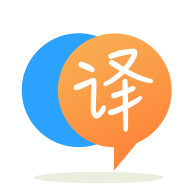
[英]How to reverse a string in JavaScript using a "for...in" loop?
[英]how to break from recursion when using for...in loop
const find = (json) => {
let result;
for (const key in json) {
if(typof json[key] === "number") {
result = json[key];
break;
} else {
find(json[key])
}
return result;
}
上面的代码有助于找到类型为 number 的属性的值,我是否有机会打破 for..in 而不继续递归直到它结束并访问我在上面的代码中尝试做的值。 请让我知道在嵌套 JSON 中访问特定值的理想方法,并在实现后中断循环。
在做递归函数时,有几个关键的事情:
看评论:
const find = (obj) => {
for (const key in obj) {
const value = obj[key];
if (typeof value === "number") {
// Found one -- return it (this is #2)
return value;
} else if (value && typeof value === "object") {
// Found an object -- recurse (this is #3)
const result = find(value);
// Did recursion find a number?
if (typeof result === "number") {
// Yes, return it (this is #3.1)
return result;
}
}
}
// Nothing found, return `undefined` (explicitly or implicitly)
// This is #4
// This is explicitly: `return undefined;`
};
请注意,我将json
更改为obj
。 它显然不是 JSON (字符串)。
现场示例:
const find = (obj) => { console.log(`Looking in ${JSON.stringify(obj)}`); for (const key in obj) { const value = obj[key]; if (typeof value === "number") { // Found one -- return it (this is #2) console.log(`Found ${value}, returning it`); return value; } else if (value && typeof value === "object") { // Found an object -- recurse (this is #3) console.log(`Found object, recursing`); const result = find(value); // Did recursion find a number? if (typeof result === "number") { // Yes, return it (this is #3.1) console.log(`Recursive call found it`); return result; } } } // Nothing found, return `undefined` (explicitly or implicitly) // This is #4 // This is explicitly: `return undefined;` console.log(`Didn't find anything`); }; // Found at the top level: console.log(find({answer: 42})); // Found nested console.log(find({ s: "string", o: { n: null, o: { s: "string", answer: 42, }, }, n: null, })); // Not found console.log(find({}));
.as-console-wrapper { max-height: 100%;important; }
组织可破坏的深度递归的一种简单方法是使用递归生成器。 当我们在for-of
循环中使用它并break
循环时,所有挂起的递归将自动停止:
function* values(obj) { if (obj instanceof Object) for (let val of Object.values(obj)) yield* values(val); else yield obj; } // obj = {deep: {object: 'hey'}, here: {be: {dragons: ['a', 'b', {see: 42}, 'skip']}}} for (let val of values(obj)) if (typeof val === 'number') { console.log('Number', val, 'found') break }
循环也可以抽象出来:
function find(iter, predicate) {
for (let x of iter)
if (predicate(x))
return x
}
接着
num = find(values(obj), x => typeof x === 'number')
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.