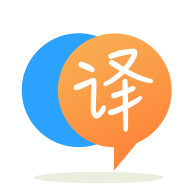
[英]How can I convert the this cascading dropdown class component to functional one using React Hooks?
[英]how to set first value of array as default in cascading dropdown in react hooks
我正在使用材料 UI 列出下拉值我有一个中心、国家和帐户下拉字段我想将数组 object 的第一个值设置为 select 初始值已选择。
数据文件:
export const dataHub = [
{ name: 'Western Europe', hubId: 1 },
{ name: 'IMMEA', hubId: 2 },
]
export const dataCountry = [
{ name: 'France', countryId: 1, hubId: 1 },
{ name: 'Germany', countryId: 2, hubId: 1 },
{ name: 'Italy', countryId: 3, hubId: 1 },
{ name: 'Spain', countryId: 4, hubId: 1 },
{ name: 'Sweden', countryId: 5, hubId: 1 },
{ name: 'Switzerland', countryId: 6, hubId: 2 },
{ name: 'Uk', countryId: 7, hubId: 1 },
]
export const dataAccount = [
{name:'Telco-channel',panterName:'',accountId:1,countryId:1},
{name:'Consumer-Online',panterName:'',accountId:2,countryId:2},
{name:'Education-Retail',panterName:'',accountId:3,countryId:2},
{name:'Non-Trade',panterName:'',accountId:4,countryId:2},
{name:'Telco-channel',panterName:'',accountId:5,countryId:3},
{name:'Commercial-channel',panterName:'',accountId:6,countryId:4},
{name:'Consumer-Retail',panterName:'',accountId:7,countryId:5},
{name:'Commercial-Online',panterName:'',accountId:8,countryId:6},
{name:'Non-Trade',panterName:'',accountId:9,countryId:6},
{name:'Education-Online',panterName:'',accountId:10,countryId:1},
{name:'Consumer-Retail',panterName:'',accountId:11,countryId:2},
{name:'Telco-channel',panterName:'',accountId:12,countryId:2},
{name:'Commercial-channel',panterName:'',accountId:13,countryId:3},
{name:'Consumer-Online',panterName:'',accountId:14,countryId:3},
{name:'Consumer-Online',panterName:'',accountId:15,countryId:4},
{name:'Consumer-Retail',panterName:'',accountId:16,countryId:4},
{name:'Non-Trade',panterName:'',accountId:17,countryId:4},
{name:'Telco-channel',panterName:'',accountId:18,countryId:4},
{name:'Consumer-Online',panterName:'',accountId:19,countryId:5},
{name:'Commercial-Retail',panterName:'',accountId:20,countryId:7},
{name:'Consumer-Online',panterName:'',accountId:21,countryId:7},
{name:'Education-Online',panterName:'',accountId:22,countryId:7},
{name:'Education-Retial',panterName:'',accountId:23,countryId:7},
{name:'Non-Trade',panterName:'',accountId:24,countryId:7},
]
下面是组件渲染下拉字段
import React, { useState, useEffect } from 'react'
import { dataHub, dataCountry, dataAccount } from "../../constants/defaultValues";
import SelectMenu from '../../components/SelectMenu';
const defaultItemHub = { name: 'Select Hub ...' };
const defaultItemCountry = { name: 'Select Country ...' };
const defaultItemAccount = { name: 'Select Account ...' };
const Filters = () => {
const [hub, setHub] = useState('')
const [country, setCountry] = useState('')
const [account, setAccount] = useState('')
const [countries, setCountries] = useState(dataCountry)
const [accounts, setAcconts] = useState(dataAccount)
useEffect(() => {
const defaultHub = sort(dataHub)[0]
const defaultCountry = sort(dataCountry).filter(country => country.hubId === defaultHub.hubId)[0];
let defaultAccount = sort(dataAccount).filter(account => account.countryId === defaultCountry.countryId)[0];
setHub(defaultHub)
setCountry(defaultCountry)
setAccount(defaultAccount)
}, [])
const hubChange = (event) => {
const hub = event.target.value;
const countries = dataCountry.filter(country => country.hubId === hub.hubId);
setHub(hub)
setCountries(countries)
setCountry('')
setAccount('')
}
