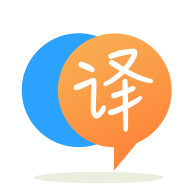
[英]LINQ query with EF Core to find records with matching items from many-to-many relationship
[英]EF Core exclude certain property in query, many-to-many relationship
如何仅在具有多对多关系的数据库中查询某些属性?
所以我有一本书可以有多个作者
public class Book
{
public Book()
{
Authors = new List<BookAuthor>();
}
public string Id { get; set; }
public string Description { get; set; }
public List<BookAuthor> Authors { get; set; }
}
书籍和作者的连接表
public class BookAuthor
{
public string BookId{ get; set; }
public string AuthorId{ get; set; }
public Book book{ get; set; }
public Author author{ get; set; }
}
最后,作者可以写多本书
public class Author
{
public string Id { get; set; }
public string Name { get; set; }
public List<BookAuthor> books { get; set; }
}
现在,当我想查询一本书并返回书的 ID 和描述而不是该书的作者列表时
return await _context.Book.Select(x => new Book
{
Id = x.Id,
Description = x.Description,
}).ToListAsync();
当我这样做时,我得到了
{
"id": "BOOK123",
"description": "Stack Overflow 101",
"Authors": []
}
我想在查询的返回中从 Book class 中排除属性 Author。 我如何实现这一目标?
您需要为 Book 创建一个 DTO object:
public class BookDto
{
public string Id { get; set; }
public string Description { get; set; }
}
然后调用:
return await _context.Book.Select(x => new BookDto
{
Id = x.Id,
Description = x.Description,
}).ToListAsync();
更高级的方法是使用 Automapper ( https://automapper.org/ ) 并在您的 Book 和 BookDto 之间创建一个映射配置文件:
public class BookProfile : Profile
{
public BookProfile()
{
CreateMap<Book, BookDto>();
}
}
然后你可以像这样检索你的 BookDtos:
return await _context.Book
.ProjectTo<BookDto>(_mapper.ConfigurationProvider)
.ToListAsync();
其中 _mapper 是 IMapper 类型。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.