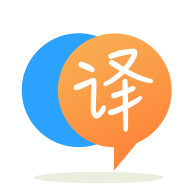
[英]error: comparison between pointer and integer ('int' and 'string' (aka 'char *'))
[英]Comparison between pointer (char*) and integer error
所以基本上用户在一个变量中输入他们访问过多少个国家,然后我把它作为数组的大小。
之后,我使用for
循环列出所有访问过的国家/地区。 但我想让我的代码更聪明一点, and
在最后一个国家的句子末尾加上和。
例如3
个国家:
You visited Japan, Korea and Canada.
^ ^^^
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int number_of_p = get_int("How many countries did you visit?\n");
string countries[number_of_p];
for (int x = 0; x < number_of_p; x++)
{
countries[x] = get_string("What countries did you visit?\n");
}
printf("You visited %i countries, including: ", number_of_p);
for (int x = 0; x < number_of_p; x++)
{
printf("%s, ", countries[x]);
/* looks for last element in arrays, inserts 'and'
to make it look grammatically correct. */
if (countries[x] == number_of_p - 1 ) // ERROR HERE
{
printf(" and ");
}
}
printf(".\n");
}
我正在比较指针( char*
)和 integer 错误。
char*
是什么意思?
如何访问数组中的最后一个元素?
country countries[x]
是一个string
(在CS50
中是char*
的 typedef), number_of_p
是一个int
,你不能比较它们,它们是不同的类型,你可能想比较索引x
,一个可能的(和快速)修复您的代码,包括标点符号可能如下所示:
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int number_of_p = get_int("How many countries did you visit?\n");
string countries[number_of_p];
for (int x = 0; x < number_of_p; x++)
{
countries[x] = get_string("What countries did you visit?\n");
}
printf("You visited %i countries, including: ", number_of_p);
for (int x = 0; x < number_of_p; x++)
{
printf("%s", countries[x]);
if(x < number_of_p - 2){
printf(", ");
}
if (x == number_of_p - 2)
{
printf(" and ");
}
}
printf(".\n");
}
输入:
3
Japan
Korea
Canada
Output:
You visited 3 countries, including: Japan, Korea and Canada.
if 语句中的条件
if (countries[x] == number_of_p - 1 )
没有意义。 左操作数countries[x]
的类型为char *
而右操作数的类型为int
。
也就是说,类型说明符string
是char *
类型的别名,而这个数组声明
string countries[number_of_p];
是相同的
char * countries[number_of_p];
所以你有一个指向字符串的指针数组。
C 中char *
类型的别名string
定义如下
typedef char * string;
循环看起来像
for (int x = 0; x < number_of_p; x++)
{
if ( x != 0 )
{
putchar( ',' );
putchar( ' ' );
if ( x == number_of_p - 1 )
{
printf( "%s ", "and " );
}
}
printf("%s", countries[x]);
}
这是一个演示程序
#include <stdio.h>
int main(void)
{
enum { number_of_p = 3 };
char *countries[number_of_p] =
{
"Japan", "Korea", "Canada"
};
printf( "You visited %i countries, including: ", number_of_p );
for (int x = 0; x < number_of_p; x++)
{
if ( x != 0 )
{
putchar( ',' );
putchar( ' ' );
if ( x == number_of_p - 1 )
{
printf( "%s ", "and" );
}
}
printf("%s", countries[x]);
}
return 0;
}
它的 output 是
You visited 3 countries, including: Japan, Korea, and Canada
您可以使用一个 printf 执行此类操作,使用预定义的字符串作为分隔符。 使用单个 printf 而不是特殊套管,列表的尾部通常更易于维护。 许多人对三元运算符感到困惑。 这是两种不同方式的方法,首先使用开关,然后使用三元运算符:
#include<stdio.h>
int main(void)
{
char *countries[] = { "foo", "bar", "baz" };
int number_of_p = sizeof countries / sizeof *countries;
/* First loop, using a switch */
for( int x = 0; x < number_of_p; x++ ) {
char *div = ", ";
switch( x ){
case sizeof countries / sizeof *countries - 2: div = ", and "; break;
case sizeof countries / sizeof *countries - 1: div = ".\n"; break;
}
printf("%s%s", countries[x], div);
}
/* Same loop as above, with if/else instead of the switch */
for( int x = 0; x < number_of_p; x++ ) {
char *div = ", ";
if( x == number_of_p - 2 ) {
div = ", and ";
} else if( x == number_of_p - 1 ) {
div = ".\n";
}
printf("%s%s", countries[x], div);
}
/* Same loop as above, written less verbosely */
for( int x = 0; x < number_of_p; x++ ) {
printf("%s%s", countries[x], x == number_of_p - 2 ? ", and " :
x == number_of_p - 1 ? ".\n" : ", ");
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.