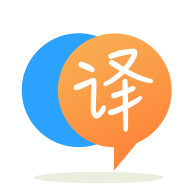
[英]How to Merge two array of objects based on same key and value in javascript?
[英]Merge two array of objects based on same key and value
我有一个看起来像这样的数组:
[
{
like_id: 1,
likes: 1,
post_id: 1,
type: "feed_post_likes",
_id: "b51771798ed01795cb8a17b028000329"
},
{
post_id: 1,
created_date: "2021-03-10T10:54:35.264Z",
post_message: "Happy",
post_pic: "",
post_type: "post"
}
]
我需要根据 post_id 合并这些数组并得到这个:
[
{
like_id: 1,
likes: 1,
post_id: 1,
type: "feed_post_likes",
_id: "b51771798ed01795cb8a17b028000329",
created_date: "2021-03-10T10:54:35.264Z",
post_message: "Happy",
post_pic: "",
post_type: "post"
}
]
您可以使用数组减少方法执行以下操作,
arr = [ { like_id: 1, likes: 1, post_id: 1, type: "feed_post_likes", _id: "b51771798ed01795cb8a17b028000329" }, { post_id: 1, created_date: "2021-03-10T10:54:35.264Z", post_message: "Happy", post_pic: "", post_type: "post" } ]; ret = arr.reduce((prev, curr) => { const idx = prev.findIndex(item => item.post_id === curr.post_id); if(idx > -1) { prev[idx] = {...prev[idx], ...curr}; } else { prev.push(curr); } return prev; }, []) console.log(ret);
这是我提出的一个解决方案:
const arrayTest = [ {like_id: 1, likes: 1, post_id: 1, type: "feed_post_likes", _id: "b51771798ed01795cb8a17b028000329"}, {post_id: 1, created_date: "2021-03-10T10:54:35.264Z", post_message: "Happy", post_pic: "", post_type: "post"} ] const result = arrayTest.reduce((acc, curr) => { const hasIdAlready = acc.some(e => e.post_id === curr.post_id); if (hasIdAlready) { return acc } const foundMatchingElems = arrayTest.filter(e => e.post_id === curr.post_id) const mergedObjects = foundMatchingElems.reduce((obj, e) => ({...obj, ...e}), {}) return [...acc, mergedObjects] }, []) console.log(result)
您可以使用Array.prototype.reduce
合并具有相同post_id
的对象,然后使用Object.values
获取合并对象的数组。
const items = [ { like_id: 1, likes: 1, post_id: 1, type: 'feed_post_likes', _id: 'b51771798ed01795cb8a17b028000329' }, { post_id: 1, created_date: '2021-03-10T10:54:35.264Z', post_message: 'Happy', post_pic: '', post_type: 'post' } ] const result = items.reduce((acc, item) => ({...acc, [item.post_id]: {...acc[item.post_id] || {}, ...item } }), {}) console.log(Object.values(result))
您可以遍历存储已处理的每个post_id
的对象数组,对于尚未处理的对象,您可以使用array.filter()
返回具有给定post_id
的对象数组,然后返回array.reduce()
使用Object.assign()
将所有对象合并为一个:
var arr = [ { like_id: 1, likes: 1, post_id: 1, type: "feed_post_likes", _id: "b51771798ed01795cb8a17b028000329" }, { post_id: 1, created_date: "2021-03-10T10:54:35.264Z", post_message: "Happy", post_pic: "", post_type: "post" } ]; let processed = []; let result = []; for(obj in arr) { if(processed.indexOf(arr[obj].post_id) < 0) { processed.push(arr[obj].post_id); result.push(arr.filter(item => item.post_id == arr[obj].post_id).reduce((acc, currVal) => Object.assign(acc, currVal), {})); } } console.log(result);
这是一个使用Map
的选项,使用for...of
循环迭代输入并使用Object.assign()
合并具有相同post.id
的对象。 结果是将Map
的.values()
迭代器转换为数组。
const input = [{ like_id: 1, likes: 1, post_id: 1, type: "feed_post_likes", _id: "b51771798ed01795cb8a17b028000329" }, { post_id: 1, created_date: "2021-03-10T10:54:35.264Z", post_message: "Happy", post_pic: "", post_type: "post" }]; const grouped = new Map(input.map(like => [like.post_id, {}])); for (let like of input) { Object.assign(grouped.get(like.post_id), like); } const result = [...grouped.values()]; console.log(result)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.