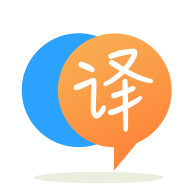
[英]How to write a random group generator in java using else-if statements?
[英]Assisstance with for loops / else-if statements in Java
在这段代码中,我试图模拟从网格左上角开始的森林火灾。 根据其位置,火势蔓延到下一棵树的百分比不同。 该代码旨在移动到下一棵树,如果其左侧的树着火,它有 85% 的机会传播,并且有 35% 的机会向所有其他方向传播。 出于某种原因,我的第三个和第四个 else-if 语句不适用于向右或上方传播火力,它基本上忽略了它,我不知道为什么。
public class ForestFire {
int[][] trees = new int [30][40];
int fire = 1;
int no_fire = 0;
double fire_count = 0;
double no_fire_count = 0;
double total_fire_count = 0;
double total_no_fire_count = 0;
static int total = 10;
double average = 0;
int trial = 0;
public void FireSpread() {
for(int x = 0; x < total; x++) {
for(int i = 0; i < trees.length; i++) {
for(int j = 0; j < trees[i].length; j++) {
double random = Math.random();
if(i == 0 && j == 0) {
trees[0][0] = fire;
System.out.print(trees[0][0] + " ");
fire_count++;
total_fire_count++;
}
else if((j-1) >= 0 && trees[i][j - 1] == fire && random < 0.85) {
trees[i][j] = fire;
System.out.print(trees[i][j] + " ");
fire_count++;
total_fire_count++;
}
else if((i-1) >= 0 && trees[i - 1][j] == fire && random < 0.35) {
trees[i][j] = fire;
System.out.print(trees[i][j] + " ");
fire_count++;
total_fire_count++;
}
else if((j+1) <= 39 && trees[i][j + 1] == fire && random < 0.35) {
trees[i][j] = fire;
System.out.print(trees[i][j] + " ");
fire_count++;
total_fire_count++;
}
else if((i+1) <= 29 && trees[i + 1][j] == fire && random < 0.35) {
trees[i][j] = fire;
System.out.print(trees[i][j] + " ");
fire_count++;
total_fire_count++;
}
else {
trees[i][j] = no_fire;
System.out.print(trees[i][j] + " ");
no_fire_count++;
total_no_fire_count++;
}
}
System.out.println();
}
System.out.println();
System.out.println("Fire Count: " + fire_count);
System.out.println("No Fire Count: " + no_fire_count);
System.out.println("Average: " + fire_count / 1200);
if(fire_count > 480) {
trial ++;
}
average += fire_count / 1200;
System.out.println();
for(int i = 0; i < trees.length; i++) {
for(int j = 0; j < trees[i].length; j++) {
trees[i][j] = no_fire;
}
}
fire_count = 0;
no_fire_count = 0;
}
System.out.println("Above 40 Percent: " + trial);
System.out.println("Total Fire Count: " + total_fire_count);
System.out.println("Total No Fire Count: " + total_no_fire_count);
System.out.println("Overall Average: " + average / total);
System.out.println();
}
public double getFireCount() {
return fire_count;
}
public double getNoFireCount() {
return no_fire_count;
}
public double getTotalFireCount() {
return total_fire_count;
}
public double getTotalNoFireCount() {
return total_no_fire_count;
}
public static void main(String[] args) {
ForestFire one = new ForestFire();
one.FireSpread();
System.out.println("Average Fire Count: " + one.getTotalFireCount() / total);
System.out.println("Average No Fire Count: " + one.getTotalNoFireCount() / total);
}
}
一般来说,我建议您学习编写单元测试并使用交互式调试器。 这两项技能将解决您在编码中面临的绝大多数问题。
您的代码的基本问题是,在迭代的每一步中,您都将模拟结果写入您正在从中读取当前 state 的同一数组中。 这使得传播取决于您碰巧编写for
循环的方式(这是错误的)。 一般来说,您需要为每个步骤创建一个新的 state,然后在步骤结束时复制到当前的 state。 一个简单的检查(您应该在单元测试中进行检查)是每个步骤最多应该有 4 棵树着火。
作为附加提示,如果您将 state 建模为一组着火的位置而不是包含值的数组,您将使您的代码更易于阅读和理解。 这将使很多代码更简单。 理想情况下,您从当前 state 计算下一个 state 的代码如下所示:
Set<Position> nextState = new HashSet<>(currentState);
for (Position fire: currentState) {
for (Direction direction: Direction.values()) {
Position neighbour = direction.move(fire);
if (neighbour.inArea() && direction.shouldSpread())
nextState.add(neighbour);
}
}
currentState = nextState;
这封装了关于哪些位置在Position
和Direction
类中的区域和传播概率的逻辑,而不是全部在单个 function 中。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.