class="container">
<h2>Login</h2><br>
<form>
<div class="form-group">
<label for="inputEmail">Email address</label>
<input #email type="email" class="form-control" aria-describedby="emailHelp" placeholder="Enter email" required>
</div>
<div class="form-group">
<label for="inputPassword">Password</label>
<input #password type="password" class="form-control" placeholder="Password" required>
</div>
<div>
<button type="submit" class="btn btn-primary" (click)="login(email.value, password.value)">Login</button><br>
<a routerLink="/register">New user? Register here!</a>
</div>
</form>
</div>
尝试在服务的组件中使用 router.navigate,因为组件是您在路由器中使用的:
RouterModule.forRoot([
{ path: '', component: RegisterComponent },
{ path: 'login', component: LoginComponent },
{ path: 'register', component: RegisterComponent },
{ path: 'chatroomlist', component: ChatroomlistComponent, canActivate: [AuthGuardService] }
])
因此,现在尝试从服务返回 promise:
register(email:string, password:string){
return new Promise((resolve, rejected)=> {
this.AngularAuth.auth.createUserWithEmailAndPassword(email,password).then(user => {
resolve(user)
}).catch(err => rejected(err));
});
}
在注册组件中:
register(email: any, password: any){
this.authService.register(email, password).then(this.router.navigate(['chatroomlist']));
}
记得在构造函数中添加路由器
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.