const countryChange = (event) => {
const country = event.target.value;
const accounts = dataAccount.filter(account => account.countryId === country.countryId);
setCountry(country)
setAcconts(accounts)
setAccount('')
}
const accountChange = (event) => {
setAccount(event.target.value);
}
const hasHub = hub && hub !== defaultItemHub;
const hasCountry = country && country !== defaultItemCountry;
//console.log("defaultHub",defaultHub)
return (
<div className="container">
<div className="d-flex mr-1 justify-content-center align-items-center">
<SelectMenu field={"Hub"} value={hub} options={dataHub} fieldtype={"dropdown"} onChange={hubChange} />
<SelectMenu field={"Country"} value={country} disabled={!hasHub} options={countries} fieldtype={"dropdown"} onChange={countryChange} />
<SelectMenu field={"Account"} value={account} disabled={!hasCountry} options={accounts} fieldtype={"dropdown"} onChange={accountChange} />
</div>
</div>
)
}
export default Filters
selectMenu 组件,我正在为下面的组件传递所需的道具
import React from 'react';
import { makeStyles } from '@material-ui/core/styles';
import InputLabel from '@material-ui/core/InputLabel';
import MenuItem from '@material-ui/core/MenuItem';
import FormControl from '@material-ui/core/FormControl';
import Select from '@material-ui/core/Select';
import TextField from '@material-ui/core/TextField';
const useStyles = makeStyles((theme) => ({
formControl: {
margin: theme.spacing(2),
minWidth: 180,
},
selectEmpty: {
marginTop: theme.spacing(2),
},
}));
export default function SelectMenu({ field, options, value, disabled, fieldtype, onChange }) {
const classes = useStyles();
const handleChange = (event) => {
onChange(event)
};
// When the field is a dropdown
if (fieldtype === "dropdown") {
return (
<div>
<FormControl variant="outlined" className={classes.formControl}>
<InputLabel id="demo-simple-select-outlined-label">{field}</InputLabel>
<Select
labelId="demo-simple-select-outlined-label"
id="demo-simple-select-outlined"
value={value || ''}
onChange={handleChange}
label={field}
disabled={disabled}
>
{
options.map((element, key) => {
return (
<MenuItem key={key} value={element}>{element.name}</MenuItem>
)
})
}
</Select>
</FormControl>
</div>
);
}
else {
// When the field is a Integer and Prepopulated
if (options != null) {
return (
<div>
<FormControl variant="outlined" className={classes.formControl}>
<TextField
id="outlined-read-only-input"
label={field}
value={options[0].value}
defaultValue="Hello World"
InputProps={{
readOnly: true,
}}
variant="outlined"
/>
</FormControl>
</div>
)
}
//When the field is a Integer and a Input from the user
else {
return (
<div>
<FormControl variant="outlined" className={classes.formControl}>
<TextField id="outlined-basic"
onChange={handleChange}
label={field} variant="outlined" />
</FormControl>
</div>
)
}
}
}
谁能帮助我我做错了什么,我无法在下拉列表中设置默认值。
尝试在<Select>
标记中使用defaultValue
而不是value
。
我觉得有几个原因可能会导致这个问题。
If the value is an object it must have reference equality with the option in order to be selected. If the value is not an object, the string representation must match with the string representation of the option in order to be selected.
If the value is an object it must have reference equality with the option in order to be selected. If the value is not an object, the string representation must match with the string representation of the option in order to be selected.
因此,您应该定义相等,以便Select
的value
属性将与MenuItem
的value
属性匹配,然后将其选为默认值。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.