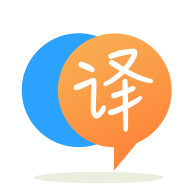
[英]Overriding methods from JavaScript function-based classes in TypeScript
[英]calling React function-based component from vanilla JavaScript DOM
我已经使用基于类的组件实现了这一点。 但是在我尝试使用 React Hooks 时希望使用基于函数的组件来获得答案。
核心问题是我想从 JavaScript DOM 中调用一个反应 function 在数据表操作列内的编辑按钮上单击。
我有一个以index.js
作为入口点的基于 CRUD 的反应组件。 里面有一个ListPage
组件。 但是在反应render/return
方法中没有渲染行。 它一直在使用 JavaScipt Datatable 进行渲染。 数据表行有一个按钮来编辑记录。 该按钮的onClick
我想更新 React 状态以显示/隐藏编辑表单。
index.js:
import React, { useState } from "react";
import ReactDOM from "react-dom";
import CardWrapper from "../../html/wrappers/Card";
import ListPage from "./ListPage";
const Organization = () => {
const page = {
title : "Organization List",
action: "list"
}
const [state, setState] = useState(page);
const showEditForm = () => {
console.log('Edit Organizatioin');
}
return(
<div>
<CardWrapper title={page.title}>
{page.action === 'list' && <ListPage editRecord={showEditForm} />}
</CardWrapper>
</div>
)
};
const domContainer = document.getElementById("react-app");
ReactDOM.render(<Organization
ref={(Organization) => { window.Organization = Organization }}
/>, domContainer);
ListPage.js:
import React, { createRef, useState } from "react";
import Config from "../../../config";
import SearchForm from "../../html/common/SearchForm";
const ListPage = (props) => {
editRef = createRef(props.editRecord);
return(
<div>
<SearchForm placeholder="keyword"
id="kt_datatable_search_query"
buttonId="kt_datatable_search_submit"
/>
<div id="kt_datatable"></div>
</div>
)
}
"use strict";
// Class definition
var KTDatatableBasic = function() {
// Private functions
// basic demo
var initDatatable = function() {
var datatable = $('#kt_datatable').KTDatatable({
// datasource definition
data: {
type: 'remote',
source: {
read: {
url: 'http://localhost:8001/api/settings/organizations/',
method: 'GET',
headers: Config.headers,
// sample custom headers
// headers: {'x-my-custom-header': 'some value', 'x-test-header': 'the value'},
// map: function(raw) {
// // sample data mapping
// var dataSet = raw;
// if (typeof raw.data !== 'undefined') {
// dataSet = raw.data;
// }
// return dataSet;
// },
},
},
pageSize: 10,
serverPaging: true,
serverFiltering: true,
serverSorting: true,
saveState: false
},
// layout definition
layout: {
scroll: true,
footer: false,
},
rows: {
autoHide: false,
},
// column sorting
sortable: true,
pagination: true,
search: {
input: $('#kt_datatable_search_query'),
key: 'name',
onEnter: true
},
// columns definition
columns: [{
field: 'id',
title: '#',
sortable: true,
width: 30,
type: 'number',
textAlign: 'center',
selector: false,
},
{
field: 'Actions',
title: 'Actions',
sortable: false,
overflow: 'visible',
autoHide: false,
template: function(row) {
return '\
<a href="#" data-toggle="modal" data-target="#exampleModalSizeLg" \
onClick="window.Organizatioin.showEditForm(event, '+row.id+')" \
class="btn btn-light btn-hover-primary btn-sm btn-icon mr-2" title="Edit">\
<i class="flaticon2-edit text-muted"></i>\
</a>\
<a href="#" class="btn btn-light btn-hover-primary btn-sm btn-icon mr-2" title="Delete" \
onClick="window.Organizatioin.showDeleteForm(event, '+row.id+')" /> \
<i class="flaticon2-rubbish-bin-delete-button text-muted"></i>\
</a>\
';
},
},
{
field: 'status',
title: 'Status',
template: function(row) {
var status = {
'A': {
'title': 'Active',
'class': ' label-light-success',
},
'I': {
'title': 'In-active',
'class': ' label-light-danger',
}
};
return '<span class="label font-weight-bold label-lg ' + status[row.status].class + ' label-inline">' + status[row.status].title + '</span>';
},
},
{
field: 'org_name',
title: 'Organization Name',
},
{
field: 'org_code',
title: 'Organization Code',
},
{
field: 'email',
title: 'Email',
},
{
field: 'website',
title: 'Website',
},
{
field: 'created_by_user.name',
title: 'Created By',
},
{
field: 'created_at',
title: 'Created At',
},
{
field: 'updated_by_user.name',
title: 'Updated By',
},
{
field: 'updated_at',
title: 'Updated At',
}
],
});
$('#kt_datatable_search_submit').on('click', function(e){
// console.log($('#kt_datatable_search_query').val());
datatable.setDataSourceParam('query.name', $('#kt_datatable_search_query').val());
$('#kt_datatable').KTDatatable("reload");
});
// $(document).on('keypress',function(e) {
// // console.log(e.key, e.code);
// if(e.code === 'Enter') {
// e.preventDefault();
// $('#kt_datatable_search_submit').trigger('click');
// }
// });
};
return {
// public functions
init: function() {
initDatatable();
},
reload: function() {
$('#kt_datatable').KTDatatable("reload");
}
};
}();
jQuery(function() {
KTDatatableBasic.init();
});
export default ListPage;
现在在上面的代码中,我需要在Actions
列的 Edit 按钮点击事件中传递 function 来调用 react function。
PS:这个问题与这篇文章有关: https://gist.github.com/primaryobjects/64734b8fe3f54aa637f444c95f061eed
解决了
在 ListPage.js 中:下面注释了需要进行的Answer
更改。
import React, { useCallback, useEffect, useRef, useState } from "react";
import Config from "../../../config";
import SearchForm from "../../html/common/SearchForm";
const ListPage = (props) => {
const [editId, setEditId] = useState('');
useEffect(()=>{
console.log('ListPage Render', editId);
if(editId){
props.editRecord(editId);
}
}, [editId]);
return(
<div>
<SearchForm placeholder="keyword"
id="kt_datatable_search_query"
buttonId="kt_datatable_search_submit"
/>
<input name="editId" type="text" onInput={(e)=>setEditId(e.target.value)} value={editId} />
<div id="kt_datatable"></div>
</div>
)
}
jQuery(function() {
KTDatatableBasic.init();
// ====== Answer: Need to fire input event manually =====
window.updateEditId = function(id) {
var ev = new Event('input', { bubbles: true});
// ev.simulated = true;
$("input[name='editId']").val(id)[0].dispatchEvent(ev);
}
});
"use strict";
// Class definition
...
...
...
/** as per above **/
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.