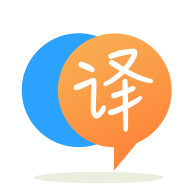
[英]Springboot + thymeleaf displaying database contents in html table
[英]SpringBoot + Thymeleaf + SQL, display HTML table from database
我想创建网站。 我已经配置了登录“/login”和注册“/registration”页面。 该站点的主要任务是显示每个学生的时间表。 现在我需要根据学生在注册期间选择的字段 GROUP 和 COURSE 使用 HTML 表在页面“/schedule”上显示科目列表。 我有 14 个带有科目列表的不同表格(1 个表格 - 一组学生)。
“/schedule”页面的 Controller:
@Controller
public class ShedulePageController {
@GetMapping("/schedule")
public String schedulePage() {
return "SchedulePage";
}
}
包含主题列表的 14 个表中的 1 个的实体:
@Entity
@Table(name = "f_fit_c2g1")
public class f_fit_c2g1 {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id_f_fit_c2g1;
@Column(length = 60)
private String monday;
@Column(length = 60)
private String tuesday;
@Column(length = 60)
private String wednesday;
@Column(length = 60)
private String thursday;
@Column(length = 60)
private String friday;
@Column(length = 60)
private String monday2;
@Column(length = 60)
private String tuesday2;
@Column(length = 60)
private String wednesday2;
@Column(length = 60)
private String thursday2;
@Column(length = 60)
private String friday2;
public String getMonday2() {
return monday2;
}
public void setMonday2(String monday2) {
this.monday2 = monday2;
}
public String getTuesday2() {
return tuesday2;
}
public void setTuesday2(String tuesday2) {
this.tuesday2 = tuesday2;
}
public String getWednesday2() {
return wednesday2;
}
public void setWednesday2(String wednesday2) {
this.wednesday2 = wednesday2;
}
public String getThursday2() {
return thursday2;
}
public void setThursday2(String thursday2) {
this.thursday2 = thursday2;
}
public String getFriday2() {
return friday2;
}
public void setFriday2(String friday2) {
this.friday2 = friday2;
}
public Long getId_c2g9() {
return id_f_fit_c2g1;
}
public void setId_c2g9(Long id_f_fit_c2g1) {
this.id_f_fit_c2g1 = id_f_fit_c2g1;
}
public String getMonday() {
return monday;
}
public void setMonday(String monday) {
this.monday = monday;
}
public String getTuesday() {
return tuesday;
}
public void setTuesday(String tuesday) {
this.tuesday = tuesday;
}
public String getWednesday() {
return wednesday;
}
public void setWednesday(String wednesday) {
this.wednesday = wednesday;
}
public String getThursday() {
return thursday;
}
public void setThursday(String thursday) {
this.thursday = thursday;
}
public String getFriday() {
return friday;
}
public void setFriday(String friday) {
this.friday = friday;
}
}
每个带有主题的表都有这样的名称 - “f_fit_c#g#”,其中 # - 数字。
学堂:
import org.springframework.data.jpa.repository.JpaRepository;
public interface f_fit_c2g1Repository extends JpaRepository<f_fit_c2g1, Long> {
}
我的“/schedule”页面的 HTML 文件(带有随机 static 数据):
<html xmlns:th="http://www.thymeleaf.org" lang="en">
<head>
<title>Table 07</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}" />
</head>
<body>
<header>
<div class="small" id="header-scroll">
<h1><a href="#" style="padding-left: 20px">KNUTE</a></h1>
<nav>
<ul>
<li><a href="#">Расписание</a></li>
<li><a href="#">Домашнее задание</a></li>
<li><a href="#">Профиль</a></li>
<li><a href="#">Полезные ссылки</a></li>
</ul>
</nav>
</div>
</header>
<section class="ftco-section">
<div class="container">
<div class="row justify-content-center">
<div class="col-md-6 text-center mb-5">
<h2 class="heading-section">Расписание на первую неделю</h2>
</div>
</div>
<div class="row">
<div class="col-md-12">
<table class="table table-bordered table-dark table-hover">
<thead>
<tr style="text-align: center; width: 20px">
<th>#</th>
<th>Время</th>
<th>Понедельник</th>
<th>Вторник</th>
<th>Среда</th>
<th>Четверг</th>
<th>Пятница</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>8:20 - 9:40</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">2</th>
<td>10:05 - 11:25</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">3</th>
<td>12:05 - 13:25</td>
<td>the Bird</td>
<td>larrybird@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">4</th>
<td>13:50 - 15:10</td>
<td>Doe</td>
<td>johndoe@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">5</th>
<td>15:25 - 16:45</td>
<td>Bird</td>
<td>garybird@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">6</th>
<td>17:00 - 18:20</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">7</th>
<td>18:45 - 20:05</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="row justify-content-center">
<div class="col-md-6 text-center mb-5">
<h2 class="heading-section" style="padding-top: 65px">Расписание на вторую неделю</h2>
</div>
</div>
<div class="row">
<div class="col-md-12">
<table class="table table-bordered table-dark table-hover">
<thead>
<tr style="text-align: center; width: 20px">
<th>#</th>
<th>Время</th>
<th>Понедельник</th>
<th>Вторник</th>
<th>Среда</th>
<th>Четверг</th>
<th>Пятница</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>8:20 - 9:40</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">2</th>
<td>10:05 - 11:25</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">3</th>
<td>12:05 - 13:25</td>
<td>the Bird</td>
<td>larrybird@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">4</th>
<td>13:50 - 15:10</td>
<td>Doe</td>
<td>johndoe@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">5</th>
<td>15:25 - 16:45</td>
<td>Bird</td>
<td>garybird@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">6</th>
<td>17:00 - 18:20</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
<tr>
<th scope="row">7</th>
<td>18:45 - 20:05</td>
<td>Otto</td>
<td>markotto@email.com</td>
<td>Thornton</td>
<td>jacobthornton@email.com</td>
<td>Thornton</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</section>
</body>
</html>
我找到了很多关于类似主题的信息,但我找不到有关使用多个表的信息。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.