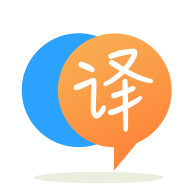
[英]I want to print the fibonacci series using two threads. Like 1st number should be printed by 1st thread and then 2nd number by 2nd thread and so on
[英]halt the 1st thread after each iteration so the 2nd thread can produce the factorial of that generated Random number
在这里,我想生成随机生成的数字的阶乘。
import java.lang.Math;
public class Assignment1 {
int random = 1;
int fact = 1;
public void RandomGenrator(){
synchronized(this){
for(int k=0; k<5;k++){
random = (int)(Math.random()*10);
System.out.println("Random Number --> "+random);
notifyAll();
}
}
}
public void Factorial(){
synchronized(this){
if(random == 0){
System.out.println("Factorial of "+random+" is "+1);
}else{
for(int j=1; j<=random; j++){
fact *=j;
}
System.out.println("Factorial of "+random+" is "+fact);
}
notify();
}
}
public static void main(String[] args) {
Assignment1 as = new Assignment1();
Thread t1 = new Thread(new Runnable(){
public void run(){
as.RandomGenrator();
}
});
Thread t2 = new Thread(new Runnable(){
public void run(){
as.Factorial();
}
});
t1.start();
t2.start();
}
}
This produces output like this
Random Number --> 2
Random Number --> 6
Random Number --> 4
Random Number --> 8
Random Number --> 4
Factorial of 4 is 24
but I want
Random Number --> 2
Factorial of 2 is 2
Random Number --> 6
Factorial of 6 is 720
Random Number --> 4
Factorial of 4 is 24
Random Number --> 8
Factorial of 8 is 5760
Random Number --> 4
Factorial of 4 is 24
我知道这可以通过简单的 for 循环来实现,if else 结构但我想使用线程来实现这一点,那么如何在每次迭代后停止第一个线程,以便第二个线程可以产生生成的随机数的阶乘
您的代码没有使用任何wait
,因此没有线程会等待另一个线程。 您可以使用boolean
变量来指示谁在等待谁。 在这里,如果flag
为真,则阶乘正在等待随机生成器生成一个数字。 如果flag
为 false,则随机生成器正在等待阶乘计算。
boolean flag = true; // initially, we wait for the random generator to generate first
public void RandomGenrator(){
for (int i = 0 ; i < 5 ; i++) {
synchronized (this) {
while (!flag) {
try {
wait();
} catch (InterruptedException e) {
System.out.println("interrupted");
Thread.currentThread().interrupt();
}
}
random = (int) (Math.random() * 10);
System.out.println("Random Number --> " + random);
flag = false; // now waiting for the factorial to compute!
notify();
}
}
}
public void Factorial(){
for (int i = 0 ; i < 5 ; i++) {
synchronized (this) {
while (flag) {
try {
wait();
} catch (InterruptedException e) {
System.out.println("interrupted");
Thread.currentThread().interrupt();
}
}
if (random == 0) {
System.out.println("Factorial of " + random + " is " + 1);
} else {
fact = 1;
for (int j = 1; j <= random; j++) {
fact *= j;
}
System.out.println("Factorial of " + random + " is " + fact);
}
flag = true; // now waiting for the random generator to generate a new number!
notify();
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.