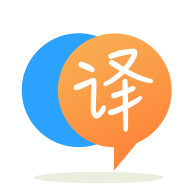
[英]Looping Through Multidimensional Array to iterate each item in javascript
[英]Javascript: How to Iterate Through An Array, Moving Last Item to First Item Each Time
JavaScript 的新功能。 我试图遍历一个数组,每次删除最后一项并将其添加为新的第一项,并在每次循环后控制台记录新数组。 例如,如果我从这个数组开始:
['1', '2', '3', '4', '5']
下一个数组如下所示:
['5', '1', '2', '3', '4']
然后这个:
['4', '5', '1', '2', '3']
等等,直到数组基本上返回到原始的['1', '2', '3', '4', '5']
。
我试过这个:
newArray = ['1', '2', '3', '4', '5']
console.log(newArray)
newArray.forEach(function() {
lastItem = newArray[-1]; // Define "lastItem" as the value of the last item in the array
newArray.pop(); // Remove the last item from the array
newArray.unshift(lastItem); // Add the value of "lastItem" to the front of the array
console.log(newArray);
});
这似乎是正确的轨道,因为 JS 每次都试图删除我的最后一个项目并将其添加到前面。 但是,新的前面项目每次都返回为“未定义”,如下所示:
["1", "2", "3", "4", "5"]
[undefined, "1", "2", "3", "4"]
[undefined, undefined, "1", "2", "3"]
[undefined, undefined, undefined, "1", "2"]
[undefined, undefined, undefined, undefined, "1"]
[undefined, undefined, undefined, undefined, undefined]
为什么每次循环遍历时,JS 都无法将我的lastItem
识别为数组中 [-1] 字段中的值? 我应该以不同的方式解决这个问题吗?
你可以简单地这样做:
newArray = ['1', '2', '3', '4', '5'] newArray.forEach(function() { newArray.push(newArray.shift()); console.log(newArray) });
newArray.push(...) 在后面添加一个新值
newArray.shift() 删除数组的第一个元素,但首先返回它的值。
首先,你永远不应该迭代一个变异数组,否则你最终会遇到问题。 对于将最后一个项目移动到第一个项目的问题,我会使用功能方法 go 。
const data = [1,2,3,4,5]; function rotate(ar) { return [ ar[ar.length -1], ...ar.slice(0, -1) ]; } const iteration1 = rotate(data); const iteration2 = rotate(iteration1); console.log(iteration1) console.log(iteration2)
已解决,提供了几种可行的解决方案:但这是代码量最少的解决方案:
newArray = ['1', '2', '3', '4', '5']
console.log(newArray)
newArray.forEach(function() {
newArray.unshift(newArray.pop());
console.log(newArray)
});
这会在控制台日志中正确返回以下内容:
["1", "2", "3", "4", "5"]
["5", "1", "2", "3", "4"]
["4", "5", "1", "2", "3"]
["3", "4", "5", "1", "2"]
["2", "3", "4", "5", "1"]
["1", "2", "3", "4", "5"]
只需尝试使用array[array.length - 1]
作为最后一个元素,如您所见,JS 中未定义索引-1
和负索引
JavaScript arrays 是 collections 的项目,其中每个项目都可以通过索引访问。 这些索引是非负整数,访问负索引只会返回 undefined
注意:如果你因为某种原因坚持使用负索引,你可以定义一个Proxy
const letters = ['a', 'b', 'c', 'd', 'e'];
const proxy = new Proxy(letters, {
get(target, prop) {
if (!isNaN(prop)) {
prop = parseInt(prop, 10);
if (prop < 0) {
prop += target.length;
}
}
return target[prop];
}
});
proxy[0]; // => 'a'
proxy[-1]; // => 'e'
proxy[-2]; // => 'd'
这篇Medium 文章对此进行了更多解释
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.